chrono::vehicle::ChInteractiveDriverIRR Class Reference
Description
Irrlicht-based interactive driver for the a vehicle.
This class implements the functionality required by the base ChInteractiveDriver class using keyboard or joystick inputs. As an Irrlicht event receiver, its OnEvent() callback is used to keep track and update the current driver inputs.
#include <ChInteractiveDriverIRR.h>
Inheritance diagram for chrono::vehicle::ChInteractiveDriverIRR:
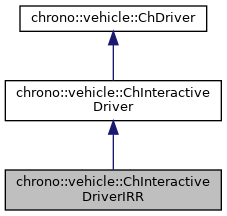
Collaboration diagram for chrono::vehicle::ChInteractiveDriverIRR:

Public Member Functions | |
ChInteractiveDriverIRR (ChVehicleVisualSystemIrrlicht &vsys) | |
Construct an Irrlicht GUI driver. | |
virtual bool | HasJoystick () const override |
Check if joystick is supported. | |
virtual void | Initialize () override |
Initialize this driver system. | |
void | SetJoystickConfigFile (const std::string &filename) |
Set joystick JSON configuration file name. | |
void | SetJoystickDebug (bool val) |
Enable/disable joystick debugging output (default: false). | |
void | SetButtonCallback (int button, void(*cbfun)()) |
Feed button number and callback function to implement a custom callback. | |
![]() | |
ChInteractiveDriver (ChVehicle &vehicle) | |
Construct an interactive driver. | |
virtual void | Synchronize (double time) override |
Update the state of this driver system at the specified time. | |
virtual void | Advance (double step) override |
Advance the state of this driver system by the specified time step. | |
void | SetInputMode (InputMode mode) |
Set the current functioning mode. | |
void | SetThrottleDelta (double delta) |
Set the increment in throttle input for each recorded keypress (default 1/50). | |
void | SetSteeringDelta (double delta) |
Set the increment in steering input for each recorded keypress (default 1/50). | |
void | SetBrakingDelta (double delta) |
Set the increment in braking input for each recorded keypress (default 1/50). | |
void | SetClutchDelta (double delta) |
Set the increment in clutch input for each recorded keypress (default 1/50). | |
void | SetStepsize (double val) |
Set the step size for integration of the internal driver dynamics. | |
void | SetGains (double steering_gain=1, double throttle_gain=1, double braking_gain=1, double clutch_gain=1) |
Set gains for internal dynamics. More... | |
void | SetInputDataFile (const std::string &filename) |
Set the input file for the underlying data driver. | |
![]() | |
ChDriver (ChVehicle &vehicle) | |
Construct a driver subsystem associated with the given vehicle. | |
double | GetThrottle () const |
Get the driver throttle input (in the range [0,1]). | |
double | GetSteering () const |
Get the driver steering input (in the range [-1,+1]). | |
double | GetBraking () const |
Get the driver braking input (in the range [0,1]). | |
double | GetClutch () const |
Get the driver clutch input (in the range [0,1]). | |
DriverInputs | GetInputs () const |
Get all current inputs at once. | |
bool | LogInit (const std::string &filename) |
Initialize output file for recording driver inputs. | |
bool | Log (double time) |
Record the current driver inputs to the log file. | |
void | SetSteering (double steering) |
Overwrite the value for the driver steering input (input is clamped in [-1,+1]). | |
void | SetThrottle (double throttle) |
Overwrite the value for the driver throttle input (input is clamped in [0,+1]). | |
void | SetBraking (double braking) |
Overwrite the value for the driver braking input (input is clamped in [0,+1]). | |
void | SetClutch (double clutch) |
Overwrite the value for the clutch braking input (input is clamped in [0,+1]). | |
Additional Inherited Members | |
![]() | |
enum | InputMode { InputMode::LOCK, InputMode::KEYBOARD, InputMode::DATAFILE, InputMode::JOYSTICK } |
Functioning modes for a ChInteractiveDriver. More... | |
![]() | |
InputMode | m_mode |
current mode of the driver | |
double | m_steering_target |
current target value for steering input | |
double | m_throttle_target |
current target value for throttle input | |
double | m_braking_target |
current target value for braking input | |
double | m_clutch_target |
current target value for clutch input | |
double | m_stepsize |
time step for internal dynamics | |
double | m_steering_delta |
steering increment on each keypress | |
double | m_throttle_delta |
throttle increment on each keypress | |
double | m_braking_delta |
braking increment on each keypress | |
double | m_clutch_delta |
clutch increment on each keypress | |
double | m_steering_gain |
gain for steering internal dynamics | |
double | m_throttle_gain |
gain for throttle internal dynamics | |
double | m_braking_gain |
gain for braking internal dynamics | |
double | m_clutch_gain |
gain for clutch internal dynamics | |
double | m_time_shift |
time at which mode was switched to DATAFILE | |
std::shared_ptr< ChDataDriver > | m_data_driver |
embedded data driver (for playback) | |
![]() | |
ChVehicle & | m_vehicle |
reference to associated vehicle | |
double | m_throttle |
current value of throttle input | |
double | m_steering |
current value of steering input | |
double | m_braking |
current value of braking input | |
double | m_clutch |
current value of clutch input | |
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_vehicle/driver/ChInteractiveDriverIRR.h
- /builds/uwsbel/chrono/src/chrono_vehicle/driver/ChInteractiveDriverIRR.cpp