Description
OpenGL-based Chrono run-time visualization system.
#include <ChVisualSystemOpenGL.h>
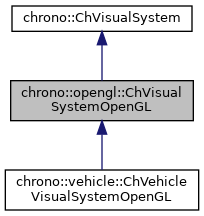
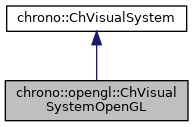
Public Member Functions | |
void | SetWindowSize (unsigned int width, unsigned int height) |
Set the window size (default 640x480). More... | |
void | SetWindowTitle (const std::string &win_title) |
Set the window title (default ""). More... | |
void | SetCameraVertical (CameraVerticalDir vert) |
Set camera vertical direction (default CameraVerticalDir::Z). | |
void | SetCameraVertical (const ChVector3d &up) |
Set camera vertical direction (default (0,0,1)). | |
void | SetCameraProperties (float scale=0.5f, float near_clip_dist=0.1f, float far_clip_dist=1000.0f) |
Set camera scale and clip distances. More... | |
void | SetRenderMode (RenderMode mode) |
Set render mode for solid objects (default: SOLID) | |
void | SetParticleRenderMode (RenderMode mode, float radius) |
Set render mode for particle systems (default: POINTS) and size. | |
virtual void | AttachSystem (ChSystem *sys) override |
Attach a Chrono system to the run-time visualization system. More... | |
void | AttachStatsRenderer (std::shared_ptr< ChOpenGLStats > renderer) |
Attach a user-defined text renderer for stats overlay. More... | |
void | EnableStats (bool state) |
Enable/disable stats overlay display (default: true). | |
virtual void | Initialize () override |
Initialize the visualization system. More... | |
virtual int | AddCamera (const ChVector3d &pos, ChVector3d targ=VNULL) override |
Add a camera to the 3d scene. | |
virtual void | SetCameraPosition (int id, const ChVector3d &pos) override |
Set the location of the specified camera. | |
virtual void | SetCameraTarget (int id, const ChVector3d &target) override |
Set the target (look-at) point of the specified camera. | |
virtual void | SetCameraPosition (const ChVector3d &pos) override |
Set the location of the current (active) camera. | |
virtual void | SetCameraTarget (const ChVector3d &target) override |
Set the target (look-at) point of the current (active) camera. | |
void | AddUserEventReceiver (std::shared_ptr< ChOpenGLEventCB > receiver) |
Attach a custom event receiver to the application. | |
void | AttachParticleSelector (std::shared_ptr< ChOpenGLParticleCB > selector) |
Attach a custom particle rendering selector. | |
virtual void | BindAll () override |
Process all visual assets in the associated ChSystem. More... | |
virtual void | BindItem (std::shared_ptr< ChPhysicsItem > item) override |
Process the visual assets for the spcified physics item. More... | |
virtual bool | Run () override |
Run the Irrlicht device. More... | |
virtual void | Quit () override |
Terminate the visualization system. | |
virtual void | BeginScene () override |
Perform any necessary operations at the beginning of each rendering frame. | |
virtual void | Render () override |
Draw all 3D shapes and GUI elements at the current frame. More... | |
virtual void | EndScene () override |
End the scene draw at the end of each animation frame. | |
virtual void | WriteImageToFile (const std::string &filename) override |
Create a snapshot of the last rendered frame and save it to the provided file. More... | |
![]() | |
void | SetVerbose (bool verbose) |
Enable/disable information terminal output during initialization (default: false). | |
virtual void | UnbindItem (std::shared_ptr< ChPhysicsItem > item) |
Remove the visual assets for the specified physics item from this visualization system. | |
virtual void | AddGrid (double x_step, double y_step, int nx, int ny, ChCoordsys<> pos=CSYSNORM, ChColor col=ChColor(0.1f, 0.1f, 0.1f)) |
Add a grid with specified parameters in the x-y plane of the given frame. More... | |
virtual ChVector3d | GetCameraPosition () const |
Get the location of the current (active) camera. | |
virtual ChVector3d | GetCameraTarget () const |
Get the target (look-at) point of the current (active) camera. | |
void | UpdateCamera (int id, const ChVector3d &pos, ChVector3d target) |
Update the location and/or target points of the specified camera. | |
void | UpdateCamera (const ChVector3d &pos, ChVector3d target) |
virtual int | AddVisualModel (std::shared_ptr< ChVisualModel > model, const ChFrame<> &frame) |
Add a visual model not associated with a physical item. More... | |
virtual int | AddVisualModel (std::shared_ptr< ChVisualShape > shape, const ChFrame<> &frame) |
Add a visual model not associated with a physical item. More... | |
virtual void | UpdateVisualModel (int id, const ChFrame<> &frame) |
Update the position of the specified visualization-only model. | |
virtual void | RenderFrame (const ChFrame<> &frame, double axis_length=1) |
Render the specified reference frame. | |
virtual void | RenderCOGFrames (double axis_length=1) |
Render COG frames for all bodies in the system. | |
std::vector< ChSystem * > | GetSystems () const |
Get the list of associated Chrono systems. | |
ChSystem & | GetSystem (int i) const |
Get the specified associated Chrono system. | |
virtual double | GetSimulationRTF () const |
Return the simulation real-time factor (simulation time / simulated time). More... | |
virtual double | GetSimulationTime () const |
Return the current simulated time. More... | |
unsigned int | GetNumBodies () const |
Get the number of bodies (across all visualized systems). More... | |
unsigned int | GetNumLinks () const |
Get the number of links (across all visualized systems). More... | |
unsigned int | GetNumMeshes () const |
Get the number of meshes (across all visualized systems). | |
unsigned int | GetNumShafts () const |
Get the number of shafts (across all visualized systems). | |
unsigned int | GetNumStates () const |
Get the number of coordinates at the velocity level (across all visualized systems). | |
unsigned int | GetNumConstraints () const |
Get the number of scalar constraints (across all visualized systems). | |
unsigned int | GetNumContacts () const |
Gets the number of contacts. | |
void | SetImageOutputDirectory (const std::string &dir) |
Set output directory for saving frame snapshots (default: "."). | |
void | SetImageOutput (bool val) |
Enable/disable writing of frame snapshots to file. | |
Static Public Member Functions | |
static void | WrapRenderStep (void *stepFunction) |
Callback wrapper for steps of the render loop. Works with Emscripten. | |
Friends | |
class | ChOpenGLViewer |
Additional Inherited Members | |
![]() | |
enum | Type { Type::IRRLICHT, Type::VSG, Type::OpenGL, Type::OptiX, NONE } |
Supported run-time visualization systems. More... | |
![]() | |
bool | m_verbose |
terminal output | |
bool | m_initialized |
std::vector< ChSystem * > | m_systems |
associated Chrono system(s) | |
bool | m_write_images |
if true, save snapshots | |
std::string | m_image_dir |
directory for image files | |
Member Function Documentation
◆ AttachStatsRenderer()
void chrono::opengl::ChVisualSystemOpenGL::AttachStatsRenderer | ( | std::shared_ptr< ChOpenGLStats > | renderer | ) |
Attach a user-defined text renderer for stats overlay.
This overwrites the default stats overlay renderer.
◆ AttachSystem()
|
overridevirtual |
Attach a Chrono system to the run-time visualization system.
ChVisualSystemOpenGL allows simultaneous rendering of multiple Chrono systems.
Reimplemented from chrono::ChVisualSystem.
◆ BindAll()
|
overridevirtual |
Process all visual assets in the associated ChSystem.
This function is called by default by Initialize(), but can also be called later if further modifications to visualization assets occur.
Reimplemented from chrono::ChVisualSystem.
◆ BindItem()
|
overridevirtual |
Process the visual assets for the spcified physics item.
This function must be called if a new physics item is added to the system or if changes to its visual model occur after the call to Initialize().
Reimplemented from chrono::ChVisualSystem.
◆ Initialize()
|
overridevirtual |
Initialize the visualization system.
This creates the Irrlicht device using the current values for the optional device parameters.
Implements chrono::ChVisualSystem.
Reimplemented in chrono::vehicle::ChVehicleVisualSystemOpenGL.
◆ Render()
|
overridevirtual |
Draw all 3D shapes and GUI elements at the current frame.
This function is typically called inside a loop such as
while(vis->Run()) {...}
Implements chrono::ChVisualSystem.
◆ Run()
|
overridevirtual |
Run the Irrlicht device.
Returns false
if the device wants to be deleted.
Implements chrono::ChVisualSystem.
◆ SetCameraProperties()
void chrono::opengl::ChVisualSystemOpenGL::SetCameraProperties | ( | float | scale = 0.5f , |
float | near_clip_dist = 0.1f , |
||
float | far_clip_dist = 1000.0f |
||
) |
Set camera scale and clip distances.
- Parameters
-
scale zoom level (default 0.5) near_clip_dist near clipping distance (default 0.1) far_clip_dist far clipping distance (default 1000)
◆ SetWindowSize()
void chrono::opengl::ChVisualSystemOpenGL::SetWindowSize | ( | unsigned int | width, |
unsigned int | height | ||
) |
Set the window size (default 640x480).
Must be called before Initialize().
◆ SetWindowTitle()
void chrono::opengl::ChVisualSystemOpenGL::SetWindowTitle | ( | const std::string & | win_title | ) |
Set the window title (default "").
Must be called before Initialize().
◆ WriteImageToFile()
|
overridevirtual |
Create a snapshot of the last rendered frame and save it to the provided file.
The file extension determines the image format.
Reimplemented from chrono::ChVisualSystem.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_opengl/ChVisualSystemOpenGL.h
- /builds/uwsbel/chrono/src/chrono_opengl/ChVisualSystemOpenGL.cpp