Description
Class for post processing implementation that generates scripts for Blender.
The script can be used in Blender to render photo-realistic animations, if the chrono_import.py add-on is installed in Blender.
#include <ChBlender.h>
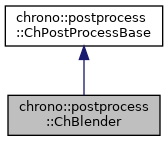
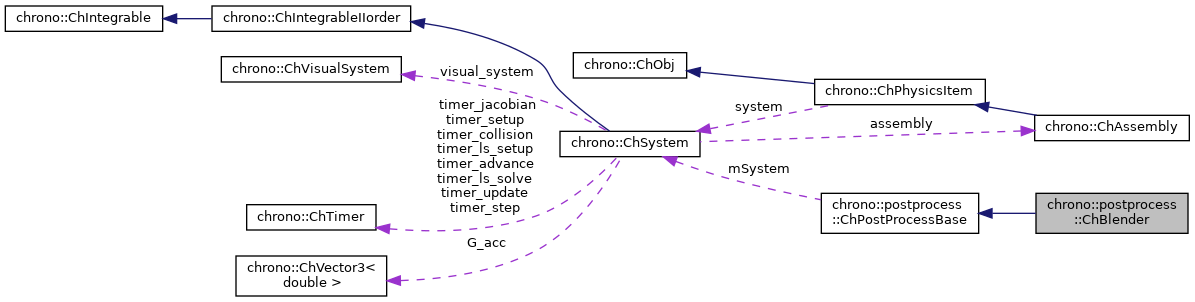
Public Types | |
enum | ContactSymbolType { NONE = 0, VECTOR, SPHERE } |
Modes for type of vector arrow length. | |
enum | ContactSymbolVectorLength { CONSTANT = 0, PROPERTY } |
Modes for type of vector arrow length. | |
enum | ContactSymbolVectorWidth { CONSTANT = 0, PROPERTY } |
Modes for type of vector arrow width. | |
enum | ContactSymbolSphereSize { CONSTANT = 0, PROPERTY } |
Modes for type of dot size. | |
enum | ContactSymbolColor { CONSTANT = 0, PROPERTY } |
Modes for colorizing vector or dot. | |
Public Member Functions | |
ChBlender (ChSystem *system) | |
void | Add (std::shared_ptr< ChPhysicsItem > item) |
Add a ChPhysicsItem object to the list of objects to render. More... | |
void | Remove (std::shared_ptr< ChPhysicsItem > item) |
Remove a ChPhysicsItem object from the list of objects to render. | |
void | AddAll () |
Add all ChPhysicsItem objects in the system to the list of objects to render. | |
void | RemoveAll () |
Remove all ChPhysicsItem objects that were previously added. | |
void | SetCustomCommands (std::shared_ptr< ChPhysicsItem > item, const std::string &commands) |
Attach custom Blender commands to the given physics item. More... | |
void | SetBasePath (const std::string &mpath) |
Set the path where all files (xxx.assets.py, output/state00001.py etc) will be saved. More... | |
void | SetBlenderFrame (const ChFrame<> mframe) |
Set transformation from Chrono frame to Blender. | |
void | SetBlenderUp_is_ChronoY () |
Set transformation from Chrono frame to Blender (in Blender, Z is up axis). Default. | |
void | SetBlenderUp_is_ChronoZ () |
Set transformation from Chrono frame to Blender (in Blender, Z is up axis). More... | |
void | SetOutputScriptFile (const std::string &filename) |
Set the filename of the output Blender script. More... | |
void | SetPictureFilebase (const std::string &filename) |
Set the filename of the image files generated by Blender. More... | |
void | SetPictureSize (unsigned int width, unsigned int height) |
Set the picture width and height - will write this in the output .ini file. | |
void | SetCamera (ChVector3d location, ChVector3d aim, double angle, bool ortho=false) |
Set the default camera position and aim point - will write this in the output .assets.py file. | |
void | SetLight (ChVector3d location, ChColor color, bool cast_shadow) |
Set the default light position and color - will write this in the output .assets.py file. | |
void | SetBackground (ChColor color) |
Set the background color - will write this in the output .assets.py file. | |
void | SetShowCOGs (bool show, double msize=0.04) |
Turn on/off the display of the COG (center of mass) of rigid bodies. More... | |
void | SetShowItemsFrames (bool show, double msize=0.05) |
Turn on/off the display of the reference coordsystems of rigid bodies, particle in particle clouds, etc. More... | |
void | SetShowAssetsFrames (bool show, double msize=0.03) |
Turn on/off the display of the reference coordsystems of each asset shape instance. More... | |
void | SetShowLinksFrames (bool show, double msize=0.04) |
Turn on/off the display of the reference coordsystems for ChLinkMate constraints. More... | |
void | SetShowContactsOff () |
Turn off the display of contacts. | |
void | SetShowContactsVectors (ContactSymbolVectorLength length_type, double scale_length, const std::string scale_attr, ContactSymbolVectorWidth width_type, double scale_width, const std::string width_attr, ContactSymbolColor color_type, ChColor const_color, const std::string color_attr, double colormap_start, double colormap_end, bool do_vector_tip=true) |
Turn on the display of contacts, using arrows to show vectors. More... | |
void | SetShowContactsSpheres (ContactSymbolSphereSize size_type, double scale_size, ContactSymbolColor color_type, ChColor const_color, double colormap_start, double colormap_end, const std::string size_attr="", const std::string color_attr="") |
Turn on the display of contacts, using spheres to show contact locations. More... | |
void | SetWireframeThickness (const double wft) |
Set thickness for wireframe mode of meshes. More... | |
double | GetWireframeThickness () const |
void | SetCustomBlenderCommandsScript (const std::string &text) |
Set a string (a text block) of custom Blender commands that you can optionally append to the Blender script file, for example adding other lights, materials, etc. More... | |
const std::string & | GetCustomBlenderCommandsScript () const |
void | SetCustomBlenderCommandsData (const std::string &text) |
Set a string (a text block) of custom Blender commands that you can optionally append to the Blender script files that are load at each timestep, e.g., state00001.py, state00002.py, for example adding other lights, materials, etc. | |
const std::string & | GetCustomBlenderCommandsData () const |
void | SetFramenumber (unsigned int fn) |
When ExportData() is called, it saves .dat files in incremental way, starting from zero: data00000.dat, data00001.dat etc., but you can override the formatted number by first calling SetFramenumber(). | |
void | ExportScript () |
Export the script that will be used by Blender that contains the definitions of assets of geometric shapes, lights, etc. More... | |
virtual void | ExportScript (const std::string &filename) override |
As ExportScript(), but overrides the filename. | |
void | ExportData () |
This function must used at each timestep to export the state of the shared assets as file(s) with incremental numbering in output/ The user should call this function in the while() loop of the simulation, once per frame. | |
virtual void | ExportData (const std::string &filename) override |
As ExportData(), but overrides the automatically generated filename. More... | |
void | SetUseSingleAssetFile (bool use) |
Set if the assets for the entre scenes at all timesteps must be appended into one single large file "exported.assets.py". More... | |
void | SetRank (int mrank) |
Se the rank of this process. More... | |
![]() | |
ChPostProcessBase (ChSystem *system) | |
virtual void | SetSystem (ChSystem *system) |
virtual ChSystem * | GetSystem () |
Additional Inherited Members | |
![]() | |
ChSystem * | mSystem |
Member Function Documentation
◆ Add()
void chrono::postprocess::ChBlender::Add | ( | std::shared_ptr< ChPhysicsItem > | item | ) |
Add a ChPhysicsItem object to the list of objects to render.
An item is added to the list only if it has a visual model.
◆ ExportData()
|
overridevirtual |
As ExportData(), but overrides the automatically generated filename.
Prefer using ExportData() so naming is automatic.
Implements chrono::postprocess::ChPostProcessBase.
◆ ExportScript()
|
inline |
Export the script that will be used by Blender that contains the definitions of assets of geometric shapes, lights, etc.
This function must be called once at the beginning of the animation.
◆ SetBasePath()
|
inline |
Set the path where all files (xxx.assets.py, output/state00001.py etc) will be saved.
The path can be absolute, or relative to the .exe current path. Note that the directory must be already existing. At the execution of ExportScript() it will create files & directories like
base_path exported.assets.py anim pic0000.bmp .... output state00000.py state00000.dat state00001.py state00001.dat ....
◆ SetBlenderUp_is_ChronoZ()
|
inline |
Set transformation from Chrono frame to Blender (in Blender, Z is up axis).
Useful when using Chrono::Vehicle, based on SAE standard with Z up.
◆ SetCustomBlenderCommandsScript()
|
inline |
Set a string (a text block) of custom Blender commands that you can optionally append to the Blender script file, for example adding other lights, materials, etc.
What you put in this string will be put at the end of the generated script, to be executed each time the menu File/Import/Chrono import is called.
◆ SetCustomCommands()
void chrono::postprocess::ChBlender::SetCustomCommands | ( | std::shared_ptr< ChPhysicsItem > | item, |
const std::string & | commands | ||
) |
Attach custom Blender commands to the given physics item.
The provided string will be inserted as-is in the assets file corresponding to the physics item. Only one commands string can be attached to any physics item; a call to this function replaces any existing commands.
◆ SetOutputScriptFile()
|
inline |
Set the filename of the output Blender script.
If not set, it defaults to "render_frames.assets.py".
◆ SetPictureFilebase()
|
inline |
Set the filename of the image files generated by Blender.
If not set, it defaults to "pic".
◆ SetRank()
|
inline |
Se the rank of this process.
This is useful when doing parallel simulations on multiple computing nodes, each with its own ChBlender exporter, each generating .py files in different directories, and later you want to load all them in a single Blender project: this is possible tanks to the "Merge" mode in the Blender plugin, but there is the risk that identifiers such as "shape_149372748349" may be duplicated in different processes, causing conflicts after loading in Blender. To fix this, for example, you set rank as 1 and 2 on two processes, so the IDs will become shape_1_149372748349 shape_2_149372748349, avoiding potential conflicts. Setting to -1 will disable the .._n_... prefix (default)
◆ SetShowAssetsFrames()
void chrono::postprocess::ChBlender::SetShowAssetsFrames | ( | bool | show, |
double | msize = 0.03 |
||
) |
Turn on/off the display of the reference coordsystems of each asset shape instance.
If setting true, you can also set the size of the symbol, in meters.
◆ SetShowCOGs()
void chrono::postprocess::ChBlender::SetShowCOGs | ( | bool | show, |
double | msize = 0.04 |
||
) |
Turn on/off the display of the COG (center of mass) of rigid bodies.
If setting true, you can also set the size of the symbol, in meters.
◆ SetShowContactsSpheres()
void chrono::postprocess::ChBlender::SetShowContactsSpheres | ( | ContactSymbolSphereSize | size_type, |
double | scale_size, | ||
ContactSymbolColor | color_type, | ||
ChColor | const_color, | ||
double | colormap_start, | ||
double | colormap_end, | ||
const std::string | size_attr = "" , |
||
const std::string | color_attr = "" |
||
) |
Turn on the display of contacts, using spheres to show contact locations.
The size of the arrow depends on force strength multiplied by 'scale_length'.
◆ SetShowContactsVectors()
void chrono::postprocess::ChBlender::SetShowContactsVectors | ( | ContactSymbolVectorLength | length_type, |
double | scale_length, | ||
const std::string | scale_attr, | ||
ContactSymbolVectorWidth | width_type, | ||
double | scale_width, | ||
const std::string | width_attr, | ||
ContactSymbolColor | color_type, | ||
ChColor | const_color, | ||
const std::string | color_attr, | ||
double | colormap_start, | ||
double | colormap_end, | ||
bool | do_vector_tip = true |
||
) |
Turn on the display of contacts, using arrows to show vectors.
The lenght of the arrow can be: constant, or force strength multiplied by 'scale_length', or property multiplied by 'scale_length'. The width of the arrow can be: constant, or force strength multiplied by 'scale_length', or property multiplied by 'scale_length'.
◆ SetShowItemsFrames()
void chrono::postprocess::ChBlender::SetShowItemsFrames | ( | bool | show, |
double | msize = 0.05 |
||
) |
Turn on/off the display of the reference coordsystems of rigid bodies, particle in particle clouds, etc.
If setting true, you can also set the size of the symbol, in meters.
◆ SetShowLinksFrames()
void chrono::postprocess::ChBlender::SetShowLinksFrames | ( | bool | show, |
double | msize = 0.04 |
||
) |
Turn on/off the display of the reference coordsystems for ChLinkMate constraints.
If setting true, you can also set the size of the symbol, in meters.
◆ SetUseSingleAssetFile()
|
inline |
Set if the assets for the entre scenes at all timesteps must be appended into one single large file "exported.assets.py".
If not, assets will be written inside each state00001.dat, state00002.dat, etc files; this would waste more disk space but would allow assets whose settings change during time (ex time-changing colors)
◆ SetWireframeThickness()
|
inline |
Set thickness for wireframe mode of meshes.
If a ChVisualShapeTriangleMesh asset was set as SetWireframe(true), it will be rendered in Blender as a cage of thin cylinders. This setting sets how thick the tubes.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_postprocess/ChBlender.h
- /builds/uwsbel/chrono/src/chrono_postprocess/ChBlender.cpp