Description
Extension of FmuComponentBase class for Chrono FMUs.
#include <ChFmuToolsExport.h>
Inherits FmuComponentBase.
Inherited by FmuComponent, FmuComponent, FmuComponent, FmuComponent, and FmuComponent.
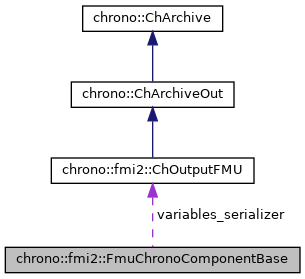
Public Member Functions | |
FmuChronoComponentBase (fmi2String instanceName, fmi2Type fmuType, fmi2String fmuGUID, fmi2String fmuResourceLocation, const fmi2CallbackFunctions *functions, fmi2Boolean visible, fmi2Boolean loggingOn) | |
void | AddFmuVecVariable (ChVector3d &v, const std::string &name, const std::string &unit_name, const std::string &description, FmuVariable::CausalityType causality=FmuVariable::CausalityType::local, FmuVariable::VariabilityType variability=FmuVariable::VariabilityType::continuous, FmuVariable::InitialType initial=FmuVariable::InitialType::none) |
Add FMU variables corresponding to the specified ChVector3d. More... | |
void | AddFmuQuatVariable (ChQuaternion<> &q, const std::string &name, const std::string &unit_name, const std::string &description, FmuVariable::CausalityType causality=FmuVariable::CausalityType::local, FmuVariable::VariabilityType variability=FmuVariable::VariabilityType::continuous, FmuVariable::InitialType initial=FmuVariable::InitialType::none) |
Add FMU variables corresponding to the specified ChQuaternion. More... | |
void | AddFmuCsysVariable (ChCoordsysd &s, const std::string &name, const std::string &unit_name, const std::string &description, FmuVariable::CausalityType causality=FmuVariable::CausalityType::local, FmuVariable::VariabilityType variability=FmuVariable::VariabilityType::continuous, FmuVariable::InitialType initial=FmuVariable::InitialType::none) |
Add FMU variables corresponding to the specified ChCoordsys. More... | |
void | AddFmuFrameVariable (ChFrame<> &s, const std::string &name, const std::string &unit_name, const std::string &description, FmuVariable::CausalityType causality=FmuVariable::CausalityType::local, FmuVariable::VariabilityType variability=FmuVariable::VariabilityType::continuous, FmuVariable::InitialType initial=FmuVariable::InitialType::none) |
Add FMU variables corresponding to the specified ChFrame. More... | |
void | AddFmuFrameMovingVariable (ChFrameMoving<> &s, const std::string &name, const std::string &unit_name, const std::string &unit_name_dt, const std::string &description, FmuVariable::CausalityType causality=FmuVariable::CausalityType::local, FmuVariable::VariabilityType variability=FmuVariable::VariabilityType::continuous, FmuVariable::InitialType initial=FmuVariable::InitialType::none) |
Add FMU variables corresponding to the specified ChFrameMoving. More... | |
void | AddFmuVisualShapes (const ChPhysicsItem &pi, std::string custom_pi_name="") |
Add FMU variables corresponding to the visual shapes attached to the specified ChPhysicsItem. More... | |
void | AddFmuVisualShapes (const ChAssembly &ass) |
Add FMU variables corresponding to the visual shapes attached to the specified ChAssembly. More... | |
Protected Types | |
using | VisTuple = std::tuple< ChFrame<>, const ChPhysicsItem *, const ChFrame<> *, bool > |
Protected Member Functions | |
void | addFmuVecVariable (ChVector3d &v, const std::string &name, const std::string &unit_name, const std::string &description, FmuVariable::CausalityType causality, FmuVariable::VariabilityType variability, FmuVariable::InitialType initial, bool cache) |
void | addFmuQuatVariable (ChQuaternion<> &q, const std::string &name, const std::string &unit_name, const std::string &description, FmuVariable::CausalityType causality, FmuVariable::VariabilityType variability, FmuVariable::InitialType initial, bool cache) |
void | addFmuCsysVariable (ChCoordsysd &s, const std::string &name, const std::string &unit_name, const std::string &description, FmuVariable::CausalityType causality, FmuVariable::VariabilityType variability, FmuVariable::InitialType initial, bool cache) |
void | addFmuFrameMovingVariable (ChFrameMoving<> &s, const std::string &name, const std::string &unit_name, const std::string &unit_name_dt, const std::string &description, FmuVariable::CausalityType causality, FmuVariable::VariabilityType variability, FmuVariable::InitialType initial, bool cache) |
virtual void | addDerivative (const std::string &derivative_name, const std::string &state_name, const std::vector< std::string > &dependency_names) override |
Add a declaration of a state derivative. More... | |
virtual void | addDependencies (const std::string &variable_name, const std::vector< std::string > &dependency_names) override |
Include a dependency of "variable_name" on "dependency_name". More... | |
Protected Attributes | |
std::unordered_set< std::string > | variables_vec |
list of ChVector3 "variables" | |
std::unordered_set< std::string > | variables_quat |
list of ChQuaternion "variables" | |
std::unordered_set< std::string > | variables_csys |
list of ChCoordsys "variables" | |
std::unordered_set< std::string > | variables_framem |
list of ChFrameMoving "variables" | |
ChOutputFMU | variables_serializer |
add variables to the FMU component by leveraging the serialization mechanism | |
std::unordered_map< fmi2Integer, VisTuple > | visualizer_frames |
Map of visualization global frames. More... | |
fmi2Integer | visualizers_counter = 0 |
total number of visualizers (not unsigned to keep compatibility with FMU standard types) | |
Static Protected Attributes | |
static const std::unordered_set< std::string > | supported_shape_types |
list of supported shapes for visualization, required to provide a memory position to getters of shape type More... | |
Member Function Documentation
◆ addDependencies()
|
inlineoverrideprotectedvirtual |
Include a dependency of "variable_name" on "dependency_name".
This version accounts for variables and/or dependencies corresponding to ChVector3, ChQuaternion, ChCoordsys, ChFrame, or ChFrameMoving types. These are expanded to the appropriate number of FMI scalar variables.
◆ addDerivative()
|
inlineoverrideprotectedvirtual |
Add a declaration of a state derivative.
This version accounts for states and state derivatives corresponding to ChVector3 or ChQuaternion types. These are expanded to the appropriate number of FMI scalar variables. Dependencies can include ChVector3, ChQuaternion, ChCoordsys, or ChFrameMoving variables.
◆ AddFmuCsysVariable()
|
inline |
Add FMU variables corresponding to the specified ChCoordsys.
This function creates 7 FMU variables, one for each component of the position ChVector3d and one for each component of the rotation quaternion, all of type FmuVariable::Type::Real.
◆ AddFmuFrameMovingVariable()
|
inline |
Add FMU variables corresponding to the specified ChFrameMoving.
This function creates 7 FMU variables for the pose, one for each component of the position ChVector3d and one for each component of the rotation quaternion, all of type FmuVariable::Type::Real. Additionally, 7 FMU variables are created to encode the position and orientation time derivatives.
◆ AddFmuFrameVariable()
|
inline |
Add FMU variables corresponding to the specified ChFrame.
This function creates 7 FMU variables, one for each component of the position ChVector3d and one for each component of the rotation quaternion, all of type FmuVariable::Type::Real.
◆ AddFmuQuatVariable()
|
inline |
Add FMU variables corresponding to the specified ChQuaternion.
This function creates 4 FMU variables, one for each component of the ChVector3d, with names "name.e0", "name.e1", "name.e2", and "name.e3", all of type FmuVariable::Type::Real.
◆ AddFmuVecVariable()
|
inline |
Add FMU variables corresponding to the specified ChVector3d.
This function creates 3 FMU variables, one for each component of the ChVector3d, with names "name.x", "name.y", and "name.z", all of type FmuVariable::Type::Real.
Member Data Documentation
◆ visualizer_frames
|
protected |
Map of visualization global frames.
Includes:
- visual shape frames (from shape local frame to global)
- ChPhysicsItem to which they are bounded
- (constant) ChFrame local to the shape
- flag to tell if the frame has been already updated in the current step
The documentation for this class was generated from the following file:
- /builds/uwsbel/chrono/src/chrono_fmi/fmi2/ChFmuToolsExport.h