chrono::vehicle::ChDriver Class Reference
Description
Base class for a vehicle driver system.
A driver system must be able to report the current values of the inputs (throttle, steering, braking). A concrete driver class must set the member variables m_throttle, m_steering, and m_braking.
#include <ChDriver.h>
Inheritance diagram for chrono::vehicle::ChDriver:
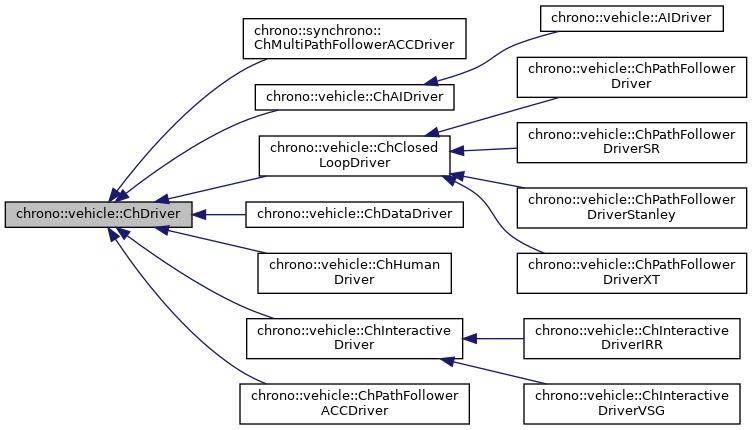
Collaboration diagram for chrono::vehicle::ChDriver:
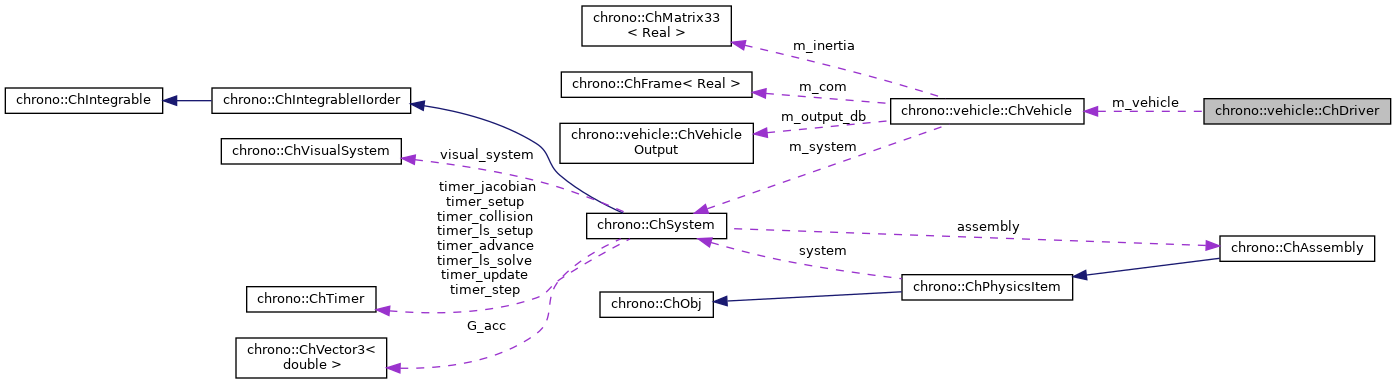
Public Member Functions | |
ChDriver (ChVehicle &vehicle) | |
Construct a driver subsystem associated with the given vehicle. | |
double | GetThrottle () const |
Get the driver throttle input (in the range [0,1]). | |
double | GetSteering () const |
Get the driver steering input (in the range [-1,+1]). | |
double | GetBraking () const |
Get the driver braking input (in the range [0,1]). | |
double | GetClutch () const |
Get the driver clutch input (in the range [0,1]). | |
DriverInputs | GetInputs () const |
Get all current inputs at once. | |
virtual void | Initialize () |
Initialize this driver system. | |
virtual void | Synchronize (double time) |
Update the state of this driver system at the current time. | |
virtual void | Advance (double step) |
Advance the state of this driver system by the specified time step. | |
bool | LogInit (const std::string &filename) |
Initialize output file for recording driver inputs. | |
bool | Log (double time) |
Record the current driver inputs to the log file. | |
void | SetSteering (double steering) |
Overwrite the value for the driver steering input (input is clamped in [-1,+1]). | |
void | SetThrottle (double throttle) |
Overwrite the value for the driver throttle input (input is clamped in [0,+1]). | |
void | SetBraking (double braking) |
Overwrite the value for the driver braking input (input is clamped in [0,+1]). | |
void | SetClutch (double clutch) |
Overwrite the value for the clutch braking input (input is clamped in [0,+1]). | |
Protected Attributes | |
ChVehicle & | m_vehicle |
reference to associated vehicle | |
double | m_throttle |
current value of throttle input | |
double | m_steering |
current value of steering input | |
double | m_braking |
current value of braking input | |
double | m_clutch |
current value of clutch input | |
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_vehicle/ChDriver.h
- /builds/uwsbel/chrono/src/chrono_vehicle/ChDriver.cpp