Description
Base class for all Chrono objects.
Each object receives a unique identifier and can be named and/or tagged.
#include <ChObject.h>
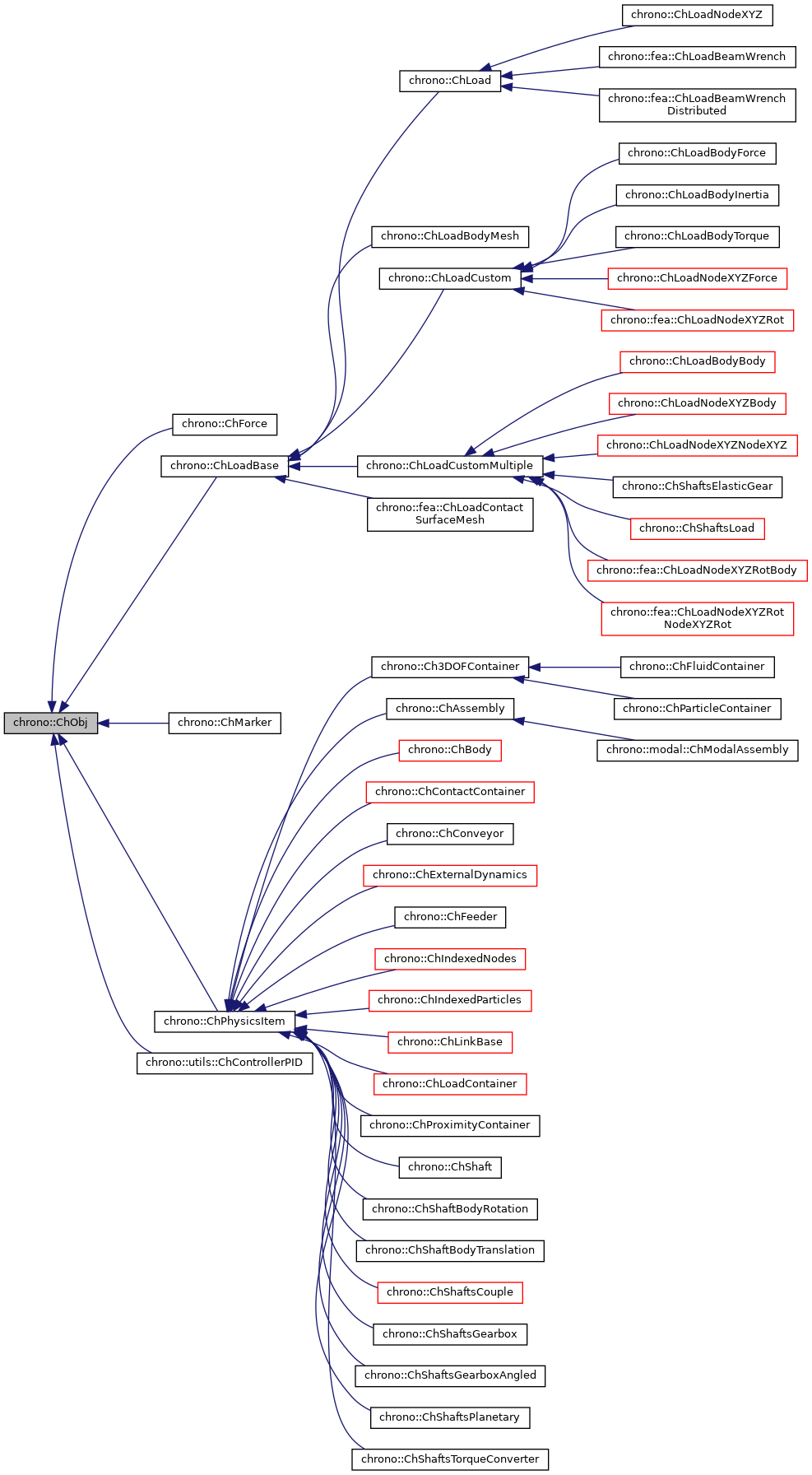
Public Member Functions | |
ChObj (const ChObj &other) | |
virtual ChObj * | Clone () const =0 |
"Virtual" copy constructor. | |
int | GetIdentifier () const |
Get the unique integer identifier of this object. More... | |
void | SetTag (int tag) |
Set an object integer tag (default: -1). More... | |
int | GetTag () const |
Get the tag of this object. | |
void | SetName (const std::string &myname) |
Set the name of this object. | |
const std::string & | GetName () const |
Get the name of this object. | |
double | GetChTime () const |
Gets the simulation time of this object. | |
void | SetChTime (double m_time) |
Sets the simulation time of this object. | |
void | AddVisualModel (std::shared_ptr< ChVisualModel > model) |
Add an (optional) visualization model. More... | |
std::shared_ptr< ChVisualModel > | GetVisualModel () const |
Access the visualization model (if any). More... | |
void | AddVisualShape (std::shared_ptr< ChVisualShape > shape, const ChFrame<> &frame=ChFrame<>()) |
Add the specified visual shape to the visualization model. More... | |
std::shared_ptr< ChVisualShape > | GetVisualShape (unsigned int i) const |
Access the specified visualization shape in the visualization model (if any). More... | |
void | AddVisualShapeFEA (std::shared_ptr< ChVisualShapeFEA > shapeFEA) |
Add the specified FEA visualization object to the visualization model. More... | |
std::shared_ptr< ChVisualShapeFEA > | GetVisualShapeFEA (unsigned int i) const |
Access the specified FEA visualization object in the visualization model (if any). More... | |
virtual ChFrame | GetVisualModelFrame (unsigned int nclone=0) const |
Get the reference frame (expressed in and relative to the absolute frame) of the visual model. More... | |
virtual unsigned int | GetNumVisualModelClones () const |
Return the number of clones of the visual model associated with this object. More... | |
void | AddCamera (std::shared_ptr< ChCamera > camera) |
Attach a camera to this object. More... | |
std::vector< std::shared_ptr< ChCamera > > | GetCameras () const |
Get the set of cameras attached to this object. | |
virtual void | Update (double time, bool update_assets) |
Perform any updates necessary at the current phase during the solution process. More... | |
void | UpdateVisualModel () |
Utility function to update only the associated visual assets (if any). | |
virtual void | ArchiveOut (ChArchiveOut &archive_out) |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIn (ChArchiveIn &archive_in) |
Method to allow de-serialization of transient data from archives. | |
virtual std::string & | ArchiveContainerName () |
Protected Member Functions | |
int | GenerateUniqueIdentifier () |
Protected Attributes | |
double | ChTime |
object simulation time | |
std::string | m_name |
object name | |
int | m_identifier |
object unique identifier | |
int | m_tag |
user-supplied tag | |
std::shared_ptr< ChVisualModelInstance > | vis_model_instance |
instantiated visualization model | |
std::vector< std::shared_ptr< ChCamera > > | cameras |
set of cameras | |
Member Function Documentation
◆ AddCamera()
void chrono::ChObj::AddCamera | ( | std::shared_ptr< ChCamera > | camera | ) |
Attach a camera to this object.
Multiple cameras can be attached to the same object.
◆ AddVisualModel()
void chrono::ChObj::AddVisualModel | ( | std::shared_ptr< ChVisualModel > | model | ) |
Add an (optional) visualization model.
Not that an instance of the given visual model is associated with this object, thus allowing sharing the same model among multiple objects.
◆ AddVisualShape()
void chrono::ChObj::AddVisualShape | ( | std::shared_ptr< ChVisualShape > | shape, |
const ChFrame<> & | frame = ChFrame<>() |
||
) |
Add the specified visual shape to the visualization model.
If this object does not have a visual model, one is created.
◆ AddVisualShapeFEA()
void chrono::ChObj::AddVisualShapeFEA | ( | std::shared_ptr< ChVisualShapeFEA > | shapeFEA | ) |
Add the specified FEA visualization object to the visualization model.
If this object does not have a visual model, one is created.
◆ GetIdentifier()
|
inline |
Get the unique integer identifier of this object.
Object identifiers are generated automatically in incremental order based on the order in which objects are created. These identifiers are transient and as such are not serialized. However, user code can cache the identifier of any Chrono object and use it later (e.g., to search the item in a ChAssembly).
◆ GetNumVisualModelClones()
|
inlinevirtual |
Return the number of clones of the visual model associated with this object.
If the visual model is cloned (for example for an object modeling a particle system), this function should return the total number of copies of the visual model, including the "original". The current coordinate frame of a given clone can be obtained by calling GetVisualModelFrame() with the corresponding clone identifier.
Reimplemented in chrono::ChIndexedParticles.
◆ GetVisualModel()
std::shared_ptr< ChVisualModel > chrono::ChObj::GetVisualModel | ( | ) | const |
Access the visualization model (if any).
Note that this model may be shared with other objects that may instance it. Returns nullptr if no visual model is present.
◆ GetVisualModelFrame()
|
inlinevirtual |
Get the reference frame (expressed in and relative to the absolute frame) of the visual model.
If the visual model is cloned (for example for an object modeling a particle system), this function returns the coordinate system of the specified clone.
Reimplemented in chrono::ChBody, chrono::ChLinkRSDA, chrono::ChLinkMarkers, chrono::ChIndexedParticles, chrono::ChLinkBase, and chrono::ChLinkMateGeneric.
◆ GetVisualShape()
std::shared_ptr< ChVisualShape > chrono::ChObj::GetVisualShape | ( | unsigned int | i | ) | const |
Access the specified visualization shape in the visualization model (if any).
Note that no range check is performed.
◆ GetVisualShapeFEA()
std::shared_ptr< ChVisualShapeFEA > chrono::ChObj::GetVisualShapeFEA | ( | unsigned int | i | ) | const |
Access the specified FEA visualization object in the visualization model (if any).
Note that no range check is performed.
◆ SetTag()
|
inline |
Set an object integer tag (default: -1).
Unlike the object identifier, this tag is completely under user control and not used anywhere else in Chrono. Tags are serialized and de-serialized.
◆ Update()
|
virtual |
Perform any updates necessary at the current phase during the solution process.
This function is called at least once per step to update auxiliary data, internal states, etc. The default implementation updates the item's time stamp and its visualization assets (if any are defined anf only if requested).
Reimplemented in chrono::ChLinkMateRackPinion, chrono::modal::ChModalAssembly, chrono::ChParticleCloud, chrono::ChBody, chrono::fea::ChLinkNodeFaceRot, chrono::ChLinkMatePlanar, chrono::fea::ChLinkNodeFrameGeneric, chrono::ChAssembly, chrono::ChExternalDynamicsDAE, chrono::ChLoadNodeXYZNodeXYZ, chrono::ChLinkMateGeneric, chrono::ChMarker, chrono::ChLinkLock, chrono::ChShaft, chrono::ChLoadNodeXYZForceAbs, chrono::ChForce, chrono::ChHydraulicActuatorBase, chrono::ChLinkMarkers, chrono::fea::ChLinkNodeFace, chrono::ChLinkRevoluteTranslational, chrono::fea::ChLoadNodeXYZRotForceAbs, chrono::ChLinkRevoluteSpherical, chrono::ChLoadNodeXYZForce, chrono::fea::ChLinkBeamIGAFrame, chrono::fea::ChLinkNodeFrame, chrono::ChLoadBase, chrono::ChShaftsTorqueConverter, chrono::fea::ChLinkNodeSlopeFrame, chrono::ChExternalDynamicsODE, chrono::ChLinkMotorRotation, chrono::fea::ChLinkNodeNode, chrono::ChLinkMotorLinear, chrono::ChLink, chrono::fea::ChLoadNodeXYZRot, chrono::ChBodyAuxRef, chrono::ChShaftsTorque, chrono::ChLinkMotor, chrono::ChLoadContainer, chrono::ChPhysicsItem, chrono::ChLinkMateOrthogonal, chrono::ChLinkMateDistanceZ, chrono::ChContactContainerSMC, chrono::ChContactContainerNSC, and chrono::fea::ChMesh.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/physics/ChObject.h
- /builds/uwsbel/chrono/src/chrono/physics/ChObject.cpp