Description
Class for referencing a set of 'glyphs', that are simple symbols such as arrows or points to be drawn for showing vector directions etc.
Remember that depending on the type of visualization system (POVray, Irrlicht,etc.) this asset might not be supported.
#include <ChGlyphs.h>
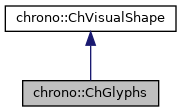
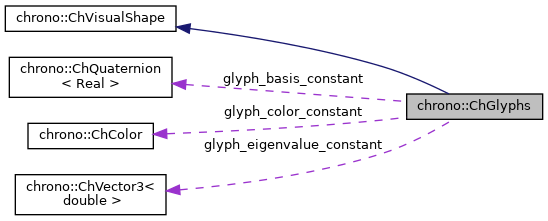
Public Types | |
enum | eCh_GlyphType { GLYPH_POINT = 0, GLYPH_VECTOR, GLYPH_COORDSYS, GLYPH_TENSOR } |
enum | eCh_GlyphLength { CONSTANT = 0, PROPERTY } |
Modes vector length, if GLYPH_VECTOR. | |
enum | eCh_GlyphWidth { CONSTANT = 0, PROPERTY } |
Modes for glyph width or size. | |
enum | eCh_GlyphBasis { CONSTANT = 0, PROPERTY } |
Modes for type of glyph rotation (for GLYPH_COORDSYS mode) | |
enum | eCh_GlyphEigenvalues { CONSTANT = 0, PROPERTY } |
Modes for type of glyph eigenvalues (for GLYPH_TENSOR mode) | |
enum | eCh_GlyphColor { CONSTANT = 0, PROPERTY } |
Modes for colorizing glyph. | |
Public Member Functions | |
eCh_GlyphType | GetDrawMode () const |
Get the way that glyphs must be rendered. | |
void | SetDrawMode (eCh_GlyphType mmode) |
Set the way that glyphs must be rendered. More... | |
void | Reserve (unsigned int n_glyphs) |
Resize the vectors of data so that memory management is faster than calling SetGlyphPoint() etc. More... | |
size_t | GetNumberOfGlyphs () const |
Get the number of glyphs. | |
double | GetGlyphsSize () const |
Get the 'size' (thickness of symbol, depending on the rendering system) of the glyph symbols. | |
void | SetGlyphsSize (double msize) |
Set the 'size' (thickness of symbol, depending on the rendering system) of the glyph symbols. | |
void | SetZbufferHide (bool mhide) |
bool | GetZbufferHide () const |
void | SetGlyphPoint (unsigned int id, ChVector3d mpoint, ChColor mcolor=ChColor(1, 0, 0)) |
Fast method to set a glyph for GLYPH_POINT draw mode. More... | |
void | SetGlyphVector (unsigned int id, ChVector3d mpoint, ChVector3d mvector, ChColor mcolor=ChColor(1, 0, 0)) |
Fast method to set a glyph for GLYPH_VECTOR draw mode. More... | |
void | SetGlyphVectorLocal (unsigned int id, ChVector3d mpoint, ChVector3d mvector, ChQuaternion<> mrot, ChColor mcolor=ChColor(1, 0, 0)) |
Fast method to set a glyph for GLYPH_VECTOR draw mode, with each vectors set in its own local (rotated) basis If the id is more than the reserved amount of glyphs (see Reserve() ) the vectors are inflated. | |
void | SetGlyphCoordsys (unsigned int id, ChCoordsys<> mcoord) |
Fast method to set a glyph for GLYPH_COORDSYS draw mode. More... | |
void | SetGlyphTensor (unsigned int id, ChVector3d mpoint, ChQuaternion<> mbasis, ChVector3d eigenvalues) |
Fast method to set a glyph for GLYPH_TENSOR draw mode. More... | |
std::vector< ChProperty * > | getProperties () |
Gets the current array of per-point properties, if any. | |
void | AddProperty (ChProperty &mprop) |
Add a property as an array of data per-point. More... | |
virtual void | ArchiveOut (ChArchiveOut &archive_out) override |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIn (ChArchiveIn &archive_in) override |
Method to allow de-serialization of transient data from archives. | |
![]() | |
void | SetVisible (bool mv) |
Set this visualization asset as visible. | |
bool | IsVisible () const |
Return true if the asset is set as visible. | |
void | SetColor (const ChColor &col) |
Set the diffuse color for this shape. More... | |
ChColor | GetColor () const |
Return the diffuse color of the first material in the list of materials for this shape. More... | |
void | SetOpacity (float val) |
Set opacity for this shape (0: fully transparent; 1: fully opaque). More... | |
float | GetOpacity () const |
Get opacity of the first material in the list of materials for this shape. More... | |
void | SetTexture (const std::string &filename, float scale_x=1, float scale_y=1) |
Set the diffuse texture map for this shape. More... | |
std::string | GetTexture () const |
Return the diffuse texture map of the first material in the list of materials for this shape. More... | |
void | SetMutable (bool val) |
Set this visualization shape as modifiable (default: false for primitive shapes, true otherwise). More... | |
bool | IsMutable () const |
Return true if the visualization shape is marked as modifiable. | |
int | AddMaterial (std::shared_ptr< ChVisualMaterial > material) |
Add a visualization material and return its index in the list of materials. | |
void | SetMaterial (int i, std::shared_ptr< ChVisualMaterial > material) |
Replace the material with specified index. More... | |
std::vector< std::shared_ptr< ChVisualMaterial > > & | GetMaterials () |
Get the list of visualization materials. | |
std::shared_ptr< ChVisualMaterial > | GetMaterial (int i) |
Get the specified material in the list. | |
unsigned int | GetNumMaterials () const |
Get the number of visualization materials. | |
virtual ChAABB | GetBoundingBox () const |
Get the shape bounding box. More... | |
Public Attributes | |
std::vector< ChVector3d > | points |
eCh_GlyphLength | glyph_length_type |
std::string | glyph_length_prop |
double | glyph_scalelenght |
eCh_GlyphWidth | glyph_width_type |
std::string | glyph_width_prop |
double | glyph_scalewidth |
eCh_GlyphBasis | glyph_basis_type |
std::string | glyph_basis_prop |
ChQuaternion | glyph_basis_constant |
eCh_GlyphEigenvalues | glyph_eigenvalues_type |
std::string | glyph_eigenvalues_prop |
ChVector3d | glyph_eigenvalue_constant |
eCh_GlyphColor | glyph_color_type |
std::string | glyph_color_prop |
ChColor | glyph_color_constant |
double | glyph_colormap_startscale |
double | glyph_colormap_endscale |
bool | vector_tip |
std::vector< ChProperty * > | m_properties |
std::vector< ChColor > * | colors |
std::vector< ChVector3d > * | vectors |
std::vector< ChQuaternion< double > > * | rotations |
std::vector< ChVector3d > * | eigenvalues |
Additional Inherited Members | |
![]() | |
virtual void | Update (ChObj *updater, const ChFrame<> &frame) |
Update this visual shape with information for the owning object. More... | |
![]() | |
bool | visible |
shape visibility flag | |
bool | is_mutable |
flag indicating whether the shape is rigid or deformable | |
std::vector< std::shared_ptr< ChVisualMaterial > > | material_list |
list of visualization materials | |
Member Function Documentation
◆ AddProperty()
|
inline |
Add a property as an array of data per-point.
Deletion will be automatic at the end of mesh life. Warning: mprop.data.size() must be equal to points.size(). Cost: allocation and a data copy.
◆ Reserve()
void chrono::ChGlyphs::Reserve | ( | unsigned int | n_glyphs | ) |
Resize the vectors of data so that memory management is faster than calling SetGlyphPoint() etc.
by starting with zero size vectors that will be inflated when needed..
◆ SetDrawMode()
void chrono::ChGlyphs::SetDrawMode | ( | eCh_GlyphType | mmode | ) |
Set the way that glyphs must be rendered.
Also automatically add needed properties, if not yet done: "V", an array of ChVector3d, for the GLYPH_VECTOR mode "rot", an array of ChQuaternion<>, for the GLYPH_COORDSYS mode "color", an array of ChColor, for all modes
◆ SetGlyphCoordsys()
void chrono::ChGlyphs::SetGlyphCoordsys | ( | unsigned int | id, |
ChCoordsys<> | mcoord | ||
) |
Fast method to set a glyph for GLYPH_COORDSYS draw mode.
If the id is more than the reserved amount of glyphs (see Reserve() ) the csys are inflated.
◆ SetGlyphPoint()
void chrono::ChGlyphs::SetGlyphPoint | ( | unsigned int | id, |
ChVector3d | mpoint, | ||
ChColor | mcolor = ChColor(1, 0, 0) |
||
) |
Fast method to set a glyph for GLYPH_POINT draw mode.
If the id is more than the reserved amount of glyphs (see Reserve() ) the vectors are inflated.
◆ SetGlyphTensor()
void chrono::ChGlyphs::SetGlyphTensor | ( | unsigned int | id, |
ChVector3d | mpoint, | ||
ChQuaternion<> | mbasis, | ||
ChVector3d | eigenvalues | ||
) |
Fast method to set a glyph for GLYPH_TENSOR draw mode.
If the id is more than the reserved amount of glyphs (see Reserve() ) the tensors are inflated.
◆ SetGlyphVector()
void chrono::ChGlyphs::SetGlyphVector | ( | unsigned int | id, |
ChVector3d | mpoint, | ||
ChVector3d | mvector, | ||
ChColor | mcolor = ChColor(1, 0, 0) |
||
) |
Fast method to set a glyph for GLYPH_VECTOR draw mode.
If the id is more than the reserved amount of glyphs (see Reserve() ) the vectors are inflated.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/assets/ChGlyphs.h
- /builds/uwsbel/chrono/src/chrono/assets/ChGlyphs.cpp