Description
template<class Ta, class Tb>
class chrono::ChConstraintTwoTuplesContactN< Ta, Tb >
Normal reaction between two objects, each represented by a tuple of ChVariables objects.
Used ONLY when also two ChConstraintTwoTuplesFrictionT objects are used to represent friction. If these two tangent constraint are not used, for frictionless case, use a simple ChConstraintTwo with the ChConstraint::Mode::UNILATERAL mode.
Differently from an unilateral constraint, this does not enforce projection on positive constraint, since it will be up to the 'companion' ChConstraintTwoTuplesFrictionT objects to call a projection on the cone, by modifying all the three components (normal, u, v) at once.
Templates Ta and Tb are of ChVariableTupleCarrier_Nvars classes
#include <ChConstraintTwoTuplesContactN.h>
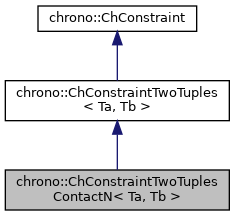
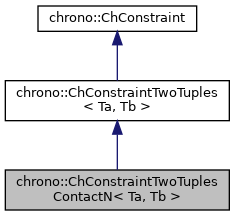
Public Member Functions | |
ChConstraintTwoTuplesContactN (const ChConstraintTwoTuplesContactN &other) | |
virtual ChConstraintTwoTuplesContactN * | Clone () const override |
"Virtual" copy constructor (covariant return type). | |
ChConstraintTwoTuplesContactN & | operator= (const ChConstraintTwoTuplesContactN &other) |
Assignment operator: copy from other object. | |
ChConstraintTwoTuplesFrictionT< Ta, Tb > * | GetTangentialConstraintU () const |
Get pointer to U tangential component. | |
ChConstraintTwoTuplesFrictionT< Ta, Tb > * | GetTangentialConstraintV () const |
Get pointer to V tangential component. | |
void | SetTangentialConstraintU (ChConstraintTwoTuplesFrictionT< Ta, Tb > *mconstr) |
Set pointer to U tangential component. | |
void | SetTangentialConstraintV (ChConstraintTwoTuplesFrictionT< Ta, Tb > *mconstr) |
Set pointer to V tangential component. | |
virtual void | Project () override |
Project the value of a possible 'l_i' value of constraint reaction onto admissible set. More... | |
![]() | |
ChConstraintTwoTuples (const ChConstraintTwoTuples &other) | |
ChConstraintTwoTuples & | operator= (const ChConstraintTwoTuples &other) |
Assignment operator: copy from other object. | |
type_constraint_tuple_a & | Get_tuple_a () |
Access tuple a. | |
type_constraint_tuple_b & | Get_tuple_b () |
Access tuple b. | |
virtual void | Update_auxiliary () override |
This function updates the following auxiliary data: More... | |
virtual double | ComputeJacobianTimesState () override |
Compute the product between the Jacobian of this constraint, [Cq_i], and the vector of variables. More... | |
virtual void | IncrementState (double deltal) override |
Increment the vector of variables with the quantity [invM]*[Cq_i]'*deltal. More... | |
virtual void | AddJacobianTimesVectorInto (double &result, ChVectorConstRef vect) const override |
Add the product of the corresponding block in the system matrix by 'vect' and add to result. More... | |
virtual void | AddJacobianTransposedTimesScalarInto (ChVectorRef result, double l) const override |
Add the product of the corresponding transposed block in the system matrix by 'l' and add to result. More... | |
virtual void | PasteJacobianInto (ChSparseMatrix &mat, unsigned int start_row, unsigned int start_col) const override |
Write the constraint Jacobian into the specified global matrix at the offsets of the associated variables. More... | |
virtual void | PasteJacobianTransposedInto (ChSparseMatrix &mat, unsigned int start_row, unsigned int start_col) const override |
Write the transposed constraint Jacobian into the specified global matrix at the offsets of the associated variables. More... | |
![]() | |
ChConstraint (const ChConstraint &other) | |
ChConstraint & | operator= (const ChConstraint &other) |
Assignment operator: copy from other object. | |
bool | operator== (const ChConstraint &other) const |
Comparison (compares only flags, not the Jacobians). | |
bool | IsValid () const |
Indicate if the constraint data is currently valid. | |
void | SetValid (bool mon) |
Set the "valid" state of this constraint. | |
bool | IsDisabled () const |
Indicate if the constraint is currently turned on or off. | |
void | SetDisabled (bool mon) |
bool | IsRedundant () const |
Indicate if the constraint is redundant or singular. | |
void | SetRedundant (bool mon) |
Mark the constraint as redundant. | |
bool | IsBroken () const |
Indicate if the constraint is broken, due to excess pulling/pushing. | |
void | SetBroken (bool mon) |
Set the constraint as broken. More... | |
virtual bool | IsUnilateral () const |
Indicate if the constraint is unilateral (typical complementarity constraint). | |
virtual bool | IsLinear () const |
Indicate if the constraint is linear. | |
Mode | GetMode () const |
Get the mode of the constraint. More... | |
void | SetMode (Mode mmode) |
Set the mode of the constraint. | |
bool | IsActive () const |
Indicate whether the constraint is currently active. More... | |
void | SetActive (bool isactive) |
Set the status of the constraint to active. | |
virtual double | ComputeResidual () |
Compute the residual of the constraint using the linear expression. More... | |
double | GetResidual () const |
Return the residual of this constraint. | |
void | SetRightHandSide (const double mb) |
Sets the known term b_i in [Cq_i]*q + b_i = 0, where: c_i = [Cq_i]*q + b_i = 0. | |
double | GetRightHandSide () const |
Return the known term b_i in [Cq_i]*q + b_i = 0, where: c_i= [Cq_i]*q + b_i = 0. | |
void | SetComplianceTerm (const double mcfm) |
Set the constraint force mixing term (default=0). More... | |
double | GetComplianceTerm () const |
Return the constraint force mixing term. | |
void | SetLagrangeMultiplier (double ml_i) |
Set the value of the corresponding Lagrange multiplier (constraint reaction). | |
double | GetLagrangeMultiplier () const |
Return the corresponding Lagrange multiplier (constraint reaction). | |
double | GetSchurComplement () const |
Return the 'g_i' product, that is [Cq_i]*[invM_i]*[Cq_i]' (+cfm) | |
void | SetSchurComplement (double m_g_i) |
Usually you should not use the SetSchurComplement function, because g_i should be automatically computed during the Update_auxiliary() . | |
virtual double | Violation (double mc_i) |
Return the constraint violation. More... | |
void | SetOffset (unsigned int off) |
Set offset in global q vector (set automatically by ChSystemDescriptor) | |
unsigned int | GetOffset () const |
Get offset in global q vector. | |
virtual void | ArchiveOut (ChArchiveOut &archive_out) |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIn (ChArchiveIn &archive_in) |
Method to allow de-serialization of transient data from archives. | |
Protected Attributes | |
ChConstraintTwoTuplesFrictionT< Ta, Tb > * | constraint_U |
U tangential component. | |
ChConstraintTwoTuplesFrictionT< Ta, Tb > * | constraint_V |
V tangential component. | |
![]() | |
type_constraint_tuple_a | tuple_a |
type_constraint_tuple_b | tuple_b |
![]() | |
double | c_i |
constraint residual (if satisfied, c must be 0) | |
double | l_i |
Lagrange multiplier (reaction) | |
double | b_i |
right-hand side term in [Cq_i]*q+b_i=0 , note: c_i= [Cq_i]*q + b_i | |
double | cfm_i |
Constraint force mixing if needed to add some numerical 'compliance' in the constraint. More... | |
Mode | mode |
mode of the constraint | |
double | g_i |
product [Cq_i]*[invM_i]*[Cq_i]' (+cfm) | |
unsigned int | offset |
offset in global "l" state vector (needed by some solvers) | |
Additional Inherited Members | |
![]() | |
enum | Mode { Mode::FREE, Mode::LOCK, Mode::UNILATERAL, Mode::FRICTION } |
Constraint mode. More... | |
Member Function Documentation
◆ Project()
|
inlineoverridevirtual |
Project the value of a possible 'l_i' value of constraint reaction onto admissible set.
This will also modify the l_i values of the two tangential friction constraints (projection onto the friction cone, as by Anitescu-Tasora theory).
Reimplemented from chrono::ChConstraint.
The documentation for this class was generated from the following file:
- /builds/uwsbel/chrono/src/chrono/solver/ChConstraintTwoTuplesContactN.h