Description
Concrete class for a (rigid) road loaded from an OpenCRG file.
This type of terrain can be used in conjunction with tire models that perform their own collision detection (e.g., ChTMeasy ChPac89, ChPac02, ChFiala).
#include <CRGTerrain.h>
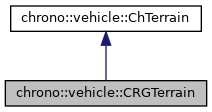
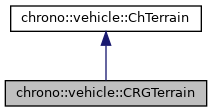
Public Member Functions | |
CRGTerrain (ChSystem *system) | |
Construct a default CRGTerrain. More... | |
void | EnableVerbose (bool val) |
Enable verbose messages from CRG (default : false). | |
void | UseMeshVisualization (bool val) |
Set the road visualization mode (mesh or boundary lines). More... | |
void | SetContactFrictionCoefficient (float friction_coefficient) |
Set coefficient of friction. More... | |
void | Initialize (const std::string &crg_file) |
Initialize the CRGTerrain from the specified OpenCRG file. More... | |
virtual double | GetHeight (const ChVector3d &loc) const override |
Get the terrain height below the specified location. | |
virtual ChVector3d | GetNormal (const ChVector3d &loc) const override |
Get the terrain normal at the point below the specified location. | |
virtual float | GetCoefficientFriction (const ChVector3d &loc) const override |
Get the terrain coefficient of friction at the point below the specified location. More... | |
std::shared_ptr< ChBezierCurve > | GetRoadCenterLine () |
Get the road center line as a Bezier curve. | |
std::shared_ptr< ChBezierCurve > | GetRoadBoundaryLeft () const |
Get the road left boundary as a Bezier curve. | |
std::shared_ptr< ChBezierCurve > | GetRoadBoundaryRight () const |
Get the road right boundary as a Bezier curve. | |
std::shared_ptr< ChTriangleMeshConnected > | GetMesh () const |
Get the road mesh. | |
bool | IsPathClosed () |
Is the road a round course (closed loop)? | |
double | GetLength () |
Get length of the road. | |
double | GetWidth () |
Get width of the road. | |
double | GetStartHeading () |
Get start heading (in radians), can be different from zero for georeferenced roads. | |
ChCoordsys | GetStartPosition () |
Get the start position (location and orientation). More... | |
std::shared_ptr< ChBody > | GetGround () const |
Get ground body (carries visualization assets). | |
void | SetRoadsidePostDistance (double dist) |
Generate roadside posts left and right (optional) | |
void | SetRoadDiffuseTextureFile (std::string diffTexFile) |
Use a texture file, if mesh representation is desired (optional, should not be used with Irrlicht) | |
void | SetRoadNormalTextureFile (std::string normTexFile) |
void | SetRoadRoughnessTextureFile (std::string roughTexFile) |
void | ExportMeshWavefront (const std::string &out_dir) |
Export road mesh to Wavefront file. | |
void | ExportMeshPovray (const std::string &out_dir) |
Export the patch mesh as a macro in a POV-Ray include file. | |
void | ExportCurvesPovray (const std::string &out_dir) |
Export the road boundary curves as a macro in a POV-Ray include file. More... | |
![]() | |
virtual void | Synchronize (double time) |
Update the state of the terrain system at the specified time. | |
virtual void | Advance (double step) |
Advance the state of the terrain system by the specified duration. | |
virtual void | GetProperties (const ChVector3d &loc, double &height, ChVector3d &normal, float &friction) const |
Get all terrain characteristics at the point below the specified location. | |
void | RegisterHeightFunctor (std::shared_ptr< HeightFunctor > functor) |
Specify the functor object to provide the terrain height at given locations. More... | |
void | RegisterNormalFunctor (std::shared_ptr< NormalFunctor > functor) |
Specify the functor object to provide the terrain normal at given locations. More... | |
void | RegisterFrictionFunctor (std::shared_ptr< FrictionFunctor > functor) |
Specify the functor object to provide the coefficient of friction at given locations. | |
Additional Inherited Members | |
![]() | |
std::shared_ptr< HeightFunctor > | m_height_fun |
functor for location-dependent terrain height | |
std::shared_ptr< NormalFunctor > | m_normal_fun |
functor for location-dependent terrain normal | |
std::shared_ptr< FrictionFunctor > | m_friction_fun |
functor for location-dependent coefficient of friction | |
Constructor & Destructor Documentation
◆ CRGTerrain()
chrono::vehicle::CRGTerrain::CRGTerrain | ( | ChSystem * | system | ) |
Construct a default CRGTerrain.
The user should call optional Set methods and then Initialize.
- Parameters
-
[in] system pointer to the containing multibody system
Member Function Documentation
◆ ExportCurvesPovray()
void chrono::vehicle::CRGTerrain::ExportCurvesPovray | ( | const std::string & | out_dir | ) |
Export the road boundary curves as a macro in a POV-Ray include file.
This function does nothing if using mesh visualization for the road.
◆ GetCoefficientFriction()
|
overridevirtual |
Get the terrain coefficient of friction at the point below the specified location.
This coefficient of friction value may be used by certain tire models to modify the tire characteristics, but it will have no effect on the interaction of the terrain with other objects (including tire models that do not explicitly use it). For CRGTerrain, this function defers to the user-provided functor object of type ChTerrain::FrictionFunctor, if one was specified. Otherwise, it returns the constant value specified at construction.
Reimplemented from chrono::vehicle::ChTerrain.
◆ GetStartPosition()
ChCoordsys chrono::vehicle::CRGTerrain::GetStartPosition | ( | ) |
Get the start position (location and orientation).
This is the (x,y,z) road location at CRG parameters u=v=0.
◆ Initialize()
void chrono::vehicle::CRGTerrain::Initialize | ( | const std::string & | crg_file | ) |
Initialize the CRGTerrain from the specified OpenCRG file.
- Parameters
-
[in] crg_file OpenCRG road specification file
◆ SetContactFrictionCoefficient()
|
inline |
Set coefficient of friction.
The default value is 0.8
◆ UseMeshVisualization()
|
inline |
Set the road visualization mode (mesh or boundary lines).
Default: mesh.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_vehicle/terrain/CRGTerrain.h
- /builds/uwsbel/chrono/src/chrono_vehicle/terrain/CRGTerrain.cpp