chrono::geometry::ChTriangle Class Reference
Description
A triangle geometric shape for collisions and visualization.
#include <ChTriangle.h>
Inheritance diagram for chrono::geometry::ChTriangle:
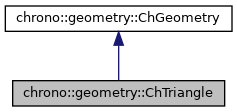
Collaboration diagram for chrono::geometry::ChTriangle:
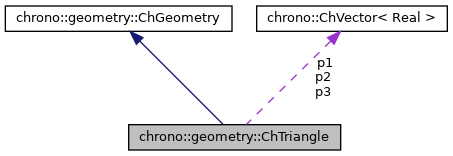
Public Member Functions | |
ChTriangle (const ChVector<> &P1, const ChVector<> &P2, const ChVector<> &P3) | |
ChTriangle (const ChTriangle &source) | |
virtual ChTriangle * | Clone () const override |
"Virtual" copy constructor (covariant return type). | |
ChTriangle & | operator= (const ChTriangle &source) |
Assignment operator: copy from another triangle. | |
virtual Type | GetClassType () const override |
Get the class type as an enum. | |
virtual ChAABB | GetBoundingBox () const override |
Compute bounding box of this triangle. | |
virtual ChVector | Baricenter () const override |
Compute center of mass. | |
virtual int | GetManifoldDimension () const override |
This is a surface. | |
bool | IsDegenerated () const |
bool | Normal (ChVector<> &N) const |
ChVector | GetNormal () const |
double | PointTriangleDistance (ChVector<> B, double &mu, double &mv, bool &is_into, ChVector<> &Bprojected) |
Given point B, computes the distance from this triangle plane, returning also the projection of point on the plane. More... | |
void | SetPoints (const ChVector<> &P1, const ChVector<> &P2, const ChVector<> &P3) |
Set the triangle vertices. | |
virtual void | ArchiveOut (ChArchiveOut &marchive) override |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIn (ChArchiveIn &marchive) override |
Method to allow de-serialization of transient data from archives. | |
![]() | |
ChGeometry (const ChGeometry &source) | |
void | InflateBoundingBox (ChAABB &bbox) const |
Enlarge the given existing bounding box with the bounding box of this object. | |
virtual double | GetBoundingSphereRadius () const |
Returns the radius of a bounding sphere for this geometry. More... | |
virtual void | Update () |
Generic update of internal data. | |
Static Public Member Functions | |
static ChAABB | GetBoundingBox (const ChVector<> &P1, const ChVector<> &P2, const ChVector<> &P3) |
Return the bounding box of a triangle with given vertices. | |
Public Attributes | |
ChVector | p1 |
first triangle vertex | |
ChVector | p2 |
second triangle vertex | |
ChVector | p3 |
third triangle vertex | |
Additional Inherited Members | |
![]() | |
enum | Type { NONE, SPHERE, ELLIPSOID, BOX, CYLINDER, TRIANGLE, CAPSULE, CONE, LINE, LINE_ARC, LINE_BEZIER, LINE_CAM, LINE_PATH, LINE_POLY, LINE_SEGMENT, ROUNDED_BOX, ROUNDED_CYLINDER, TRIANGLEMESH, TRIANGLEMESH_CONNECTED, TRIANGLEMESH_SOUP } |
Enumeration of geometric object types. | |
Member Function Documentation
◆ PointTriangleDistance()
double chrono::geometry::ChTriangle::PointTriangleDistance | ( | ChVector<> | B, |
double & | mu, | ||
double & | mv, | ||
bool & | is_into, | ||
ChVector<> & | Bprojected | ||
) |
Given point B, computes the distance from this triangle plane, returning also the projection of point on the plane.
- Parameters
-
B point to be measured mu returns U parametric coord of projection mv returns V parametric coord of projection is_into returns true if projection falls on the triangle Bprojected returns the position of the projected point
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/geometry/ChTriangle.h
- /builds/uwsbel/chrono/src/chrono/geometry/ChTriangle.cpp