Description
An iterative solver based on modified Krylov iteration of MINRES type with gradient projections (similar to nonlinear CG with Polyak-Ribiere).
The PMINRES solver can use diagonal precondityioning (enabled by default).
See ChSystemDescriptor for more information about the problem formulation and the data structures passed to the solver.
- Deprecated:
- Use ChSolverMINRES instead. This class will be removed in a future Chrono release.
#include <ChSolverPMINRES.h>
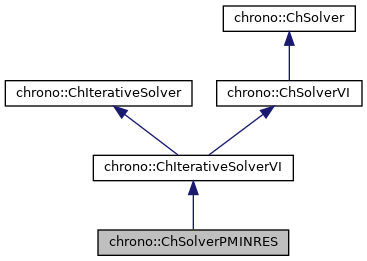
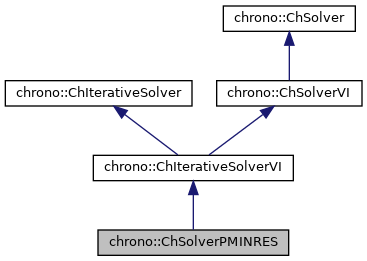
Public Member Functions | |
virtual Type | GetType () const override |
Return type of the solver. | |
virtual double | Solve (ChSystemDescriptor &sysd) override |
Performs the solution of the problem. More... | |
double | Solve_SupportingStiffness (ChSystemDescriptor &sysd) |
Same as Solve(), but this also supports the presence of ChKRMBlock blocks. More... | |
void | SetGradDiffStep (double mf) |
For the case where inequalities are introduced, the gradient is projected. More... | |
double | GetGradDiffStep () |
void | SetRelTolerance (double mrt) |
Set relative tolerance. More... | |
double | GetRelTolerance () |
virtual double | GetError () const override |
Return the tolerance error reached during the last solve. More... | |
virtual void | ArchiveOut (ChArchiveOut &archive_out) override |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIn (ChArchiveIn &archive_in) override |
Method to allow de-serialization of transient data from archives. | |
![]() | |
void | SetOmega (double mval) |
Set the overrelaxation factor (default: 1.0). More... | |
void | SetSharpnessLambda (double mval) |
Set the sharpness factor (default: 1.0). More... | |
virtual void | SetMaxIterations (int max_iterations) override |
Set the maximum number of iterations. | |
void | SetRecordViolation (bool mval) |
Enable/disable recording of the constraint violation history. More... | |
double | GetOmega () const |
Return the current value of the overrelaxation factor. | |
double | GetSharpnessLambda () const |
Return the current value of the sharpness factor. | |
virtual int | GetIterations () const override |
Return the number of iterations performed during the last solve. | |
const std::vector< double > & | GetViolationHistory () const |
Access the vector of constraint violation history. More... | |
const std::vector< double > & | GetDeltalambdaHistory () const |
Access the vector with history of maximum change in Lagrange multipliers Note that collection of constraint violations must be enabled through SetRecordViolation. | |
![]() | |
void | SetTolerance (double tolerance) |
Set the tolerance threshold used by the stopping criteria. | |
void | EnableDiagonalPreconditioner (bool val) |
Enable/disable use of a simple diagonal preconditioner (default: true). More... | |
void | EnableWarmStart (bool val) |
Enable/disable warm starting by providing an initial guess (default: false). More... | |
int | GetMaxIterations () const |
Get the current maximum number of iterations. | |
double | GetTolerance () const |
Get the current tolerance value. | |
![]() | |
virtual ChDirectSolverLS * | AsDirect () |
Downcast to ChDirectSolver. | |
virtual bool | Setup (ChSystemDescriptor &sysd) |
This function does the setup operations for the solver. More... | |
void | SetVerbose (bool mv) |
Set verbose output from solver. | |
void | EnableWrite (bool val, const std::string &frame, const std::string &out_dir=".") |
Enable/disable debug output of matrix, RHS, and solution vector. | |
Additional Inherited Members | |
![]() | |
enum | Type { Type::PSOR, Type::PSSOR, Type::PJACOBI, Type::PMINRES, Type::BARZILAIBORWEIN, Type::APGD, Type::ADMM, Type::SPARSE_LU, Type::SPARSE_QR, Type::PARDISO_MKL, Type::MUMPS, Type::GMRES, Type::MINRES, Type::BICGSTAB, CUSTOM } |
Available types of solvers. More... | |
![]() | |
static std::string | GetTypeAsString (Type type) |
Return the solver type as a string. | |
![]() | |
virtual bool | IsIterative () const override |
Return true if iterative solver. | |
virtual bool | IsDirect () const override |
Return true if direct solver. | |
virtual ChIterativeSolver * | AsIterative () override |
Downcast to ChIterativeSolver. | |
void | AtIterationEnd (double mmaxviolation, double mdeltalambda, unsigned int iternum) |
This method MUST be called by all iterative methods INSIDE their iteration loops (at the end). More... | |
virtual bool | SolveRequiresMatrix () const override |
Indicate whether ot not the Solve() phase requires an up-to-date problem matrix. More... | |
![]() | |
ChIterativeSolver (int max_iterations, double tolerance, bool use_precond, bool warm_start) | |
void | WriteMatrices (ChSystemDescriptor &sysd, bool one_indexed=true) |
double | CheckSolution (ChSystemDescriptor &sysd, const ChVectorDynamic<> &x) |
![]() | |
int | m_iterations |
number of iterations performed by the solver during last call to Solve() | |
double | m_omega |
over-relaxation factor | |
double | m_shlambda |
sharpness factor | |
bool | record_violation_history |
std::vector< double > | violation_history |
std::vector< double > | dlambda_history |
![]() | |
bool | m_use_precond |
use diagonal preconditioning? | |
bool | m_warm_start |
use initial guesss? | |
int | m_max_iterations |
maximum number of iterations | |
double | m_tolerance |
tolerance threshold in stopping criteria | |
![]() | |
bool | verbose |
bool | write_matrix |
std::string | output_dir |
std::string | frame_id |
Member Function Documentation
◆ GetError()
|
inlineoverridevirtual |
Return the tolerance error reached during the last solve.
For the PMINRES solver, this is the norm of the projected residual.
Implements chrono::ChIterativeSolver.
◆ SetGradDiffStep()
|
inline |
For the case where inequalities are introduced, the gradient is projected.
A numerical differentiation is used, this is the delta.
◆ SetRelTolerance()
|
inline |
Set relative tolerance.
the iteration stops when the (projected) residual r is smaller than absolute tolerance, that you set via SetTolerance(), OR it is smaller than 'rhs_rel_tol' i.e. the norm of the right hand side multiplied by this relative tolerance. Set to 0 if you do not want relative tolerance to enter into play.
◆ Solve()
|
overridevirtual |
Performs the solution of the problem.
- Returns
- the maximum constraint violation after termination.
- Parameters
-
sysd system description with constraints and variables
Implements chrono::ChSolver.
◆ Solve_SupportingStiffness()
double chrono::ChSolverPMINRES::Solve_SupportingStiffness | ( | ChSystemDescriptor & | sysd | ) |
Same as Solve(), but this also supports the presence of ChKRMBlock blocks.
If Solve() is called and stiffness is present, Solve() automatically falls back to this function. It does not solve the Schur complement N*l-r=0 as Solve does, here the entire system KKT matrix with duals l and primals q is used.
double numerator = mr.MatrDot(mz,mZMr); // 1) r'* Z r double denominator = mr.MatrDot(mz_old,mZMr_old); // 2) r_old' Z *r_old
- Parameters
-
sysd system description with constraints and variables
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/solver/ChSolverPMINRES.h
- /builds/uwsbel/chrono/src/chrono/solver/ChSolverPMINRES.cpp