Description
Base class for chrono wheeled trailer systems.
This class provides the interface between the trailer system and other systems (vehicle, tires, etc.). The reference frame for a trailer follows the ISO standard: Z-axis up, X-axis pointing forward, and Y-axis towards the left of the vehicle.
#include <ChWheeledTrailer.h>
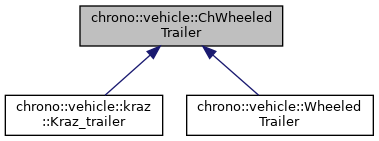
Public Member Functions | |
virtual | ~ChWheeledTrailer () |
Destructor. | |
const std::string & | GetName () const |
Get the name identifier for this vehicle. | |
void | SetName (const std::string &name) |
Set the name identifier for this vehicle. | |
virtual unsigned int | GetNumberAxles () const =0 |
Return the number of axles for this trailer. | |
virtual std::string | GetTemplateName () const |
Get the name of the trailer system template. | |
std::shared_ptr< ChChassisRear > | GetChassis () const |
Get the trailer chassis subsystem. | |
const chrono::vehicle::ChAxleList & | GetAxles () const |
Get all trailer axle subsystems. | |
std::shared_ptr< chrono::vehicle::ChAxle > | GetAxle (int id) const |
Get the specified trailer axle subsystem. | |
void | SetChassisVisualizationType (VisualizationType vis) |
Set visualization mode for the chassis subsystem. More... | |
void | SetSuspensionVisualizationType (VisualizationType vis) |
Set visualization type for the suspension subsystems. More... | |
void | SetWheelVisualizationType (VisualizationType vis) |
Set visualization type for the wheel subsystems. More... | |
void | SetTireVisualizationType (VisualizationType vis) |
Set visualization type for the tire subsystems. More... | |
virtual void | Initialize (std::shared_ptr< ChChassis > frontChassis) |
Initialize this trailer relative to the specified front chassis. More... | |
void | InitializeTire (std::shared_ptr< ChTire > tire, std::shared_ptr< ChWheel > wheel, VisualizationType tire_vis=VisualizationType::PRIMITIVES, ChTire::CollisionType tire_coll=ChTire::CollisionType::SINGLE_POINT) |
Initialize the given tire and attach it to the specified wheel. More... | |
void | Synchronize (double time, const DriverInputs &driver_inputs, const ChTerrain &terrain) |
Update the state of this trailer at the current time. More... | |
void | Advance (double step) |
Advance the state of this trailer by the specified time step. More... | |
Protected Member Functions | |
ChWheeledTrailer (const std::string &name, ChSystem *system) | |
Construct a trailer system using the specified ChSystem. More... | |
Protected Attributes | |
std::string | m_name |
trailer system name | |
std::shared_ptr< ChChassisRear > | m_chassis |
trailer chassis | |
std::shared_ptr< ChChassisConnector > | m_connector |
connector to pulling vehicle | |
chrono::vehicle::ChAxleList | m_axles |
list of axle subsystems | |
Constructor & Destructor Documentation
◆ ChWheeledTrailer()
|
protected |
Construct a trailer system using the specified ChSystem.
- Parameters
-
[in] name trailer system name [in] system containing mechanical system
Member Function Documentation
◆ Advance()
void chrono::vehicle::ChWheeledTrailer::Advance | ( | double | step | ) |
Advance the state of this trailer by the specified time step.
This function advances the states of all associated tires.
◆ Initialize()
|
virtual |
Initialize this trailer relative to the specified front chassis.
This base class implementation only initializes the trailer chassis and connector subsystems. Derived classes must extend this function to initialize the axle subsystems.
Reimplemented in chrono::vehicle::kraz::Kraz_trailer, and chrono::vehicle::WheeledTrailer.
◆ InitializeTire()
void chrono::vehicle::ChWheeledTrailer::InitializeTire | ( | std::shared_ptr< ChTire > | tire, |
std::shared_ptr< ChWheel > | wheel, | ||
VisualizationType | tire_vis = VisualizationType::PRIMITIVES , |
||
ChTire::CollisionType | tire_coll = ChTire::CollisionType::SINGLE_POINT |
||
) |
Initialize the given tire and attach it to the specified wheel.
Optionally, specify tire visualization mode and tire-terrain collision detection method. This function should be called only after trailer initialization.
◆ SetChassisVisualizationType()
void chrono::vehicle::ChWheeledTrailer::SetChassisVisualizationType | ( | VisualizationType | vis | ) |
Set visualization mode for the chassis subsystem.
This function should be called only after trailer initialization.
◆ SetSuspensionVisualizationType()
void chrono::vehicle::ChWheeledTrailer::SetSuspensionVisualizationType | ( | VisualizationType | vis | ) |
Set visualization type for the suspension subsystems.
This function should be called only after trailer initialization.
◆ SetTireVisualizationType()
void chrono::vehicle::ChWheeledTrailer::SetTireVisualizationType | ( | VisualizationType | vis | ) |
Set visualization type for the tire subsystems.
This function should be called only after trailer and tire initialization.
◆ SetWheelVisualizationType()
void chrono::vehicle::ChWheeledTrailer::SetWheelVisualizationType | ( | VisualizationType | vis | ) |
Set visualization type for the wheel subsystems.
This function should be called only after trailer initialization.
◆ Synchronize()
void chrono::vehicle::ChWheeledTrailer::Synchronize | ( | double | time, |
const DriverInputs & | driver_inputs, | ||
const ChTerrain & | terrain | ||
) |
Update the state of this trailer at the current time.
The trailer system is provided the current driver inputs and a reference to the terrain system.
- Parameters
-
[in] time current time [in] driver_inputs current driver inputs [in] terrain reference to the terrain system
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_vehicle/wheeled_vehicle/ChWheeledTrailer.h
- /builds/uwsbel/chrono/src/chrono_vehicle/wheeled_vehicle/ChWheeledTrailer.cpp