Description
Base class communicator used to establish and facilitate communication between nodes.
#include <SynCommunicator.h>
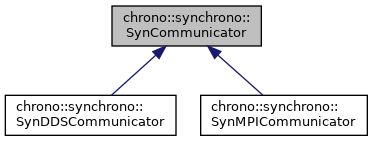
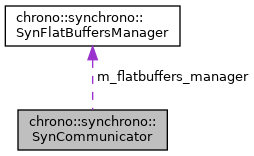
Public Member Functions | |
SynCommunicator () | |
Default constructor. More... | |
virtual | ~SynCommunicator () |
Destructor. More... | |
virtual void | Initialize () |
Initialization method typically responsible for establishing a connection. More... | |
virtual void | Synchronize ()=0 |
This method is responsible for continuous synchronous synchronization steps This method is the blocking form of the communication interface. More... | |
virtual void | Barrier ()=0 |
This method is responsible for blocking until an action is received or done. More... | |
void | Reset () |
Reset the communicator Will clear out message buffers. More... | |
void | AddOutgoingMessages (SynMessageList &messages) |
Add the messages to the outgoing message buffer. More... | |
void | AddQuitMessage () |
Adds a quit message to the queue telling other nodes to end the simulation. | |
void | AddIncomingMessages (SynMessageList &messages) |
Add the messages to the incoming message buffer. More... | |
void | ProcessBuffer (std::vector< uint8_t > &data) |
Process a data buffer by passing it to the underlying FlatBuffersManager. More... | |
virtual SynMessageList & | GetMessages () |
Get the messages received by the communicator. More... | |
Protected Attributes | |
bool | m_initialized |
whether the communicator has been initialized | |
SynMessageList | m_incoming_messages |
Incoming messages. | |
SynFlatBuffersManager | m_flatbuffers_manager |
flatbuffer manager for this rank | |
Constructor & Destructor Documentation
◆ SynCommunicator()
chrono::synchrono::SynCommunicator::SynCommunicator | ( | ) |
Default constructor.
◆ ~SynCommunicator()
|
virtual |
Destructor.
Member Function Documentation
◆ AddIncomingMessages()
void chrono::synchrono::SynCommunicator::AddIncomingMessages | ( | SynMessageList & | messages | ) |
Add the messages to the incoming message buffer.
- Parameters
-
messages a list of handles to messages to add to the incoming buffer
◆ AddOutgoingMessages()
void chrono::synchrono::SynCommunicator::AddOutgoingMessages | ( | SynMessageList & | messages | ) |
Add the messages to the outgoing message buffer.
- Parameters
-
messages a list of handles to messages to add to the outgoing buffer
◆ Barrier()
|
pure virtual |
This method is responsible for blocking until an action is received or done.
For example, a process may call Barrier to wait until another process has established certain classes and initialized certain quantities. This functionality should be implemented in this method.
Implemented in chrono::synchrono::SynDDSCommunicator, and chrono::synchrono::SynMPICommunicator.
◆ GetMessages()
|
inlinevirtual |
Get the messages received by the communicator.
- Returns
- SynMessageList the received messages
Reimplemented in chrono::synchrono::SynMPICommunicator.
◆ Initialize()
|
virtual |
Initialization method typically responsible for establishing a connection.
Although not necessarily true, this method should handle initial peer-to-peer communication. This could mean a simple handshake or an actual exchange of information used during the simulation.
Reimplemented in chrono::synchrono::SynDDSCommunicator, and chrono::synchrono::SynMPICommunicator.
◆ ProcessBuffer()
void chrono::synchrono::SynCommunicator::ProcessBuffer | ( | std::vector< uint8_t > & | data | ) |
Process a data buffer by passing it to the underlying FlatBuffersManager.
- Parameters
-
data the data to process
◆ Reset()
void chrono::synchrono::SynCommunicator::Reset | ( | ) |
Reset the communicator Will clear out message buffers.
◆ Synchronize()
|
pure virtual |
This method is responsible for continuous synchronous synchronization steps This method is the blocking form of the communication interface.
This method could use synchronous method calls to implement its communication interface. For asynchronous method cases, please use/implement Asynchronize()
Implemented in chrono::synchrono::SynDDSCommunicator, and chrono::synchrono::SynMPICommunicator.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_synchrono/communication/SynCommunicator.h
- /builds/uwsbel/chrono/src/chrono_synchrono/communication/SynCommunicator.cpp