chrono::fea::ChBeamSectionRayleighEasyRectangular Class Reference
Description
This works exactly as ChBeamSectionEulerEasyRectangular, but adds the effect of Jyy Jzz rotational sectional inertias.
#include <ChBeamSectionEuler.h>
Inheritance diagram for chrono::fea::ChBeamSectionRayleighEasyRectangular:
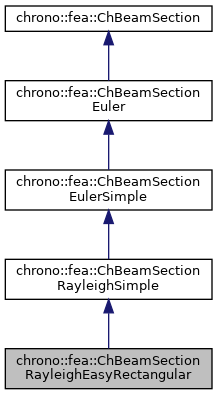
Collaboration diagram for chrono::fea::ChBeamSectionRayleighEasyRectangular:
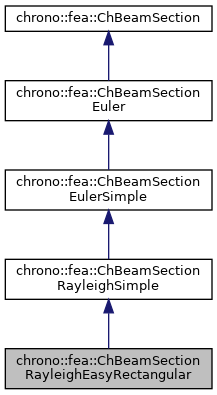
Public Member Functions | |
ChBeamSectionRayleighEasyRectangular (double width_y, double width_z, double E, double G, double density) | |
![]() | |
virtual void | ComputeInertiaMatrix (ChMatrix66d &M) override |
Compute the 6x6 sectional inertia matrix, as in {x_momentum,w_momentum}=[Mm]{xvel,wvel}. | |
virtual void | ComputeInertiaDampingMatrix (ChMatrix66d &Ri, const ChVector3d &mW) override |
Compute the 6x6 sectional inertia damping matrix [Ri] (gyroscopic matrix damping) More... | |
virtual void | ComputeInertiaStiffnessMatrix (ChMatrix66d &Ki, const ChVector3d &mWvel, const ChVector3d &mWacc, const ChVector3d &mXacc) override |
Compute the 6x6 sectional inertia stiffness matrix [Ki^]. More... | |
virtual void | ComputeQuadraticTerms (ChVector3d &mF, ChVector3d &mT, const ChVector3d &mW) override |
Compute the centrifugal term and gyroscopic term. | |
![]() | |
void | SetArea (const double ma) |
Set the cross sectional area A of the beam (m^2) | |
double | GetArea () const |
void | SetIyy (double ma) |
Set the Iyy moment of inertia of the beam (for flexion about y axis) Note: some textbook calls this Iyy as Iz. | |
double | GetIyy () const |
void | SetIzz (double ma) |
Set the Izz moment of inertia of the beam (for flexion about z axis) Note: some textbook calls this Izz as Iy. | |
double | GetIzz () const |
void | SetJ (double ma) |
Set the J torsion constant of the beam (for torsion about x axis) | |
double | GetJ () const |
void | SetKsy (double ma) |
Set the Timoshenko shear coefficient Ks for y shear, usually about 0.8, (for elements that use this, ex. More... | |
double | GetKsy () const |
void | SetKsz (double ma) |
Set the Timoshenko shear coefficient Ks for z shear, usually about 0.8, (for elements that use this, ex. More... | |
double | GetKsz () const |
void | SetAsRectangularSection (double width_y, double width_z) |
Shortcut: set Area, Ixx, Iyy, Ksy, Ksz and J torsion constant at once, given the y and z widths of the beam assumed with rectangular shape. | |
void | SetAsCircularSection (double diameter) |
Shortcut: set Area, Ixx, Iyy, Ksy, Ksz and J torsion constant at once, given the diameter of the beam assumed with circular shape. | |
void | SetDensity (double md) |
Set the density of the beam (kg/m^3) | |
double | GetDensity () const |
void | SetYoungModulus (double mE) |
Set E, the Young elastic modulus (N/m^2) | |
double | GetYoungModulus () const |
void | SetShearModulus (double mG) |
Set the shear modulus, used for computing the torsion rigidity = J*G. | |
double | GetShearModulus () const |
void | SetShearModulusFromPoisson (double mpoisson) |
Set the shear modulus, given current Young modulus and the specified Poisson ratio. | |
virtual double | GetAxialRigidity () const override |
Gets the axial rigidity, usually A*E. | |
virtual double | GetTorsionRigidityX () const override |
Gets the torsion rigidity, for torsion about X axis at elastic center, usually J*G. | |
virtual double | GetBendingRigidityY () const override |
Gets the bending rigidity, for bending about Y axis at elastic center, usually Iyy*E. | |
virtual double | GetBendingRigidityZ () const override |
Gets the bending rigidity, for bending about Z axis at elastic center, usually Izz*E. | |
virtual double | GetSectionRotation () const override |
Set the rotation of the Y Z section axes for which the YbendingRigidity and ZbendingRigidity are defined. | |
virtual double | GetCentroidY () const override |
Gets the Y position of the elastic center respect to centerline. | |
virtual double | GetCentroidZ () const override |
Gets the Z position of the elastic center respect to centerline. | |
virtual double | GetShearCenterY () const override |
Gets the Y position of the shear center respect to centerline. | |
virtual double | GetShearCenterZ () const override |
Gets the Z position of the shear center respect to centerline. | |
virtual double | GetMassPerUnitLength () const override |
Get mass per unit length, ex.SI units [kg/m]. | |
virtual double | GetInertiaJxxPerUnitLength () const override |
Get the Jxx component of the inertia per unit length (polar inertia) in the Y Z unrotated reference frame of the section at centerline. More... | |
![]() | |
virtual void | ComputeInertialForce (ChVector3d &mFi, ChVector3d &mTi, const ChVector3d &mWvel, const ChVector3d &mWacc, const ChVector3d &mXacc) |
Compute the total inertial forces (per unit length). More... | |
void | SetArtificialJyyJzzFactor (double mf) |
The Euler beam model has no rotational inertia per each section, assuming mass is concentrated on the centerline. More... | |
double | GetArtificialJyyJzzFactor () |
virtual void | SetRayleighDampingAlpha (double malpha) |
Set the "alpha" Rayleigh damping ratio, the mass-proportional structural damping in: R = alpha*M + beta*K. | |
double | GetRayleighDampingAlpha () |
virtual void | SetRayleighDampingBeta (double mbeta) |
Set the "beta" Rayleigh damping ratio, the stiffness-proportional structural damping in: R = alpha*M + beta*K. | |
double | GetRayleighDampingBeta () |
virtual void | SetRayleighDamping (double mbeta, double malpha=0) |
Set both beta and alpha coefficients in Rayleigh damping model: R = alpha*M + beta*K. More... | |
![]() | |
void | SetDrawShape (std::shared_ptr< ChBeamSectionShape > mshape) |
Set the graphical representation for this section. More... | |
std::shared_ptr< ChBeamSectionShape > | GetDrawShape () const |
Get the drawing shape of this section (i.e.a 2D profile used for drawing 3D tesselation and visualization) By default a thin square section, use SetDrawShape() to change it. | |
void | SetDrawThickness (double thickness_y, double thickness_z) |
Shortcut: adds a ChBeamSectionShapeRectangular for visualization as a centered rectangular beam, and sets its width/height. More... | |
void | SetDrawCircularRadius (double draw_rad) |
Shortcut: adds a ChBeamSectionShapeCircular for visualization as a centered circular beam, and sets its radius. More... | |
void | SetCircular (bool ic) |
OBSOLETE only for backward compability. | |
Additional Inherited Members | |
![]() | |
double | Area |
double | Iyy |
double | Izz |
double | J |
double | G |
double | E |
double | density |
double | Ks_y |
double | Ks_z |
![]() | |
bool | compute_inertia_damping_matrix = true |
Flag that turns on/off the computation of the [Ri] 'gyroscopic' inertial damping matrix. More... | |
bool | compute_inertia_stiffness_matrix = true |
Flag that turns on/off the computation of the [Ki] inertial stiffness matrix. More... | |
bool | compute_Ri_Ki_by_num_diff = false |
Flag for computing the Ri and Ki matrices via numerical differentiation even if an analytical expression is provided. More... | |
![]() | |
double | rdamping_beta |
double | rdamping_alpha |
double | JzzJyy_factor |
Constructor & Destructor Documentation
◆ ChBeamSectionRayleighEasyRectangular()
chrono::fea::ChBeamSectionRayleighEasyRectangular::ChBeamSectionRayleighEasyRectangular | ( | double | width_y, |
double | width_z, | ||
double | E, | ||
double | G, | ||
double | density | ||
) |
- Parameters
-
width_y width of section in y direction width_z width of section in z direction E Young modulus G Shear modulus (only needed for the torsion) density volumetric density (ex. in SI units: [kg/m^3])
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/fea/ChBeamSectionEuler.h
- /builds/uwsbel/chrono/src/chrono/fea/ChBeamSectionEuler.cpp