Description
Simple bond-based peridynamic material whose elasticity depends on a single parameter, that is K, the bulk modulus.
The Poisson ratio is always 1/4 and cannot be set otherwise, as in general for bond-based elasticity models. Having a fixed Poisson ration can be a limitation, but the positive note is that this material is very computationally-efficient. For very high stiffness, time integration might diverge because it does not introduce any tangent stiffness matrix (even if using implicit integrators like HHT, this will behave like in explicit anyway); use ChMatterPeriBBimplicit in these cases.
#include <ChMatterPeriBB.h>
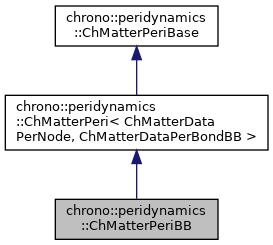
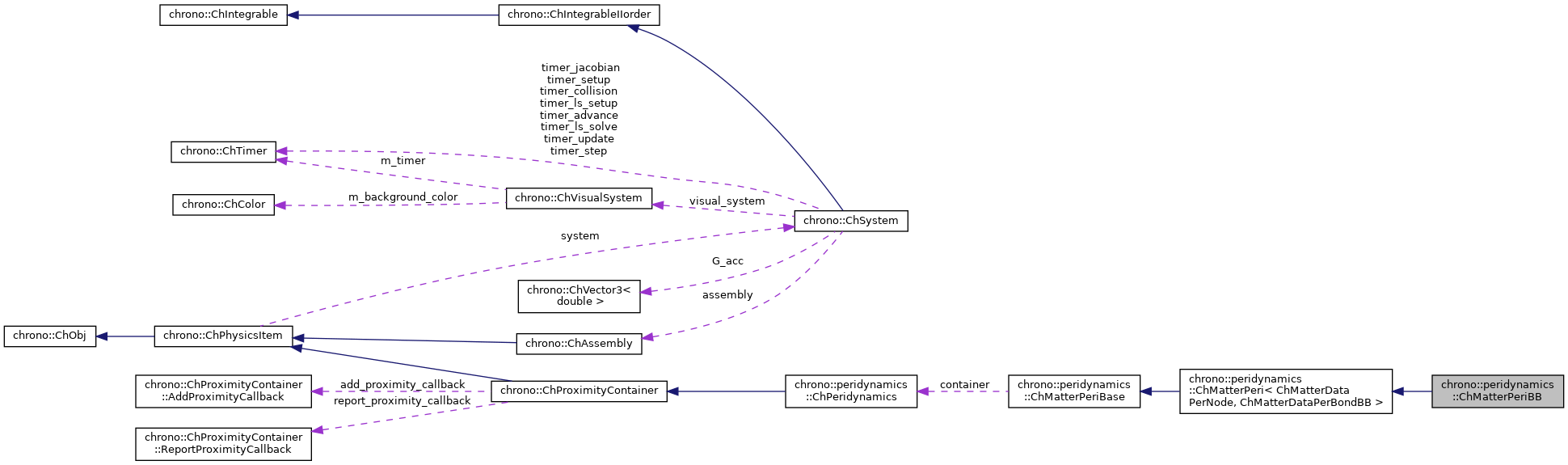
Public Member Functions | |
void | SetYoungModulus (double mE) |
Set the material Young modulus. More... | |
double | VolumeCorrection (double dist, double horizon, double vol_size) |
When doing quadrature, particle volumes that overlap with the horizon should be scaled proportionally to how much of their volume is really inside the horizon sphere. More... | |
virtual void | ComputeForces () |
CONSTITUTIVE MODEL - INTERFACE TO IMPLEMENT: IMPORTANT Add the forces caused by this material to the ChNodePeri::F vector of each ChNodePeri. More... | |
void | SetupInitial () override |
CONSTITUTIVE MODEL - INTERFACE TO IMPLEMENT: (optionally) This function is called where system construction is completed, at the beginning of the simulation. More... | |
![]() | |
ChMatterPeri () | |
Build a cluster of nodes for peridynamics. More... | |
ChMatterPeri (const ChMatterPeri &other) | |
unsigned int | GetNnodes () const |
Get the number of nodes. | |
unsigned int | GetNbonds () const |
Get the number of bonds. | |
const std::unordered_map< ChNodePeri *, ChMatterDataPerNode > & | GetMapOfNodes () |
Access the node container. | |
std::unordered_map< std::pair< ChNodePeri *, ChNodePeri * >, ChMatterDataPerBondBB > & | GetMapOfBonds () |
Access the bonds container. | |
virtual std::shared_ptr< ChNodePeri > | AddNode (std::shared_ptr< ChNodePeri > mnode) override |
Add a node to the particle cluster. | |
virtual bool | RemoveNode (std::shared_ptr< ChNodePeri > mnode) override |
Remove a node from the particle cluster. | |
virtual bool | AddProximity (ChNodePeri *nodeA, ChNodePeri *nodeB) override |
Add a proximity: this will be called thousand of times by the broadphase collision detection. More... | |
virtual void | ComputeForcesReset () override |
CONSTITUTIVE MODEL - INTERFACE TO IMPLEMENT: (optionally) Base behaviour: More... | |
virtual void | ComputeCollisionStateChanges () override |
CONSTITUTIVE MODEL - INTERFACE TO IMPLEMENT: (optionally) Changes the collision model of nodes, from collision to no collision, etc., ex when an interface is generated, depending on the evolution of the system. More... | |
void | SetContactMaterial (const std::shared_ptr< ChContactMaterial > &mnewsurf) |
Set the material surface for 'boundary contact'. | |
std::shared_ptr< ChContactMaterial > & | GetContactMaterial () |
Set the material surface for 'boundary contact'. | |
virtual void | ArchiveOut (ChArchiveOut &marchive) |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIn (ChArchiveIn &marchive) |
Method to allow de-serialization of transient data from archives. | |
![]() | |
virtual void | Setup () |
CONSTITUTIVE MODEL - INTERFACE TO IMPLEMENT: (optionally) This function is called at each time step. More... | |
virtual unsigned int | GetNumConstraints () |
Get the number of scalar constraints (maybe used for implicit materials) | |
virtual void | IntLoadResidual_CqL (const unsigned int off_L, ChVectorDynamic<> &R, const ChVectorDynamic<> &L, const double c) |
virtual void | IntLoadConstraint_C (const unsigned int off, ChVectorDynamic<> &Qc, const double c, bool do_clamp, double recovery_clamp) |
Takes the term C, scale and adds to Qc at given offset: Qc += c*C. More... | |
virtual void | InjectConstraints (ChSystemDescriptor &descriptor) |
Register with the given system descriptor any ChConstraint objects associated with this item. | |
virtual void | LoadConstraintJacobians () |
Compute and load current Jacobians in encapsulated ChConstraint objects. | |
virtual void | IntToDescriptor (const unsigned int off_v, const ChStateDelta &v, const ChVectorDynamic<> &R, const unsigned int off_L, const ChVectorDynamic<> &L, const ChVectorDynamic<> &Qc) |
virtual void | IntFromDescriptor (const unsigned int off_v, ChStateDelta &v, const unsigned int off_L, ChVectorDynamic<> &L) |
ChPeridynamics * | GetContainer () const |
void | SetContainer (ChPeridynamics *mc) |
Public Attributes | |
double | k_bulk = 100 |
bulk modulus, unit Pa, i.e. N/m^2 | |
double | damping = 0.001 |
damping, as Rayleigh beta (bulk stiffness-proportional) | |
double | max_stretch = 1e30 |
maximum stretch - after this, bonds will break. Default no break. | |
Additional Inherited Members | |
![]() | |
std::unordered_map< ChNodePeri *, ChMatterDataPerNode > | nodes |
nodes | |
std::unordered_map< std::pair< ChNodePeri *, ChNodePeri * >, ChMatterDataPerBondBB > | bonds |
bonds | |
std::shared_ptr< ChContactMaterial > | matsurface |
data for surface contact and impact | |
![]() | |
ChPeridynamics * | container = 0 |
Member Function Documentation
◆ ComputeForces()
|
inlinevirtual |
CONSTITUTIVE MODEL - INTERFACE TO IMPLEMENT: IMPORTANT Add the forces caused by this material to the ChNodePeri::F vector of each ChNodePeri.
Child class can use the containers this->nodes and this->bonds to compute F, assuming bonds have been updated with latest collision detection.
Implements chrono::peridynamics::ChMatterPeriBase.
◆ SetupInitial()
|
inlineoverridevirtual |
CONSTITUTIVE MODEL - INTERFACE TO IMPLEMENT: (optionally) This function is called where system construction is completed, at the beginning of the simulation.
Maybe your constitutive law has to initialize some state data, if so you can implement this function, otherwise leave as empty.
Reimplemented from chrono::peridynamics::ChMatterPeriBase.
◆ SetYoungModulus()
|
inline |
Set the material Young modulus.
The unique bulk modulus will be automatically computed, since in this material Poisson is always =1/4
◆ VolumeCorrection()
|
inline |
When doing quadrature, particle volumes that overlap with the horizon should be scaled proportionally to how much of their volume is really inside the horizon sphere.
A simple and very often used approximation is the following 'fading' function.
The documentation for this class was generated from the following file:
- /builds/uwsbel/chrono/src/chrono_peridynamics/ChMatterPeriBB.h