Description
Base class for the chassis vehicle subsystem.
ChChassis is the base class for a main vehicle chassis (a single chassis or a front chassis).
#include <ChChassis.h>
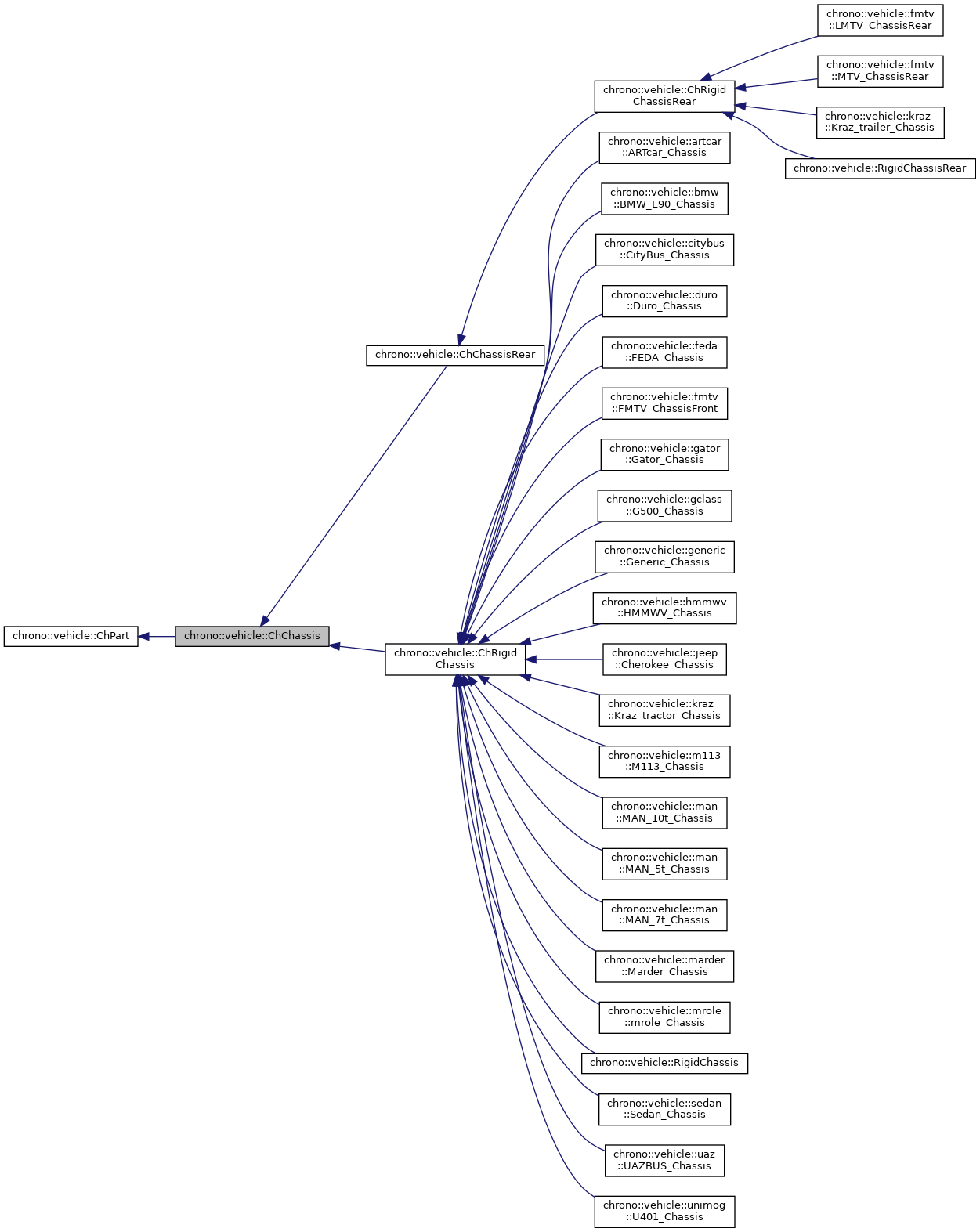
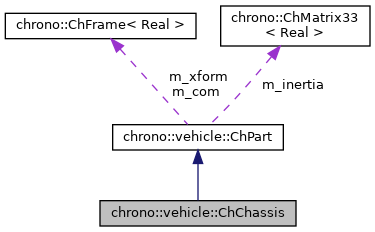
Classes | |
class | ExternalForceTorque |
Base class for a user-defined custom force/torque acting on the chassis body. More... | |
Public Member Functions | |
virtual uint16_t | GetVehicleTag () const override |
Get the tag of the associated vehicle. More... | |
virtual ChCoordsys | GetLocalDriverCoordsys () const =0 |
Get the local driver position and orientation. More... | |
virtual const ChVector3d | GetLocalPosRearConnector () const |
Get the location (in the local frame of this chassis) of the connection to a rear chassis. | |
std::shared_ptr< ChBodyAuxRef > | GetBody () const |
Get a handle to the vehicle's chassis body. | |
ChSystem * | GetSystem () const |
Get a pointer to the containing system. | |
const ChVector3d & | GetPos () const |
Get the global location of the chassis reference frame origin. | |
ChQuaternion | GetRot () const |
Get the orientation of the chassis reference frame. More... | |
ChVector3d | GetDriverPos () const |
Get the global location of the driver. | |
double | GetSpeed () const |
Get the vehicle speed. More... | |
double | GetCOMSpeed () const |
Get the speed of the chassis COM. More... | |
double | GetRollRate () const |
Get the roll rate of the chassis. More... | |
double | GetPitchRate () const |
Get the pitch rate of the chassis. More... | |
double | GetYawRate () const |
Get the yaw rate of the chassis. More... | |
double | GetTurnRate () const |
Get the turn rate of the chassis. More... | |
ChVector3d | GetPointLocation (const ChVector3d &locpos) const |
Get the global position of the specified point. More... | |
ChVector3d | GetPointVelocity (const ChVector3d &locpos) const |
Get the global velocity of the specified point. More... | |
ChVector3d | GetPointAcceleration (const ChVector3d &locpos) const |
Get the acceleration at the specified point. More... | |
void | Initialize (ChVehicle *vehicle, const ChCoordsys<> &chassisPos, double chassisFwdVel, int collision_family=0) |
Initialize the chassis at the specified global position and orientation. More... | |
virtual void | EnableCollision (bool state)=0 |
Enable/disable contact for the chassis. More... | |
void | SetFixed (bool val) |
Set the "fixed to ground" status of the chassis body. | |
bool | IsFixed () const |
Return true if the chassis body is fixed to ground. | |
bool | HasBushings () const |
Return true if the vehicle model contains bushings. | |
void | AddMarker (const std::string &name, const ChFrame<> &frame) |
Add a marker on the chassis body at the specified position (relative to the chassis reference frame). More... | |
const std::vector< std::shared_ptr< ChMarker > > & | GetMarkers () const |
void | SetAerodynamicDrag (double Cd, double area, double air_density) |
Set parameters and enable aerodynamic drag force calculation. More... | |
virtual void | Synchronize (double time) |
Update the state of the chassis subsystem. More... | |
void | AddJoint (std::shared_ptr< ChJoint > joint) |
Utility function to add a joint (kinematic or bushing) to the vehicle system. | |
void | AddExternalForceTorque (std::shared_ptr< ExternalForceTorque > load) |
Utility force to add an external load to the chassis body. | |
void | AddTerrainLoad (std::shared_ptr< ChLoadBase > terrain_load) |
Add a terrain load to the vehicle system. More... | |
virtual void | InitializeInertiaProperties () override |
Initialize subsystem inertia properties. More... | |
virtual void | UpdateInertiaProperties () override |
Update subsystem inertia properties. More... | |
![]() | |
const std::string & | GetName () const |
Get the name identifier for this subsystem. | |
void | SetName (const std::string &name) |
Set the name identifier for this subsystem. | |
virtual std::string | GetTemplateName () const =0 |
Get the name of the vehicle subsystem template. | |
bool | IsInitialized () const |
Return flag indicating whether or not the part is fully constructed. | |
int | GetBodyTag () const |
Get the tag for component bodies. | |
double | GetMass () const |
Get the subsystem mass. More... | |
const ChFrame & | GetCOMFrame () const |
Get the current subsystem COM frame (relative to and expressed in the subsystem's reference frame). More... | |
const ChMatrix33 & | GetInertia () const |
Get the current subsystem inertia (relative to the subsystem COM frame). More... | |
const ChFrame & | GetTransform () const |
Get the current subsystem position relative to the global frame. More... | |
void | SetVisualizationType (VisualizationType vis) |
Set the visualization mode for this subsystem. | |
virtual void | AddVisualizationAssets (VisualizationType vis) |
Add visualization assets to this subsystem, for the specified visualization mode. | |
virtual void | RemoveVisualizationAssets () |
Remove all visualization assets from this subsystem. | |
virtual void | SetOutput (bool state) |
Enable/disable output for this subsystem. | |
bool | OutputEnabled () const |
Return the output state for this subsystem. | |
virtual void | ExportComponentList (rapidjson::Document &jsonDocument) const |
Export this subsystem's component list to the specified JSON object. More... | |
virtual void | Output (ChVehicleOutput &database) const |
Output data for this subsystem's component list to the specified database. | |
Static Public Member Functions | |
static void | RemoveJoint (std::shared_ptr< ChJoint > joint) |
Utility function to remove a joint (kinematic or bushing) from the vehicle system. | |
![]() | |
static ChMatrix33 | TransformInertiaMatrix (const ChVector3d &moments, const ChVector3d &products, const ChMatrix33<> &vehicle_rot, const ChMatrix33<> &body_rot) |
Utility function for transforming inertia tensors between centroidal frames. More... | |
Protected Member Functions | |
ChChassis (const std::string &name, bool fixed=false) | |
Construct a chassis subsystem with the specified name. More... | |
virtual void | Construct (ChVehicle *vehicle, const ChCoordsys<> &chassisPos, double chassisFwdVel, int collision_family)=0 |
Construct the concrete chassis at the specified global position and orientation. More... | |
virtual double | GetBodyMass () const =0 |
virtual ChFrame | GetBodyCOMFrame () const =0 |
virtual ChMatrix33 | GetBodyInertia () const =0 |
![]() | |
ChPart (const std::string &name) | |
Construct a vehicle subsystem with the specified name. | |
void | AddMass (double &mass) |
Add this subsystem's mass. More... | |
void | AddInertiaProperties (ChVector3d &com, ChMatrix33<> &inertia) |
Add this subsystem's inertia properties. More... | |
virtual void | Create (const rapidjson::Document &d) |
Create a vehicle subsystem from JSON data. More... | |
void | ExportBodyList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChBody >> bodies) const |
Export the list of bodies to the specified JSON document. | |
void | ExportShaftList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaft >> shafts) const |
Export the list of shafts to the specified JSON document. | |
void | ExportJointList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLink >> joints) const |
Export the list of joints to the specified JSON document. | |
void | ExportCouplesList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaftsCouple >> couples) const |
Export the list of shaft couples to the specified JSON document. | |
void | ExportMarkerList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChMarker >> markers) const |
Export the list of markers to the specified JSON document. | |
void | ExportLinSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkTSDA >> springs) const |
Export the list of translational springs to the specified JSON document. | |
void | ExportRotSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkRSDA >> springs) const |
Export the list of rotational springs to the specified JSON document. | |
void | ExportBodyLoadList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLoadBodyBody >> loads) const |
Export the list of body-body loads to the specified JSON document. | |
Protected Attributes | |
ChVehicle * | m_vehicle |
associated vehicle | |
std::shared_ptr< ChBodyAuxRef > | m_body |
handle to the chassis body | |
std::shared_ptr< ChLoadContainer > | m_container_bushings |
load container for vehicle bushings | |
std::shared_ptr< ChLoadContainer > | m_container_external |
load container for external forces | |
std::shared_ptr< ChLoadContainer > | m_container_terrain |
load container for terrain forces | |
std::vector< std::shared_ptr< ExternalForceTorque > > | m_external_loads |
external loads | |
std::vector< std::shared_ptr< ChMarker > > | m_markers |
list of user-defined markers | |
bool | m_fixed |
is the chassis body fixed to ground? | |
![]() | |
std::string | m_name |
subsystem name | |
bool | m_initialized |
specifies whether ot not the part is fully constructed | |
bool | m_output |
specifies whether or not output is generated for this subsystem | |
std::shared_ptr< ChPart > | m_parent |
parent subsystem (empty if parent is vehicle) | |
double | m_mass |
subsystem mass | |
ChMatrix33 | m_inertia |
inertia tensor (relative to subsystem COM) | |
ChFrame | m_com |
COM frame (relative to subsystem reference frame) | |
ChFrame | m_xform |
subsystem frame expressed in the global frame | |
int | m_obj_tag |
tag for part objects | |
Friends | |
class | ChChassisRear |
Additional Inherited Members | |
![]() | |
static void | RemoveVisualizationAssets (std::shared_ptr< ChPhysicsItem > item) |
Erase all visual shapes from the visual model associated with the specified physics item (if any). | |
static void | RemoveVisualizationAsset (std::shared_ptr< ChPhysicsItem > item, std::shared_ptr< ChVisualShape > shape) |
Erase the given shape from the visual model associated with the specified physics item (if any). | |
Constructor & Destructor Documentation
◆ ChChassis()
|
protected |
Construct a chassis subsystem with the specified name.
- Parameters
-
[in] name name of the subsystem [in] fixed is the chassis body fixed to ground?
Member Function Documentation
◆ AddMarker()
void chrono::vehicle::ChChassis::AddMarker | ( | const std::string & | name, |
const ChFrame<> & | frame | ||
) |
Add a marker on the chassis body at the specified position (relative to the chassis reference frame).
If called before initialization, this function has no effect.
- Parameters
-
[in] name marker name [in] frame marker position relative to chassis reference frame
◆ AddTerrainLoad()
void chrono::vehicle::ChChassis::AddTerrainLoad | ( | std::shared_ptr< ChLoadBase > | terrain_load | ) |
Add a terrain load to the vehicle system.
Terrain forces and torques (generated by the vehicle-terrain interface) are managed through a load container maintained on the vehicle chassis.
◆ Construct()
|
protectedpure virtual |
Construct the concrete chassis at the specified global position and orientation.
The initial position and forward velocity are assumed to be given in the current world frame.
- Parameters
-
[in] vehicle containing vehicle [in] chassisPos absolute chassis position [in] chassisFwdVel initial chassis forward velocity [in] collision_family chassis collision family
Implemented in chrono::vehicle::ChRigidChassis.
◆ EnableCollision()
|
pure virtual |
Enable/disable contact for the chassis.
This function controls contact of the chassis with all other collision shapes in the simulation. Must be called after initialization and has effect only if the derived object has defined some collision shapes.
Implemented in chrono::vehicle::ChRigidChassisRear, and chrono::vehicle::ChRigidChassis.
◆ GetCOMSpeed()
double chrono::vehicle::ChChassis::GetCOMSpeed | ( | ) | const |
Get the speed of the chassis COM.
Return the speed measured at the chassis center of mass.
◆ GetLocalDriverCoordsys()
|
pure virtual |
Get the local driver position and orientation.
This is a coordinate system relative to the chassis reference frame.
Implemented in chrono::vehicle::bmw::BMW_E90_Chassis, chrono::vehicle::jeep::Cherokee_Chassis, chrono::vehicle::unimog::U401_Chassis, chrono::vehicle::artcar::ARTcar_Chassis, chrono::vehicle::duro::Duro_Chassis, chrono::vehicle::gclass::G500_Chassis, chrono::vehicle::hmmwv::HMMWV_Chassis, chrono::vehicle::mrole::mrole_Chassis, chrono::vehicle::sedan::Sedan_Chassis, chrono::vehicle::uaz::UAZBUS_Chassis, chrono::vehicle::fmtv::FMTV_ChassisFront, chrono::vehicle::generic::Generic_Chassis, chrono::vehicle::kraz::Kraz_tractor_Chassis, chrono::vehicle::citybus::CityBus_Chassis, chrono::vehicle::feda::FEDA_Chassis, chrono::vehicle::gator::Gator_Chassis, chrono::vehicle::m113::M113_Chassis, chrono::vehicle::man::MAN_10t_Chassis, chrono::vehicle::man::MAN_5t_Chassis, chrono::vehicle::man::MAN_7t_Chassis, chrono::vehicle::marder::Marder_Chassis, and chrono::vehicle::RigidChassis.
◆ GetPitchRate()
double chrono::vehicle::ChChassis::GetPitchRate | ( | ) | const |
Get the pitch rate of the chassis.
The yaw rate is referenced to the chassis frame.
◆ GetPointAcceleration()
ChVector3d chrono::vehicle::ChChassis::GetPointAcceleration | ( | const ChVector3d & | locpos | ) | const |
Get the acceleration at the specified point.
The point is assumed to be given relative to the chassis reference frame. The returned acceleration is expressed in the chassis reference frame.
◆ GetPointLocation()
ChVector3d chrono::vehicle::ChChassis::GetPointLocation | ( | const ChVector3d & | locpos | ) | const |
Get the global position of the specified point.
The point is assumed to be given relative to the chassis reference frame. The returned location is expressed in the global reference frame.
◆ GetPointVelocity()
ChVector3d chrono::vehicle::ChChassis::GetPointVelocity | ( | const ChVector3d & | locpos | ) | const |
Get the global velocity of the specified point.
The point is assumed to be given relative to the chassis reference frame. The returned velocity is expressed in the global reference frame.
◆ GetRollRate()
double chrono::vehicle::ChChassis::GetRollRate | ( | ) | const |
Get the roll rate of the chassis.
The yaw rate is referenced to the chassis frame.
◆ GetRot()
ChQuaternion chrono::vehicle::ChChassis::GetRot | ( | ) | const |
Get the orientation of the chassis reference frame.
Returns a rotation with respect to the global reference frame.
◆ GetSpeed()
double chrono::vehicle::ChChassis::GetSpeed | ( | ) | const |
Get the vehicle speed.
Return the speed measured at the origin of the chassis reference frame.
◆ GetTurnRate()
double chrono::vehicle::ChChassis::GetTurnRate | ( | ) | const |
Get the turn rate of the chassis.
Unlike the yaw rate (referenced to the chassis frame), the turn rate is referenced to the global frame.
◆ GetVehicleTag()
|
overridevirtual |
Get the tag of the associated vehicle.
This method can only be called after initialization of the chassis subsystem.
Reimplemented from chrono::vehicle::ChPart.
◆ GetYawRate()
double chrono::vehicle::ChChassis::GetYawRate | ( | ) | const |
Get the yaw rate of the chassis.
The yaw rate is referenced to the chassis frame.
◆ Initialize()
void chrono::vehicle::ChChassis::Initialize | ( | ChVehicle * | vehicle, |
const ChCoordsys<> & | chassisPos, | ||
double | chassisFwdVel, | ||
int | collision_family = 0 |
||
) |
Initialize the chassis at the specified global position and orientation.
The initial position and forward velocity are assumed to be given in the current world frame.
- Parameters
-
[in] vehicle containing vehicle [in] chassisPos absolute chassis position [in] chassisFwdVel initial chassis forward velocity [in] collision_family chassis collision family
◆ InitializeInertiaProperties()
|
overridevirtual |
Initialize subsystem inertia properties.
Derived classes must override this function and set the subsystem mass (m_mass) and, if constant, the subsystem COM frame and its inertia tensor. This function is called during initialization of the vehicle system.
Implements chrono::vehicle::ChPart.
◆ SetAerodynamicDrag()
void chrono::vehicle::ChChassis::SetAerodynamicDrag | ( | double | Cd, |
double | area, | ||
double | air_density | ||
) |
Set parameters and enable aerodynamic drag force calculation.
By default, aerodynamic drag force calculation is disabled.
- Parameters
-
[in] Cd drag coefficient [in] area reference area [in] air_density air density
◆ Synchronize()
|
virtual |
Update the state of the chassis subsystem.
The base class implementation applies all defined external forces to the chassis body.
◆ UpdateInertiaProperties()
|
overridevirtual |
Update subsystem inertia properties.
Derived classes must override this function and set the global subsystem transform (m_xform) and, unless constant, the subsystem COM frame (m_com) and its inertia tensor (m_inertia). Calculate the current inertia properties and global frame of this subsystem. This function is called every time the state of the vehicle system is advanced in time.
Implements chrono::vehicle::ChPart.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_vehicle/ChChassis.h
- /builds/uwsbel/chrono/src/chrono_vehicle/ChChassis.cpp