Description
Performs a step of a Leapfrog explicit integrator.
This is a symplectic method, with 2nd order accuracy, at least when F depends on positions only. Note: uses last step acceleration: changing or resorting the numbering of DOFs will invalidate it. Suggestion: use the ChTimestepperEulerSemiImplicit, it gives the same accuracy with better performance.
#include <ChTimestepper.h>
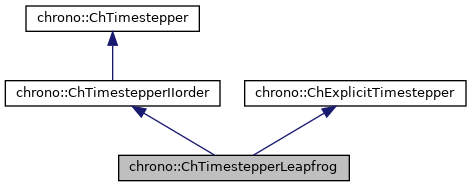
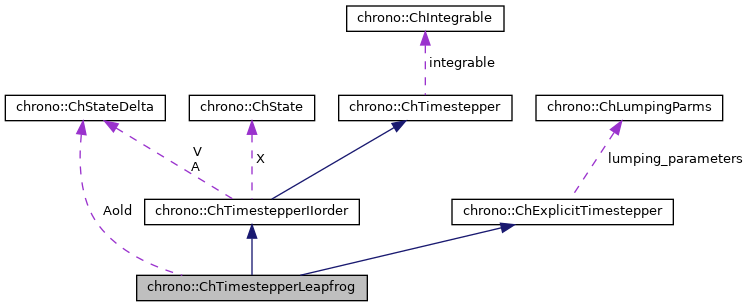
Public Member Functions | |
ChTimestepperLeapfrog (ChIntegrableIIorder *intgr=nullptr) | |
Constructors (default empty) | |
virtual Type | GetType () const override |
Return type of the integration method. More... | |
virtual void | Advance (const double dt) override |
Performs an integration timestep. More... | |
virtual void | ArchiveOut (ChArchiveOut &archive) override |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIn (ChArchiveIn &archive) override |
Method to allow de-serialization of transient data from archives. | |
![]() | |
ChTimestepperIIorder (ChIntegrableIIorder *intgr=nullptr) | |
Constructor. | |
virtual | ~ChTimestepperIIorder () |
Destructor. | |
virtual ChState & | GetStatePos () |
Access the state, position part, at current time. | |
virtual ChStateDelta & | GetStateVel () |
Access the state, speed part, at current time. | |
virtual ChStateDelta & | GetStateAcc () |
Access the acceleration, at current time. | |
virtual void | SetIntegrable (ChIntegrableIIorder *intgr) |
Set the integrable object. | |
![]() | |
ChTimestepper (ChIntegrable *intgr=nullptr) | |
Constructor. | |
virtual | ~ChTimestepper () |
Destructor. | |
virtual ChVectorDynamic & | GetLagrangeMultipliers () |
Access the lagrangian multipliers, if any. | |
virtual void | SetIntegrable (ChIntegrable *intgr) |
Set the integrable object. | |
ChIntegrable * | GetIntegrable () |
Get the integrable object. | |
virtual double | GetTime () const |
Get the current time. | |
virtual void | SetTime (double mt) |
Set the current time. | |
void | SetVerbose (bool verb) |
Turn on/off logging of messages. | |
![]() | |
void | SetDiagonalLumpingON (double Ck=1000, double Cr=0) |
Turn on the diagonal lumping. More... | |
void | SetDiagonalLumpingOFF () |
Turn off the diagonal lumping (default is off) | |
double | GetLumpingError () |
Gets the diagonal lumping error done last time the integrator has been called. | |
void | ResetLumpingError () |
Resets the diagonal lumping error. | |
Protected Attributes | |
ChStateDelta | Aold |
![]() | |
ChState | X |
ChStateDelta | V |
ChStateDelta | A |
![]() | |
ChIntegrable * | integrable |
double | T |
ChVectorDynamic | L |
bool | verbose |
bool | Qc_do_clamp |
double | Qc_clamping |
![]() | |
ChLumpingParms * | lumping_parameters |
Additional Inherited Members | |
![]() | |
enum | Type { EULER_IMPLICIT_LINEARIZED, EULER_IMPLICIT_PROJECTED, EULER_IMPLICIT, TRAPEZOIDAL, TRAPEZOIDAL_LINEARIZED, HHT, HEUN, RUNGEKUTTA45, EULER_EXPLICIT, LEAPFROG, NEWMARK, CUSTOM } |
Available methods for time integration (time steppers). | |
![]() | |
static std::string | GetTypeAsString (Type type) |
Return the integrator type as a string. | |
Member Function Documentation
◆ Advance()
|
overridevirtual |
Performs an integration timestep.
- Parameters
-
dt timestep to advance
Implements chrono::ChTimestepper.
◆ GetType()
|
inlineoverridevirtual |
Return type of the integration method.
Default is CUSTOM. Derived classes should override this function.
Reimplemented from chrono::ChTimestepper.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/timestepper/ChTimestepper.h
- /builds/uwsbel/chrono/src/chrono/timestepper/ChTimestepper.cpp