Description
Granular terrain model.
This class implements a rectangular patch of granular terrain with spherical particles. Boundary conditions (model of a container bin) are imposed through a custom collision detection object.
#include <GranularTerrain.h>
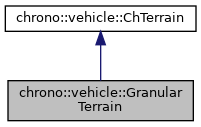
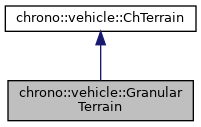
Public Member Functions | |
GranularTerrain (ChSystem *system) | |
Construct a default GranularTerrain. More... | |
void | SetContactMaterial (std::shared_ptr< ChContactMaterial > material) |
Set contact material (must be consistent with the containing system). | |
std::shared_ptr< ChContactMaterial > | GetContactMaterial () const |
Get the current contact material. | |
void | SetCollisionEnvelope (double envelope) |
Set outward collision envelope. More... | |
void | SetMinNumParticles (unsigned int min_num_particles) |
Set the minimum number of particles to be generated (default: 0). | |
void | EnableVerbose (bool val) |
Enable/disable verbose output (default: false). | |
void | EnableRoughSurface (int num_spheres_x, int num_spheres_y) |
Enable creation of particles fixed to bottom container. More... | |
void | EnableMovingPatch (std::shared_ptr< ChBody > body, double buffer_distance, double shift_distance, const ChVector3d &init_vel=ChVector3d()) |
Enable moving patch and set parameters. More... | |
void | EnableVisualization (bool val) |
Enable/disable visualization of boundaries (default: false). | |
bool | IsVisualizationEnabled () const |
std::shared_ptr< ChBody > | GetGroundBody () |
Return a handle to the ground body. | |
void | Initialize (const ChVector3d ¢er, double length, double width, unsigned int num_layers, double radius, double density, const ChVector3d &init_vel=ChVector3d()) |
Initialize the granular terrain system. More... | |
virtual void | Synchronize (double time) override |
Update the state of the terrain system at the specified time. | |
double | GetPatchFront () const |
Get current front boundary location (in positive X direction). | |
double | GetPatchRear () const |
Get current rear boundary location (in negative X direction). | |
double | GetPatchLeft () const |
Get left boundary location (in positive Y direction). | |
double | GetPatchRight () const |
Get right boundary location (in negative Y direction). | |
double | GetPatchBottom () const |
Get bottom boundary location. | |
bool | PatchMoved () const |
Report if the patch was moved during the last call to Synchronize(). | |
unsigned int | GetNumParticles () const |
Get the number of particles. | |
virtual double | GetHeight (const ChVector3d &loc) const override |
Get the terrain height below the specified location. More... | |
virtual chrono::ChVector3d | GetNormal (const ChVector3d &loc) const override |
Get the terrain normal at the point below the specified location. | |
virtual float | GetCoefficientFriction (const ChVector3d &loc) const override |
Get the terrain coefficient of friction at the point below the specified location. More... | |
![]() | |
virtual void | Advance (double step) |
Advance the state of the terrain system by the specified duration. | |
virtual void | GetProperties (const ChVector3d &loc, double &height, ChVector3d &normal, float &friction) const |
Get all terrain characteristics at the point below the specified location. | |
void | RegisterHeightFunctor (std::shared_ptr< HeightFunctor > functor) |
Specify the functor object to provide the terrain height at given locations. More... | |
void | RegisterNormalFunctor (std::shared_ptr< NormalFunctor > functor) |
Specify the functor object to provide the terrain normal at given locations. More... | |
void | RegisterFrictionFunctor (std::shared_ptr< FrictionFunctor > functor) |
Specify the functor object to provide the coefficient of friction at given locations. | |
Friends | |
class | BoundaryContact |
Additional Inherited Members | |
![]() | |
std::shared_ptr< HeightFunctor > | m_height_fun |
functor for location-dependent terrain height | |
std::shared_ptr< NormalFunctor > | m_normal_fun |
functor for location-dependent terrain normal | |
std::shared_ptr< FrictionFunctor > | m_friction_fun |
functor for location-dependent coefficient of friction | |
Constructor & Destructor Documentation
◆ GranularTerrain()
chrono::vehicle::GranularTerrain::GranularTerrain | ( | ChSystem * | system | ) |
Construct a default GranularTerrain.
The user is responsible for calling various Set methods before Initialize.
- Parameters
-
[in] system pointer to the containing multibody system
Member Function Documentation
◆ EnableMovingPatch()
void chrono::vehicle::GranularTerrain::EnableMovingPatch | ( | std::shared_ptr< ChBody > | body, |
double | buffer_distance, | ||
double | shift_distance, | ||
const ChVector3d & | init_vel = ChVector3d() |
||
) |
Enable moving patch and set parameters.
- Parameters
-
body monitored body buffer_distance look-ahead distance shift_distance chunk size of relocated particles init_vel initial particle velocity
◆ EnableRoughSurface()
void chrono::vehicle::GranularTerrain::EnableRoughSurface | ( | int | num_spheres_x, |
int | num_spheres_y | ||
) |
Enable creation of particles fixed to bottom container.
- Parameters
-
num_spheres_x number of fixed spheres in X direction num_spheres_y number of fixed spheres in Y direction
◆ GetCoefficientFriction()
|
overridevirtual |
Get the terrain coefficient of friction at the point below the specified location.
This coefficient of friction value may be used by certain tire models to modify the tire characteristics, but it will have no effect on the interaction of the terrain with other objects (including tire models that do not explicitly use it). For GranularTerrain, this function defers to the user-provided functor object of type ChTerrain::FrictionFunctor, if one was specified. Otherwise, it returns the constant value specified through SetContactFrictionCoefficient.
Reimplemented from chrono::vehicle::ChTerrain.
◆ GetHeight()
|
overridevirtual |
Get the terrain height below the specified location.
This function returns the highest point over all granular particles.
Reimplemented from chrono::vehicle::ChTerrain.
◆ Initialize()
void chrono::vehicle::GranularTerrain::Initialize | ( | const ChVector3d & | center, |
double | length, | ||
double | width, | ||
unsigned int | num_layers, | ||
double | radius, | ||
double | density, | ||
const ChVector3d & | init_vel = ChVector3d() |
||
) |
Initialize the granular terrain system.
The granular material is created in successive layers within the specified volume, using the specified generator, until the number of particles exceeds the specified minimum value (see SetMinNumParticles). The initial particle locations are obtained with Poisson Disk sampling, using the given minimum separation distance.
- Parameters
-
[in] center center of bottom [in] length patch dimension in X direction [in] width patch dimension in Y direction [in] num_layers number of layers [in] radius particle radius [in] density particle density [in] init_vel particle initial velocity
◆ SetCollisionEnvelope()
|
inline |
Set outward collision envelope.
This value is used for the internal custom collision detection for imposing boundary conditions. Note that if the underlying system is of SMC type (i.e., using a penalty-based contact method), the envelope is automatically set to 0. For NSC systems (i.e., when using a complementarity-based contact method), if the envelope is not specified, the default value is 5% of the particle radius.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_vehicle/terrain/GranularTerrain.h
- /builds/uwsbel/chrono/src/chrono_vehicle/terrain/GranularTerrain.cpp