Description
Geometric object representing a NURBS surface.
#include <ChSurfaceNurbs.h>
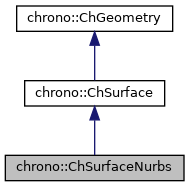
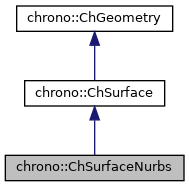
Public Member Functions | |
ChSurfaceNurbs () | |
Constructor. By default, a segment (order = 1, two points on X axis, at -1, +1) | |
ChSurfaceNurbs (int morder_u, int morder_v, ChMatrixDynamic< ChVector3d > &mpoints, ChVectorDynamic<> *mknots_u=0, ChVectorDynamic<> *mknots_v=0, ChMatrixDynamic<> *new_weights=0) | |
Constructor from a given array of control points. More... | |
ChSurfaceNurbs (const ChSurfaceNurbs &source) | |
virtual ChSurfaceNurbs * | Clone () const override |
"Virtual" copy constructor (covariant return type). | |
virtual ChVector3d | Evaluate (double parU, double parV) const override |
Return a point on the surface, given parametric coordinates U,V. More... | |
double | ComputeUfromKnotU (double u) const |
When using Evaluate() etc. More... | |
double | ComputeKnotUfromU (double U) const |
When using Evaluate() etc. More... | |
double | ComputeVfromKnotV (double v) const |
When using Evaluate() etc. More... | |
double | ComputeKnotVfromV (double V) const |
When using Evaluate() etc. More... | |
ChMatrixDynamic< ChVector3d > & | Points () |
Access the points. | |
ChMatrixDynamic & | Weights () |
Access the weights. | |
ChVectorDynamic & | Knots_u () |
Access the U knots. | |
ChVectorDynamic & | Knots_v () |
Access the U knots. | |
int | GetOrder_u () |
Get the order in u direction. | |
int | GetOrder_v () |
Get the order in v direction. | |
virtual void | Setup (int morder_u, int morder_v, ChMatrixDynamic< ChVector3d > &mpoints, ChVectorDynamic<> *mknots_u=0, ChVectorDynamic<> *mknots_v=0, ChMatrixDynamic<> *weights=0) |
Initial easy setup from a given array of control points. More... | |
virtual void | ArchiveOut (ChArchiveOut &archive_out) override |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIn (ChArchiveIn &archive_in) override |
Method to allow de-serialization of transient data from archives. | |
![]() | |
ChSurface (const ChSurface &other) | |
virtual ChVector3d | GetNormal (double parU, double parV) const |
Return the normal unit vector at the parametric coordinates (U,V). More... | |
virtual bool | IsClosedU () const |
Return true if the surface is closed (periodic) in the 1st parametric coordinate. | |
virtual bool | IslosedV () const |
Return true if the surface is closed (periodic) in the 2nd parametric coordinate. | |
virtual int | GetManifoldDimension () const override final |
Return dimensionality of this object. More... | |
bool | IsWireframe () |
Return true if visualization is done only as UV isolines. | |
void | SetWireframe (bool mw) |
Enable/disable visualization as wireframe. | |
![]() | |
ChGeometry (const ChGeometry &other) | |
virtual Type | GetType () const |
Get the class type as an enum. | |
virtual ChAABB | GetBoundingBox () const |
Compute bounding box along the directions of the shape definition frame. More... | |
void | InflateBoundingBox (ChAABB &bbox) const |
Enlarge the given existing bounding box with the bounding box of this object. | |
virtual double | GetBoundingSphereRadius () const |
Returns the radius of a bounding sphere for this geometry. More... | |
virtual ChVector3d | Baricenter () const |
Compute center of mass. | |
virtual void | Update () |
Generic update of internal data. | |
Public Attributes | |
ChMatrixDynamic< ChVector3d > | points |
ChMatrixDynamic | weights |
ChVectorDynamic | knots_u |
ChVectorDynamic | knots_v |
int | p_u |
int | p_v |
Additional Inherited Members | |
![]() | |
enum | Type { NONE, SPHERE, ELLIPSOID, BOX, CYLINDER, TRIANGLE, CAPSULE, CONE, LINE, LINE_ARC, LINE_BEZIER, LINE_CAM, LINE_PATH, LINE_POLY, LINE_SEGMENT, ROUNDED_BOX, ROUNDED_CYLINDER, TRIANGLEMESH, TRIANGLEMESH_CONNECTED, TRIANGLEMESH_SOUP } |
Enumeration of geometric object types. | |
![]() | |
bool | wireframe |
Constructor & Destructor Documentation
◆ ChSurfaceNurbs()
chrono::ChSurfaceNurbs::ChSurfaceNurbs | ( | int | morder_u, |
int | morder_v, | ||
ChMatrixDynamic< ChVector3d > & | mpoints, | ||
ChVectorDynamic<> * | mknots_u = 0 , |
||
ChVectorDynamic<> * | mknots_v = 0 , |
||
ChMatrixDynamic<> * | new_weights = 0 |
||
) |
Constructor from a given array of control points.
Input data is copied. If the knots are not provided, a uniformly spaced knot vector is made. If the weights are not provided, a constant weight vector is made.
- Parameters
-
morder_u order pu: 1= linear, 2=quadratic, etc. morder_v order pv: 1= linear, 2=quadratic, etc. mpoints control points, size nuxnv. Required: nu at least pu+1, same for v mknots_u knots, size ku. Required ku=nu+pu+1. If not provided, initialized to uniform. mknots_v knots, size kv. Required ku=nu+pu+1. If not provided, initialized to uniform. new_weights weights, size nuxnv. If not provided, all weights as 1.
Member Function Documentation
◆ ComputeKnotUfromU()
|
inline |
When using Evaluate() etc.
you need U parameter to be in 0..1 range, but knot range is not necessarily in 0..1. So you can convert U->u, where u is in knot range, calling this:
◆ ComputeKnotVfromV()
|
inline |
When using Evaluate() etc.
you need V parameter to be in 0..1 range, but knot range is not necessarily in 0..1. So you can convert V->v, where v is in knot range, calling this:
◆ ComputeUfromKnotU()
|
inline |
When using Evaluate() etc.
you need U parameter to be in 0..1 range, but knot range is not necessarily in 0..1. So you can convert u->U, where u is in knot range, calling this:
◆ ComputeVfromKnotV()
|
inline |
When using Evaluate() etc.
you need V parameter to be in 0..1 range, but knot range is not necessarily in 0..1. So you can convert v->V, where v is in knot range, calling this:
◆ Evaluate()
|
overridevirtual |
Return a point on the surface, given parametric coordinates U,V.
Parameters U and V always work in 0..1 range. As such, to use u' in knot range, use ComputeUfromKnotU().
Reimplemented from chrono::ChSurface.
◆ Setup()
|
virtual |
Initial easy setup from a given array of control points.
Input data is copied. If the knots are not provided, a uniformly spaced knot vector is made. If the weights are not provided, a constant weight vector is made.
- Parameters
-
morder_u order pu: 1= linear, 2=quadratic, etc. morder_v order pv: 1= linear, 2=quadratic, etc. mpoints control points, size nuxnv. Required: at least nu >= pu+1, same for v mknots_u knots u, size ku. Required ku=nu+pu+1. If not provided, initialized to uniform. mknots_v knots v, size kv. Required ku=nu+pu+1. If not provided, initialized to uniform. weights weights, size nuxnv. If not provided, all weights as 1.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/geometry/ChSurfaceNurbs.h
- /builds/uwsbel/chrono/src/chrono/geometry/ChSurfaceNurbs.cpp