Description
Class for wrapping the HACD convex decomposition code revisited by John Ratcliff.
#include <ChConvexDecomposition.h>
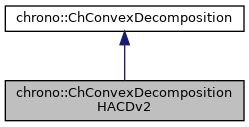
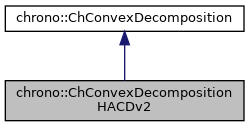
Public Member Functions | |
ChConvexDecompositionHACDv2 () | |
Basic constructor. | |
virtual | ~ChConvexDecompositionHACDv2 () |
Destructor. | |
virtual void | Reset (void) |
Reset the input mesh data. | |
virtual bool | AddTriangle (const ChVector3d &v1, const ChVector3d &v2, const ChVector3d &v3) |
Add a triangle, by passing three points for vertexes. More... | |
virtual bool | AddTriangleMesh (const ChTriangleMesh &tm) |
Add a triangle mesh soup, by passing an entire ChTriangleMesh object. More... | |
void | SetParameters (unsigned int mMaxHullCount=256, unsigned int mMaxMergeHullCount=256, unsigned int mMaxHullVertices=64, float mConcavity=0.2f, float mSmallClusterThreshold=0.0f, float mFuseTolerance=1e-9) |
Set the parameters for this convex decomposition algorithm. More... | |
virtual int | ComputeConvexDecomposition () |
Perform the convex decomposition. More... | |
virtual unsigned int | GetHullCount () |
Get the number of computed hulls after the convex decomposition. | |
virtual bool | GetConvexHullResult (unsigned int hullIndex, ChTriangleMesh &convextrimesh) |
Get the n-th computed convex hull, by filling a ChTriangleMesh object that is passed as a parameter. | |
virtual bool | GetConvexHullResult (unsigned int hullIndex, std::vector< ChVector3d > &convexhull) |
Get the n-th computed convex hull, by filling a vector of points of the vertexes of the n-th hull that is passed as a parameter. | |
virtual void | WriteConvexHullsAsWavefrontObj (std::ostream &mstream) |
Save the computed convex hulls as a Wavefront file using the '.obj' fileformat, with each hull as a separate group. More... | |
![]() | |
ChConvexDecomposition () | |
Basic constructor. | |
virtual | ~ChConvexDecomposition () |
Destructor. | |
virtual bool | AddTriangle (const ChTriangle &t1) |
Add a triangle, by passing a ChTriangle object (that will be copied, not referenced). More... | |
virtual bool | WriteConvexHullsAsChullsFile (std::ostream &mstream) |
Write the convex decomposition to a ".chulls" file, where each hull is a sequence of x y z coords. More... | |
Member Function Documentation
◆ AddTriangle()
|
virtual |
Add a triangle, by passing three points for vertexes.
Note: the vertexes must be properly ordered (oriented triangle, normal pointing outside)
Implements chrono::ChConvexDecomposition.
◆ AddTriangleMesh()
|
virtual |
Add a triangle mesh soup, by passing an entire ChTriangleMesh object.
Note 1: the triangle mesh does not need connectivity information (a basic 'triangle soup' is enough) Note 2: all vertexes must be properly ordered (oriented triangles, normals pointing outside). Note 3: the triangles must define closed volumes (holes, gaps in edges, etc. may trouble the decomposition)
Reimplemented from chrono::ChConvexDecomposition.
◆ ComputeConvexDecomposition()
|
virtual |
Perform the convex decomposition.
This operation is time consuming, and it may take a while to complete. Quality of the results can depend a lot on the parameters. Also, meshes with triangles that are not well oriented (normals always pointing outside) or with gaps/holes, may give wrong results.
Implements chrono::ChConvexDecomposition.
◆ SetParameters()
void chrono::ChConvexDecompositionHACDv2::SetParameters | ( | unsigned int | mMaxHullCount = 256 , |
unsigned int | mMaxMergeHullCount = 256 , |
||
unsigned int | mMaxHullVertices = 64 , |
||
float | mConcavity = 0.2f , |
||
float | mSmallClusterThreshold = 0.0f , |
||
float | mFuseTolerance = 1e-9 |
||
) |
Set the parameters for this convex decomposition algorithm.
Use this function before calling ComputeConvexDecomposition(). Repeated vertices will be fused with mFuseTolerance tolerance.
◆ WriteConvexHullsAsWavefrontObj()
|
virtual |
Save the computed convex hulls as a Wavefront file using the '.obj' fileformat, with each hull as a separate group.
May throw exceptions if file locked etc.
Implements chrono::ChConvexDecomposition.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/collision/ChConvexDecomposition.h
- /builds/uwsbel/chrono/src/chrono/collision/ChConvexDecomposition.cpp