Description
A helper class to provide some basic mechanism of C++ reflection (introspection).
Properties of objects can be accessed/listed as name-value pairs. Names are those provided in the CHNVP pairs in ArchiveOut/ArchiveIn. As this is a quite simple reflection system, the value is always passed as void*, it is up to you to do the proper static_cast<> to the class of the object. There are some shortcuts though, to access double, int and other fundamental types with a single statement.
#include <ChObjectExplorer.h>
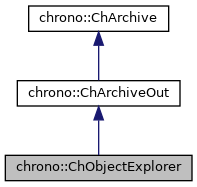
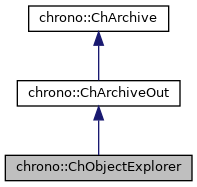
Public Member Functions | |
template<class T , class P > | |
bool | FetchValue (P &val, const T &root, const std::string &property_name) |
Search a property in "root" and directly assign it to "vl". More... | |
template<class T > | |
std::vector< ChValue * > & | FetchValues (T &root, const std::string &value_name) |
Search one or more values in "root" and return reference to a vector with results. More... | |
std::vector< ChValue * > & | FetchValues (ChValue &root, const std::string &value_name) |
Same as generic FetchValues(), but specialized for the case of finding sub ChValue properties of an already found ChValue. | |
std::vector< ChValue * > & | GetFetchResults () |
Access the results of the last search, ex. after FetchValues() | |
template<class T > | |
bool | IsObject (const T &root) |
Tell if "root" has sub values (i.e. More... | |
bool | IsObject (ChValue &root) |
Tell if "root" has sub values (i.e. More... | |
void | SetUseWildcards (const bool mw) |
bool | GetUseWildcards () |
void | SetUseUserNames (const bool mw) |
bool | GetUseUserNames () |
virtual void | out (ChNameValue< bool > bVal) |
virtual void | out (ChNameValue< int > bVal) |
virtual void | out (ChNameValue< double > bVal) |
virtual void | out (ChNameValue< float > bVal) |
virtual void | out (ChNameValue< unsigned int > bVal) |
virtual void | out (ChNameValue< unsigned long > bVal) |
virtual void | out (ChNameValue< unsigned long long > bVal) |
virtual void | out (ChNameValue< ChEnumMapperBase > bVal) |
virtual void | out (ChNameValue< char > bVal) |
virtual void | out (ChNameValue< std::string > bVal) |
virtual void | out_array_pre (ChValue &bVal, size_t msize) |
virtual void | out_array_between (ChValue &bVal, size_t msize) |
virtual void | out_array_end (ChValue &bVal, size_t msize) |
virtual void | out (ChValue &bVal, bool tracked, size_t obj_ID) |
virtual void | out_ref (ChValue &bVal, bool already_inserted, size_t obj_ID, size_t ext_ID) |
![]() | |
void | SetCutAllPointers (bool mcut) |
If you enable SetCutAllPointers(true), no serialization happens for objects referenced via pointers. More... | |
std::unordered_set< void * > & | CutPointers () |
Access the container of pointers that must not be serialized. More... | |
void | UnbindExternalPointer (void *mptr, size_t ID) |
Use the following to declare pointer(s) that must not be de-serialized but rather be 'unbind' and be saved just as unique IDs. More... | |
void | UnbindExternalPointer (std::shared_ptr< void > mptr, size_t ID) |
Use the following to declare pointer(s) that must not be de-serialized but rather be 'unbind' and be saved just as unique IDs. More... | |
virtual void | out (ChNameValue< FunGetSet< bool >> bVal) const |
virtual void | out (ChNameValue< FunGetSet< int >> bVal) const |
virtual void | out (ChNameValue< FunGetSet< double >> bVal) const |
virtual void | out (ChNameValue< FunGetSet< float >> bVal) const |
virtual void | out (ChNameValue< FunGetSet< char >> bVal) const |
virtual void | out (ChNameValue< FunGetSet< unsigned int >> bVal) const |
virtual void | out (ChNameValue< FunGetSet< const char * >> bVal) const |
virtual void | out (ChNameValue< FunGetSet< unsigned long >> bVal) const |
virtual void | out (ChNameValue< FunGetSet< unsigned long long >> bVal) const |
template<class T > | |
void | out (ChNameValue< ChEnumMapper< T >> bVal) |
template<class T , size_t N> | |
void | out (ChNameValue< T[N]> bVal) |
template<class T > | |
void | out (ChNameValue< std::vector< T >> bVal) |
template<class T > | |
void | out (ChNameValue< std::list< T >> bVal) |
template<class T , class Tv > | |
void | out (ChNameValue< std::pair< T, Tv >> bVal) |
template<class T , class Tv > | |
void | out (ChNameValue< std::unordered_map< T, Tv >> bVal) |
template<class T , class Tv > | |
void | out (ChNameValue< std::map< T, Tv >> bVal) |
template<class T > | |
void | out (ChNameValue< std::shared_ptr< T >> bVal) |
template<class T > | |
void | out (ChNameValue< T * > bVal) |
template<class T > | |
void | out (ChNameValue< T > bVal) |
template<class T > | |
ChArchiveOut & | operator<< (ChNameValue< T > bVal) |
Operator to allow easy serialization as myarchive << mydata;. | |
void | VersionWrite (int iv) |
template<class T > | |
void | VersionWrite () |
![]() | |
void | SetUseVersions (bool muse) |
Turn off version info in archives. More... | |
void | SetClusterClassVersions (bool mcl) |
If true, the version number is not saved in each class. More... | |
Protected Member Functions | |
void | ClearSearch () |
void | PrepareSearch (const std::string &msearched_property) |
int | wildcard_compare (const char *wildcard, const char *string) |
bool | MatchName (const std::string &token, const std::string &string) |
![]() | |
void | PutPointer (void *object, bool &already_stored, size_t &obj_ID) |
Find a pointer in pointer map: eventually add it to map if it was not previously inserted. More... | |
virtual void | out_version (int mver, const std::type_index mtypeid) |
Protected Attributes | |
std::string | searched_property |
std::vector< std::string > | search_tokens |
std::vector< ChValue * > | results |
bool | found |
bool | find_all |
bool | found_obj |
int | tablevel |
bool | use_wildcards |
bool | use_user_names |
std::vector< bool > | in_array |
![]() | |
std::unordered_map< void *, size_t > | internal_ptr_id |
size_t | currentID |
std::unordered_map< void *, size_t > | external_ptr_id |
std::unordered_set< void * > | cut_pointers |
bool | cut_all_pointers |
![]() | |
bool | cluster_class_versions |
std::unordered_map< std::type_index, int > | class_versions |
bool | use_versions |
Additional Inherited Members | |
![]() | |
template<class T > | |
using | FunGetSet = std::pair< std::function< T(void)>, std::function< void(T)> > |
Member Function Documentation
◆ FetchValue()
|
inline |
Search a property in "root" and directly assign it to "vl".
The property is searched by the "property_name", returning true if it matches the names you used in the CHNVP macros of ArchiveOut of your classes, and if the type of "val" is the same of the property (exactly the same type: no superclass/subclass). Note: the "root" object should be a class with sub properties and/or sub objects, and the root is not part of the search. The search string "property_name" can explore subobjects with "/", as in "employee/force/z" The search string "property_name" can contain wildcards * and ?, as in "e??loyee/*‍/z" In case of multiple matching properties it returns at the first found property.
◆ FetchValues()
|
inline |
Search one or more values in "root" and return reference to a vector with results.
The properties are searched by the "value_name", returning true if it matches the names you used in the CHNVP macros of ArchiveOut of your classes Note: the "root" object should be a class with sub properties and/or sub objects, and the root is not part of the search. Wildcards * and ? can be used. For example use property_name = "*" to ge the list of all subproperties of root object.
◆ IsObject() [1/2]
|
inline |
Tell if "root" has sub values (i.e.
"root" is a class with ArchiveOut implemented or a std container such as std::vector).
◆ IsObject() [2/2]
|
inline |
Tell if "root" has sub values (i.e.
"root" is a class with ArchiveOut implemented or a std container such as std::vector).
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/serialization/ChObjectExplorer.h
- /builds/uwsbel/chrono/src/chrono/serialization/ChObjectExplorer.cpp