Description
Class for a single 'point' node, that has 3 DOF degrees of freedom and a mass.
#include <ChNodeXYZ.h>
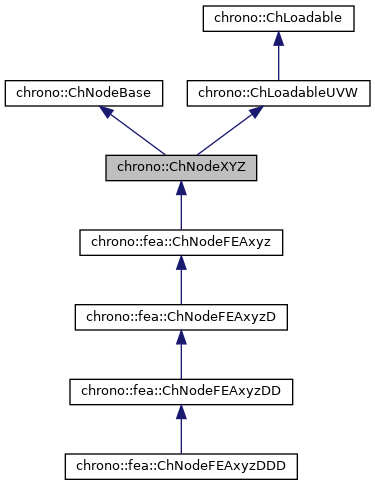
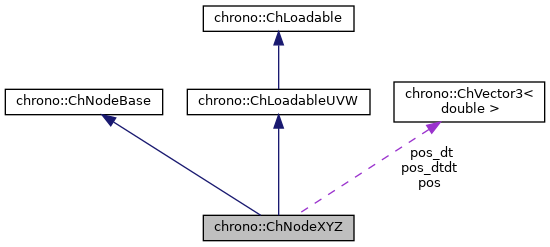
Public Member Functions | |
ChNodeXYZ (const ChVector3d &initial_pos) | |
ChNodeXYZ (const ChNodeXYZ &other) | |
ChNodeXYZ & | operator= (const ChNodeXYZ &other) |
virtual ChVariablesNode & | Variables ()=0 |
const ChVector3d & | GetPos () const |
void | SetPos (const ChVector3d &mpos) |
const ChVector3d & | GetPosDt () const |
void | SetPosDt (const ChVector3d &mposdt) |
const ChVector3d & | GetPosDt2 () const |
void | SetPosDt2 (const ChVector3d &mposdtdt) |
virtual double | GetMass () const =0 |
virtual void | SetMass (double mm)=0 |
virtual unsigned int | GetNumCoordsPosLevel () const override |
Get the number of degrees of freedom. | |
virtual unsigned int | GetLoadableNumCoordsPosLevel () override |
Gets the number of DOFs affected by this element (position part) | |
virtual unsigned int | GetLoadableNumCoordsVelLevel () override |
Gets the number of DOFs affected by this element (speed part) | |
virtual void | LoadableGetStateBlockPosLevel (int block_offset, ChState &mD) override |
Gets all the DOFs packed in a single vector (position part) | |
virtual void | LoadableGetStateBlockVelLevel (int block_offset, ChStateDelta &mD) override |
Gets all the DOFs packed in a single vector (speed part) | |
virtual void | LoadableStateIncrement (const unsigned int off_x, ChState &x_new, const ChState &x, const unsigned int off_v, const ChStateDelta &Dv) override |
Increment all DOFs using a delta. | |
virtual unsigned int | GetNumFieldCoords () override |
Number of coordinates in the interpolated field, ex=3 for a tetrahedron finite element or a cable, etc. More... | |
virtual unsigned int | GetNumSubBlocks () override |
Get the number of DOFs sub-blocks. | |
virtual unsigned int | GetSubBlockOffset (unsigned int nblock) override |
Get the offset of the specified sub-block of DOFs in global vector. | |
virtual unsigned int | GetSubBlockSize (unsigned int nblock) override |
Get the size of the specified sub-block of DOFs in global vector. | |
virtual bool | IsSubBlockActive (unsigned int nblock) const override |
Check if the specified sub-block of DOFs is active. | |
virtual void | LoadableGetVariables (std::vector< ChVariables * > &mvars) override |
Get the pointers to the contained ChVariables, appending to the mvars vector. | |
virtual void | ComputeNF (const double U, const double V, const double W, ChVectorDynamic<> &Qi, double &detJ, const ChVectorDynamic<> &F, ChVectorDynamic<> *state_x, ChVectorDynamic<> *state_w) override |
Evaluate Q=N'*F , for Q generalized lagrangian load, where N is some type of matrix evaluated at point P(U,V,W) assumed in absolute coordinates, and F is a load assumed in absolute coordinates. More... | |
virtual double | GetDensity () override |
This is not needed because not used in quadrature. | |
virtual void | ArchiveOut (ChArchiveOut &archive_out) override |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIn (ChArchiveIn &archive_in) override |
Method to allow de serialization of transient data from archives. | |
![]() | |
ChNodeBase (const ChNodeBase &other) | |
ChNodeBase & | operator= (const ChNodeBase &other) |
virtual unsigned int | GetNumCoordsVelLevel () const |
Get the number of degrees of freedom, derivative. More... | |
virtual unsigned int | GetNumCoordsPosLevelActive () const |
Get the actual number of active degrees of freedom. More... | |
virtual unsigned int | GetNumCoordsVelLevelActive () const |
Get the actual number of active degrees of freedom, derivative. More... | |
virtual bool | IsAllCoordsActive () const |
Return true if all node DOFs are active (no node variable is fixed). | |
unsigned int | NodeGetOffsetPosLevel () |
Get offset in the state vector (position part). | |
unsigned int | NodeGetOffsetVelLevel () |
Get offset in the state vector (speed part). | |
void | NodeSetOffsetPosLevel (const unsigned int moff) |
Set offset in the state vector (position part). | |
void | NodeSetOffsetVelLevel (const unsigned int moff) |
Set offset in the state vector (speed part). | |
virtual void | NodeIntStateGather (const unsigned int off_x, ChState &x, const unsigned int off_v, ChStateDelta &v, double &T) |
virtual void | NodeIntStateScatter (const unsigned int off_x, const ChState &x, const unsigned int off_v, const ChStateDelta &v, const double T) |
virtual void | NodeIntStateGatherAcceleration (const unsigned int off_a, ChStateDelta &a) |
virtual void | NodeIntStateScatterAcceleration (const unsigned int off_a, const ChStateDelta &a) |
virtual void | NodeIntStateIncrement (const unsigned int off_x, ChState &x_new, const ChState &x, const unsigned int off_v, const ChStateDelta &Dv) |
virtual void | NodeIntStateGetIncrement (const unsigned int off_x, const ChState &x_new, const ChState &x, const unsigned int off_v, ChStateDelta &Dv) |
virtual void | NodeIntLoadResidual_F (const unsigned int off, ChVectorDynamic<> &R, const double c) |
virtual void | NodeIntLoadResidual_Mv (const unsigned int off, ChVectorDynamic<> &R, const ChVectorDynamic<> &w, const double c) |
virtual void | NodeIntLoadLumpedMass_Md (const unsigned int off, ChVectorDynamic<> &Md, double &error, const double c) |
virtual void | NodeIntToDescriptor (const unsigned int off_v, const ChStateDelta &v, const ChVectorDynamic<> &R) |
virtual void | NodeIntFromDescriptor (const unsigned int off_v, ChStateDelta &v) |
virtual void | InjectVariables (ChSystemDescriptor &descriptor) |
Register with the given system descriptor any ChVariable objects associated with this item. | |
virtual void | VariablesFbReset () |
Set the 'fb' part (the known term) of the encapsulated ChVariables to zero. | |
virtual void | VariablesFbLoadForces (double factor=1) |
Add the current forces (applied to node) into the encapsulated ChVariables. More... | |
virtual void | VariablesQbLoadSpeed () |
Initialize the 'qb' part of the ChVariables with the current value of speeds. | |
virtual void | VariablesFbIncrementMq () |
Add M*q (masses multiplied current 'qb') to Fb, ex. More... | |
virtual void | VariablesQbSetSpeed (double step=0) |
Fetch the item speed (ex. More... | |
virtual void | VariablesQbIncrementPosition (double step) |
Increment node positions by the 'qb' part of the ChVariables, multiplied by a 'step' factor. More... | |
![]() | |
virtual bool | IsTetrahedronIntegrationNeeded () |
If true, use quadrature over u,v,w in [0..1] range as tetrahedron volumetric coords (with z=1-u-v-w) otherwise use default quadrature over u,v,w in [-1..+1] as box isoparametric coords. | |
virtual bool | IsTrianglePrismIntegrationNeeded () |
If true, use quadrature over u,v in [0..1] range as triangle natural coords (with z=1-u-v), and use linear quadrature over w in [-1..+1], otherwise use default quadrature over u,v,w in [-1..+1] as box isoparametric coords. | |
Public Attributes | |
ChVector3d | pos |
ChVector3d | pos_dt |
ChVector3d | pos_dtdt |
Additional Inherited Members | |
![]() | |
unsigned int | offset_x |
offset in vector of state (position part) | |
unsigned int | offset_w |
offset in vector of state (speed part) | |
Member Function Documentation
◆ ComputeNF()
|
overridevirtual |
Evaluate Q=N'*F , for Q generalized lagrangian load, where N is some type of matrix evaluated at point P(U,V,W) assumed in absolute coordinates, and F is a load assumed in absolute coordinates.
The det[J] is unused.
- Parameters
-
U x coordinate of application point in absolute space V y coordinate of application point in absolute space W z coordinate of application point in absolute space Qi Return result of N'*F here, maybe with offset block_offset detJ Return det[J] here F Input F vector, size is 3, it is Force x,y,z in absolute coords. state_x if != 0, update state (pos. part) to this, then evaluate Q state_w if != 0, update state (speed part) to this, then evaluate Q
Implements chrono::ChLoadableUVW.
Reimplemented in chrono::fea::ChNodeFEAxyzDDD, chrono::fea::ChNodeFEAxyzDD, and chrono::fea::ChNodeFEAxyzD.
◆ GetNumFieldCoords()
|
inlineoverridevirtual |
Number of coordinates in the interpolated field, ex=3 for a tetrahedron finite element or a cable, etc.
Here is 6: xyz displ + xyz rots
Implements chrono::ChLoadable.
Reimplemented in chrono::fea::ChNodeFEAxyzDDD, chrono::fea::ChNodeFEAxyzDD, and chrono::fea::ChNodeFEAxyzD.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/physics/ChNodeXYZ.h
- /builds/uwsbel/chrono/src/chrono/physics/ChNodeXYZ.cpp