Description
Implementation of the HHT implicit integrator for II order systems.
This timestepper allows use of an adaptive time-step, as well as optional use of a modified Newton scheme for the solution of the resulting nonlinear problem.
#include <ChTimestepperHHT.h>
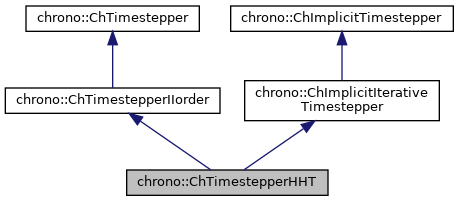

Public Member Functions | |
ChTimestepperHHT (ChIntegrableIIorder *intgr=nullptr) | |
virtual Type | GetType () const override |
Return type of the integration method. | |
void | SetAlpha (double val) |
Set the numerical damping parameter (in the [-1/3, 0] range). More... | |
double | GetAlpha () |
Return the current value of the method parameter alpha. | |
void | SetStepControl (bool enable) |
Turn on/off the internal step size control. More... | |
void | SetMinStepSize (double step) |
Set the minimum step size. More... | |
void | SetMaxItersSuccess (int iters) |
Set the maximum allowable number of iterations for counting a step towards a stepsize increase. More... | |
void | SetRequiredSuccessfulSteps (int num_steps) |
Set the minimum number of (internal) steps that use at most maxiters_success before considering a stepsize increase. More... | |
void | SetStepIncreaseFactor (double factor) |
Set the multiplicative factor for a stepsize increase (must be larger than 1). More... | |
void | SetStepDecreaseFactor (double factor) |
Set the multiplicative factor for a stepsize decrease (must be smaller than 1). More... | |
void | SetModifiedNewton (bool enable) |
Enable/disable modified Newton. More... | |
virtual void | Advance (const double dt) override |
Perform an integration timestep, by advancing the state by the specified time step. | |
double | GetEstimatedConvergenceRate () const |
Get the last estimated convergence rate for the internal Newton solver. More... | |
virtual void | ArchiveOut (ChArchiveOut &archive) override |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIn (ChArchiveIn &archive) override |
Method to allow de-serialization of transient data from archives. | |
![]() | |
ChTimestepperIIorder (ChIntegrableIIorder *intgr=nullptr) | |
Constructor. | |
virtual | ~ChTimestepperIIorder () |
Destructor. | |
virtual ChState & | GetStatePos () |
Access the state, position part, at current time. | |
virtual ChStateDelta & | GetStateVel () |
Access the state, speed part, at current time. | |
virtual ChStateDelta & | GetStateAcc () |
Access the acceleration, at current time. | |
virtual void | SetIntegrable (ChIntegrableIIorder *intgr) |
Set the integrable object. | |
![]() | |
ChTimestepper (ChIntegrable *intgr=nullptr) | |
Constructor. | |
virtual | ~ChTimestepper () |
Destructor. | |
virtual ChVectorDynamic & | GetLagrangeMultipliers () |
Access the lagrangian multipliers, if any. | |
virtual void | SetIntegrable (ChIntegrable *intgr) |
Set the integrable object. | |
ChIntegrable * | GetIntegrable () |
Get the integrable object. | |
virtual double | GetTime () const |
Get the current time. | |
virtual void | SetTime (double mt) |
Set the current time. | |
void | SetVerbose (bool verb) |
Turn on/off logging of messages. | |
![]() | |
void | SetMaxIters (int iters) |
Set the max number of iterations using the Newton Raphson procedure. | |
double | GetMaxIters () |
Get the max number of iterations using the Newton Raphson procedure. | |
void | SetRelTolerance (double rel_tol) |
Set the relative tolerance. More... | |
void | SetAbsTolerances (double abs_tolS, double abs_tolL) |
Set the absolute tolerances. More... | |
void | SetAbsTolerances (double abs_tol) |
Set the absolute tolerances. More... | |
unsigned int | GetNumIterations () const |
Return the number of iterations. | |
unsigned int | GetNumSetupCalls () const |
Return the number of calls to the solver's Setup function. | |
unsigned int | GetNumSolveCalls () const |
Return the number of calls to the solver's Solve function. | |
Additional Inherited Members | |
![]() | |
enum | Type { EULER_IMPLICIT_LINEARIZED = 0, EULER_IMPLICIT_PROJECTED = 1, EULER_IMPLICIT = 2, TRAPEZOIDAL = 3, TRAPEZOIDAL_LINEARIZED = 4, HHT = 5, HEUN = 6, RUNGEKUTTA45 = 7, EULER_EXPLICIT = 8, LEAPFROG = 9, NEWMARK = 10, CUSTOM = 20 } |
Available methods for time integration (time steppers). | |
![]() | |
ChState | X |
ChStateDelta | V |
ChStateDelta | A |
![]() | |
ChIntegrable * | integrable |
double | T |
ChVectorDynamic | L |
bool | verbose |
bool | Qc_do_clamp |
double | Qc_clamping |
![]() | |
unsigned int | maxiters |
maximum number of iterations | |
double | reltol |
relative tolerance | |
double | abstolS |
absolute tolerance (states) | |
double | abstolL |
absolute tolerance (Lagrange multipliers) | |
unsigned int | numiters |
number of iterations | |
unsigned int | numsetups |
number of calls to the solver's Setup function | |
unsigned int | numsolves |
number of calls to the solver's Solve function | |
Member Function Documentation
◆ GetEstimatedConvergenceRate()
|
inline |
Get the last estimated convergence rate for the internal Newton solver.
Note that an estimate can only be calculated after the 3rd iteration. For the first 2 iterations, the convergence rate estimate is set to 1.
◆ SetAlpha()
void chrono::ChTimestepperHHT::SetAlpha | ( | double | val | ) |
Set the numerical damping parameter (in the [-1/3, 0] range).
The closer to -1/3, the more damping. The closer to 0, the less damping (for 0, it is the trapezoidal method). The method coefficients gamma and beta are set automatically, based on alpha. Default: -0.2.
◆ SetMaxItersSuccess()
|
inline |
Set the maximum allowable number of iterations for counting a step towards a stepsize increase.
Default: 3.
◆ SetMinStepSize()
|
inline |
Set the minimum step size.
An exception is thrown if the internal step size decreases below this limit. Default: 1e-10.
◆ SetModifiedNewton()
|
inline |
Enable/disable modified Newton.
If enabled, the Newton matrix is evaluated, assembled, and factorized only once per step or if the Newton iteration does not converge with an out-of-date matrix. If disabled, the Newton matrix is evaluated at every iteration of the nonlinear solver. Default: true.
◆ SetRequiredSuccessfulSteps()
|
inline |
Set the minimum number of (internal) steps that use at most maxiters_success before considering a stepsize increase.
Default: 5.
◆ SetStepControl()
|
inline |
Turn on/off the internal step size control.
Default: true.
◆ SetStepDecreaseFactor()
|
inline |
Set the multiplicative factor for a stepsize decrease (must be smaller than 1).
Default: 0.5.
◆ SetStepIncreaseFactor()
|
inline |
Set the multiplicative factor for a stepsize increase (must be larger than 1).
Default: 2.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/timestepper/ChTimestepperHHT.h
- /builds/uwsbel/chrono/src/chrono/timestepper/ChTimestepperHHT.cpp