Description
Forces are objects which must be attached to rigid bodies in order to apply torque or force to such body.
ChForce objects are able to represent either forces and torques, depending on a flag.
#include <ChForce.h>
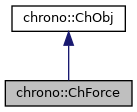
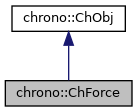
Public Types | |
enum | ForceType { FORCE, TORQUE } |
Types of force application. | |
enum | ReferenceFrame { BODY, WORLD } |
Reference for position frame. | |
enum | AlignmentFrame { BODY_DIR, WORLD_DIR } |
Reference for alignment. | |
Public Member Functions | |
ChForce (const ChForce &other) | |
virtual ChForce * | Clone () const override |
"Virtual" copy constructor (covariant return type). | |
ChBody * | GetBody () |
Return the parent body (the force belongs to this rigid body) | |
void | SetBody (ChBody *newRB) |
Sets the parent body (the force belongs to this rigid body) | |
void | SetMode (ForceType m_mode) |
Sets the mode (force or torque) | |
ForceType | GetMode () const |
void | SetAlign (AlignmentFrame m_align) |
Sets the alignment method. More... | |
AlignmentFrame | GetAlign () const |
void | SetFrame (ReferenceFrame m_frame) |
Sets the alignment method. More... | |
ReferenceFrame | GetFrame () const |
ChVector3d | GetVpoint () const |
Gets the application point, in absolute coordinates. | |
ChVector3d | GetVrelpoint () const |
Gets the application point, in rigid body coordinates. | |
void | SetVpoint (ChVector3d mypoint) |
Sets the application point, in absolute coordinates. | |
void | SetVrelpoint (ChVector3d myrelpoint) |
Sets the application point, in rigid body coordinates. | |
ChVector3d | GetDir () const |
Gets the force (or torque) direction, in absolute coordinates. | |
ChVector3d | GetRelDir () const |
Gets the force (or torque) direction, in rigid body coordinates. | |
void | SetDir (ChVector3d newf) |
Sets the force (or torque) direction, in absolute coordinates. | |
void | SetRelDir (ChVector3d newf) |
Sets the force (or torque) direction, in rigid body coordinates. | |
void | SetMforce (double newf) |
Sets force (or torque) modulus. | |
double | GetMforce () const |
Gets force (or torque) modulus. | |
void | SetModulation (std::shared_ptr< ChFunction > m_funct) |
Sets a function for time-modulation of the force. | |
std::shared_ptr< ChFunction > | GetModulation () const |
void | SetMove_x (std::shared_ptr< ChFunction > m_funct) |
Sets a function for time dependency of position (on x axis) | |
std::shared_ptr< ChFunction > | GetMove_x () const |
void | SetMove_y (std::shared_ptr< ChFunction > m_funct) |
Sets a function for time dependency of position (on y axis) | |
std::shared_ptr< ChFunction > | GetMove_y () const |
void | SetMove_z (std::shared_ptr< ChFunction > m_funct) |
Sets a function for time dependency of position (on z axis) | |
std::shared_ptr< ChFunction > | GetMove_z () const |
void | SetF_x (std::shared_ptr< ChFunction > m_funct) |
Sets a function for time dependency of force X component. | |
std::shared_ptr< ChFunction > | GetF_x () const |
void | SetF_y (std::shared_ptr< ChFunction > m_funct) |
Sets a function for time dependency of force Y component. | |
std::shared_ptr< ChFunction > | GetF_y () const |
void | SetF_z (std::shared_ptr< ChFunction > m_funct) |
Sets a function for time dependency of force Z component. | |
std::shared_ptr< ChFunction > | GetF_z () const |
ChVector3d | GetForce () const |
Gets the instant force vector -or torque vector- in absolute coordinates. | |
ChVector3d | GetRelForce () const |
Gets the instant force vector -or torque vector- in rigid body coordinates. | |
double | GetForceMod () const |
Gets the instant force vector -or torque vector- modulus. | |
const ChVectorN< double, 7 > & | GetQf () const |
Gets force-torque applied to rigid body, as lagrangian generalized force (7x1 matrix). | |
void | GetBodyForceTorque (ChVector3d &body_force, ChVector3d &body_torque) const |
Gets force-torque applied to rigid body, as force vector (in absol.coords) and torque vector (in body coords). | |
void | UpdateState () |
virtual void | Update (double time, bool update_assets) override |
Perform any updates necessary at the current phase during the solution process. More... | |
virtual void | ArchiveOut (ChArchiveOut &archive_out) override |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIn (ChArchiveIn &archive_in) override |
Method to allow deserialization of transient data from archives. | |
![]() | |
ChObj (const ChObj &other) | |
int | GetIdentifier () const |
Get the unique integer identifier of this object. More... | |
void | SetTag (int tag) |
Set an object integer tag (default: -1). More... | |
int | GetTag () const |
Get the tag of this object. | |
void | SetName (const std::string &myname) |
Set the name of this object. | |
const std::string & | GetName () const |
Get the name of this object. | |
double | GetChTime () const |
Gets the simulation time of this object. | |
void | SetChTime (double m_time) |
Sets the simulation time of this object. | |
void | AddVisualModel (std::shared_ptr< ChVisualModel > model) |
Add an (optional) visualization model. More... | |
std::shared_ptr< ChVisualModel > | GetVisualModel () const |
Access the visualization model (if any). More... | |
void | AddVisualShape (std::shared_ptr< ChVisualShape > shape, const ChFrame<> &frame=ChFrame<>()) |
Add the specified visual shape to the visualization model. More... | |
std::shared_ptr< ChVisualShape > | GetVisualShape (unsigned int i) const |
Access the specified visualization shape in the visualization model (if any). More... | |
void | AddVisualShapeFEA (std::shared_ptr< ChVisualShapeFEA > shapeFEA) |
Add the specified FEA visualization object to the visualization model. More... | |
std::shared_ptr< ChVisualShapeFEA > | GetVisualShapeFEA (unsigned int i) const |
Access the specified FEA visualization object in the visualization model (if any). More... | |
virtual ChFrame | GetVisualModelFrame (unsigned int nclone=0) const |
Get the reference frame (expressed in and relative to the absolute frame) of the visual model. More... | |
virtual unsigned int | GetNumVisualModelClones () const |
Return the number of clones of the visual model associated with this object. More... | |
void | AddCamera (std::shared_ptr< ChCamera > camera) |
Attach a camera to this object. More... | |
std::vector< std::shared_ptr< ChCamera > > | GetCameras () const |
Get the set of cameras attached to this object. | |
void | UpdateVisualModel () |
Utility function to update only the associated visual assets (if any). | |
virtual std::string & | ArchiveContainerName () |
Additional Inherited Members | |
![]() | |
int | GenerateUniqueIdentifier () |
![]() | |
double | ChTime |
object simulation time | |
std::string | m_name |
object name | |
int | m_identifier |
object unique identifier | |
int | m_tag |
user-supplied tag | |
std::shared_ptr< ChVisualModelInstance > | vis_model_instance |
instantiated visualization model | |
std::vector< std::shared_ptr< ChCamera > > | cameras |
set of cameras | |
Member Function Documentation
◆ SetAlign()
|
inline |
Sets the alignment method.
The force will rotate together with this reference.
◆ SetFrame()
|
inline |
Sets the alignment method.
The force application point will follow this reference.
◆ Update()
|
overridevirtual |
Perform any updates necessary at the current phase during the solution process.
This function is called at least once per step to update auxiliary data, internal states, etc. The default implementation updates the item's time stamp and its visualization assets (if any are defined anf only if requested).
Reimplemented from chrono::ChObj.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/physics/ChForce.h
- /builds/uwsbel/chrono/src/chrono/physics/ChForce.cpp