Description
Base class for a Chrono run-time visualization system.
#include <ChVisualSystem.h>
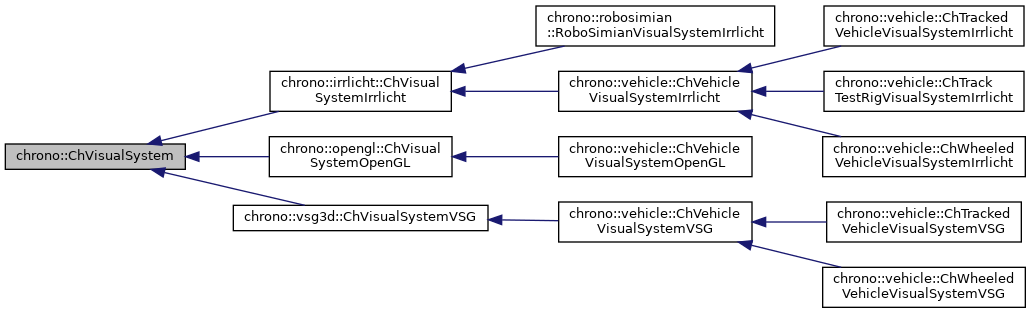
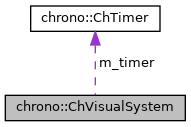
Public Types | |
enum | Type { Type::IRRLICHT, Type::VSG, Type::OptiX, NONE } |
Supported run-time visualization systems. More... | |
Public Member Functions | |
void | SetVerbose (bool verbose) |
Enable/disable information terminal output during initialization (default: false). | |
bool | IsInitialized () const |
Indicate whether the visual system was initialized or not. | |
virtual void | AttachSystem (ChSystem *sys) |
Attach a Chrono system to this visualization system. | |
virtual void | Initialize () |
Initialize the visualization system. More... | |
virtual void | BindAll () |
Process all visual assets in the associated Chrono systems. More... | |
virtual void | BindItem (std::shared_ptr< ChPhysicsItem > item) |
Process the visual assets for the specified physics item. More... | |
virtual void | UnbindItem (std::shared_ptr< ChPhysicsItem > item) |
Remove the visual assets for the specified physics item from this visualization system. | |
virtual int | AddCamera (const ChVector3d &pos, ChVector3d targ=VNULL) |
Add a camera to the 3D scene. More... | |
virtual void | AddGrid (double x_step, double y_step, int nx, int ny, ChCoordsys<> pos=CSYSNORM, ChColor col=ChColor(0.1f, 0.1f, 0.1f)) |
Add a grid with specified parameters in the x-y plane of the given frame. More... | |
void | SetBackgroundColor (const ChColor &color) |
Set window background color. | |
virtual void | SetCameraPosition (int id, const ChVector3d &pos) |
Set the location of the specified camera. | |
virtual void | SetCameraTarget (int id, const ChVector3d &target) |
Set the target (look-at) point of the specified camera. | |
virtual void | SetCameraPosition (const ChVector3d &pos) |
Set the location of the current (active) camera. | |
virtual void | SetCameraTarget (const ChVector3d &target) |
Set the target (look-at) point of the current (active) camera. | |
virtual ChVector3d | GetCameraPosition () const |
Get the location of the current (active) camera. | |
virtual ChVector3d | GetCameraTarget () const |
Get the target (look-at) point of the current (active) camera. | |
void | UpdateCamera (int id, const ChVector3d &pos, ChVector3d target) |
Update the location and/or target points of the specified camera. | |
void | UpdateCamera (const ChVector3d &pos, ChVector3d target) |
virtual int | AddVisualModel (std::shared_ptr< ChVisualModel > model, const ChFrame<> &frame) |
Add a visual model not associated with a physical item. More... | |
virtual int | AddVisualModel (std::shared_ptr< ChVisualShape > shape, const ChFrame<> &frame) |
Add a visual model not associated with a physical item. More... | |
virtual void | UpdateVisualModel (int id, const ChFrame<> &frame) |
Update the position of the specified visualization-only model. | |
virtual bool | Run () |
Run the visualization system. More... | |
virtual void | Quit () |
Terminate the visualization system. | |
virtual void | BeginScene () |
Perform any necessary operations at the beginning of each rendering frame. | |
virtual void | Render () |
Draw all 3D shapes and GUI elements at the current frame. More... | |
virtual void | RenderFrame (const ChFrame<> &frame, double axis_length=1) |
Render the specified reference frame. | |
virtual void | RenderCOGFrames (double axis_length=1) |
Render COG frames for all bodies in the system. | |
virtual void | EndScene () |
Perform any necessary operations ar the end of each rendering frame. | |
std::vector< ChSystem * > | GetSystems () const |
Get the list of associated Chrono systems. | |
ChSystem & | GetSystem (int i) const |
Get the specified associated Chrono system. | |
double | GetSimulationTime () const |
Return the current simulated time. More... | |
double | GetRTF () const |
Return the overall real time factor. More... | |
double | GetSimulationRTF (unsigned int i) const |
Return the simulation real-time factor (simulation time / simulated time) for the specified associated system. More... | |
std::vector< double > | GetSimulationRTFs () const |
Return the simulation real-time factor (simulation time / simulated time) for all associated system. More... | |
unsigned int | GetNumBodies () const |
Get the number of bodies (across all visualized systems). More... | |
unsigned int | GetNumLinks () const |
Get the number of links (across all visualized systems). More... | |
unsigned int | GetNumMeshes () const |
Get the number of meshes (across all visualized systems). | |
unsigned int | GetNumShafts () const |
Get the number of shafts (across all visualized systems). | |
unsigned int | GetNumStates () const |
Get the number of coordinates at the velocity level (across all visualized systems). | |
unsigned int | GetNumConstraints () const |
Get the number of scalar constraints (across all visualized systems). | |
unsigned int | GetNumContacts () const |
Gets the number of contacts. | |
virtual void | WriteImageToFile (const std::string &filename) |
Create a snapshot of the last rendered frame and save it to the provided file. More... | |
void | SetImageOutputDirectory (const std::string &dir) |
Set output directory for saving frame snapshots (default: "."). | |
void | SetImageOutput (bool val) |
Enable/disable writing of frame snapshots to file. | |
Protected Member Functions | |
virtual void | OnSetup (ChSystem *sys) |
Perform any necessary setup operations at the beginning of a time step. More... | |
virtual void | OnUpdate (ChSystem *sys) |
Perform any necessary update operations at the end of a time step. More... | |
virtual void | OnClear (ChSystem *sys) |
Remove all visualization objects from this visualization system. More... | |
Protected Attributes | |
bool | m_verbose |
terminal output | |
bool | m_initialized |
visual system initialized | |
ChColor | m_background_color |
window background color | |
ChTimer | m_timer |
timer for evaluating RTF | |
double | m_rtf |
overall real time factor | |
std::vector< ChSystem * > | m_systems |
associated Chrono system(s) | |
bool | m_write_images |
if true, save snapshots | |
std::string | m_image_dir |
directory for image files | |
Friends | |
class | ChSystem |
Member Enumeration Documentation
◆ Type
|
strong |
Member Function Documentation
◆ AddCamera()
|
inlinevirtual |
Add a camera to the 3D scene.
Return an ID which can be used later to modify camera location and/or target points. A concrete visualization system may or may not support multiuple cameras.
Reimplemented in chrono::vsg3d::ChVisualSystemVSG, and chrono::irrlicht::ChVisualSystemIrrlicht.
◆ AddGrid()
|
inlinevirtual |
Add a grid with specified parameters in the x-y plane of the given frame.
- Parameters
-
x_step grid cell size in X direction y_step grid cell size in Y direction nx number of cells in X direction ny number of cells in Y direction pos grid reference frame col grid line color
Reimplemented in chrono::vsg3d::ChVisualSystemVSG, and chrono::irrlicht::ChVisualSystemIrrlicht.
◆ AddVisualModel() [1/2]
|
inlinevirtual |
Add a visual model not associated with a physical item.
Return an ID which can be used later to modify the position of this visual model.
Reimplemented in chrono::irrlicht::ChVisualSystemIrrlicht, and chrono::vsg3d::ChVisualSystemVSG.
◆ AddVisualModel() [2/2]
|
inlinevirtual |
Add a visual model not associated with a physical item.
This version constructs a visual model consisting of the single specified shape Return an ID which can be used later to modify the position of this visual model.
Reimplemented in chrono::irrlicht::ChVisualSystemIrrlicht, and chrono::vsg3d::ChVisualSystemVSG.
◆ BindAll()
|
inlinevirtual |
Process all visual assets in the associated Chrono systems.
This function is called by default for a Chrono system attached to this visualization system during initialization, but can also be called later if further modifications to visualization assets occur.
Reimplemented in chrono::irrlicht::ChVisualSystemIrrlicht, and chrono::vsg3d::ChVisualSystemVSG.
◆ BindItem()
|
inlinevirtual |
Process the visual assets for the specified physics item.
This function must be called if a new physics item is added to the system or if changes to its visual model occur after the visualization system was attached to the Chrono system.
Reimplemented in chrono::irrlicht::ChVisualSystemIrrlicht, and chrono::vsg3d::ChVisualSystemVSG.
◆ GetNumBodies()
unsigned int chrono::ChVisualSystem::GetNumBodies | ( | ) | const |
Get the number of bodies (across all visualized systems).
The reported number represents only active bodies, excluding sleeping or fixed.
◆ GetNumLinks()
unsigned int chrono::ChVisualSystem::GetNumLinks | ( | ) | const |
Get the number of links (across all visualized systems).
The reported number represents only active bodies, excluding sleeping or fixed.
◆ GetRTF()
|
inline |
Return the overall real time factor.
This value represents the ratio between the wall clock time elapsed between two render frames and the duration by which simulation was advanced in this interval.
◆ GetSimulationRTF()
double chrono::ChVisualSystem::GetSimulationRTF | ( | unsigned int | i | ) | const |
Return the simulation real-time factor (simulation time / simulated time) for the specified associated system.
See ChSystem::GetRTF.
◆ GetSimulationRTFs()
std::vector< double > chrono::ChVisualSystem::GetSimulationRTFs | ( | ) | const |
Return the simulation real-time factor (simulation time / simulated time) for all associated system.
See ChSystem::GetRTF.
◆ GetSimulationTime()
double chrono::ChVisualSystem::GetSimulationTime | ( | ) | const |
Return the current simulated time.
The default value returned by this base class is the time from the first associated system (if any).
◆ Initialize()
|
inlinevirtual |
Initialize the visualization system.
This call must trigger a parsing of the associated Chrono systems to process all visual models. A derived class must ensure that this function is called only once (use the m_initialized flag).
Reimplemented in chrono::irrlicht::ChVisualSystemIrrlicht, chrono::modal::ChModalVisualSystemIrrlicht< ScalarType >, chrono::vehicle::ChVehicleVisualSystemIrrlicht, chrono::vsg3d::ChVisualSystemVSG, chrono::vehicle::ChTrackTestRigVisualSystemIRR, chrono::vehicle::ChVehicleVisualSystemVSG, chrono::vehicle::ChSuspensionTestRigVisualSystemIRR, chrono::vehicle::ChSuspensionTestRigVisualSystemVSG, and chrono::vehicle::ChTrackTestRigVisualSystemVSG.
◆ OnClear()
|
inlineprotectedvirtual |
Remove all visualization objects from this visualization system.
Called by an associated ChSystem.
Reimplemented in chrono::irrlicht::ChVisualSystemIrrlicht.
◆ OnSetup()
|
inlineprotectedvirtual |
Perform any necessary setup operations at the beginning of a time step.
Called by an associated ChSystem.
Reimplemented in chrono::irrlicht::ChVisualSystemIrrlicht, and chrono::vsg3d::ChVisualSystemVSG.
◆ OnUpdate()
|
inlineprotectedvirtual |
Perform any necessary update operations at the end of a time step.
Called by an associated ChSystem.
Reimplemented in chrono::irrlicht::ChVisualSystemIrrlicht.
◆ Render()
|
virtual |
Draw all 3D shapes and GUI elements at the current frame.
This function is typically called inside a loop such as
while(vis->Run()) { ... vis->Render(); ... }
The default implementation only updates the overall RTF.
Reimplemented in chrono::irrlicht::ChVisualSystemIrrlicht, chrono::modal::ChModalVisualSystemIrrlicht< ScalarType >, chrono::vsg3d::ChVisualSystemVSG, chrono::vehicle::ChVehicleVisualSystemIrrlicht, and chrono::vehicle::ChTrackTestRigVisualSystemIRR.
◆ Run()
|
inlinevirtual |
Run the visualization system.
Returns false
if the system must shut down.
Reimplemented in chrono::irrlicht::ChVisualSystemIrrlicht, and chrono::vsg3d::ChVisualSystemVSG.
◆ WriteImageToFile()
|
inlinevirtual |
Create a snapshot of the last rendered frame and save it to the provided file.
The file extension determines the image format.
Reimplemented in chrono::irrlicht::ChVisualSystemIrrlicht, and chrono::vsg3d::ChVisualSystemVSG.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/assets/ChVisualSystem.h
- /builds/uwsbel/chrono/src/chrono/assets/ChVisualSystem.cpp