Description
The simplest peridynamic material: a bond-based material based on a network of springs, each with the same stiffness k regardless of length, etc.
Just for didactical purposes - do not use it for serious applications. Also use a damping coefficient r.
#include <ChMatterPeriSprings.h>
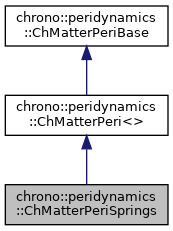
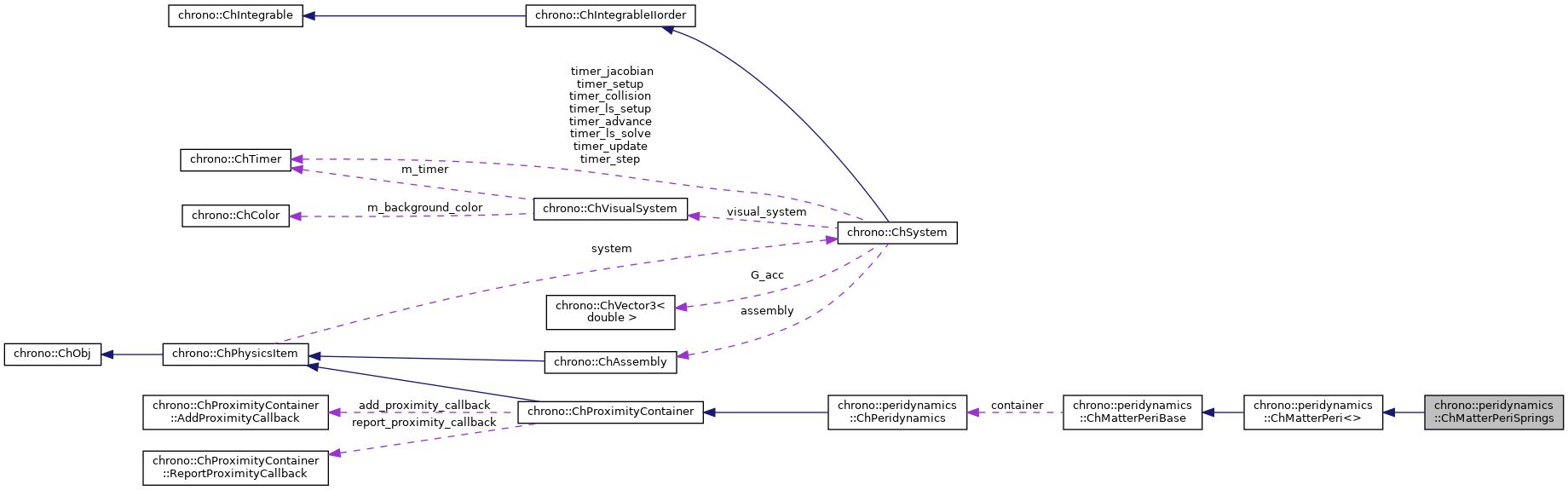
Public Member Functions | |
virtual void | ComputeForces () |
CONSTITUTIVE MODEL - INTERFACE TO IMPLEMENT: IMPORTANT Add the forces caused by this material to the ChNodePeri::F vector of each ChNodePeri. More... | |
![]() | |
ChMatterPeri () | |
Build a cluster of nodes for peridynamics. More... | |
ChMatterPeri (const ChMatterPeri &other) | |
unsigned int | GetNnodes () const |
Get the number of nodes. | |
unsigned int | GetNbonds () const |
Get the number of bonds. | |
const std::unordered_map< ChNodePeri *, ChMatterDataPerNode > & | GetMapOfNodes () |
Access the node container. | |
std::unordered_map< std::pair< ChNodePeri *, ChNodePeri * >, ChMatterDataPerBond > & | GetMapOfBonds () |
Access the bonds container. | |
virtual std::shared_ptr< ChNodePeri > | AddNode (std::shared_ptr< ChNodePeri > mnode) override |
Add a node to the particle cluster. | |
virtual bool | RemoveNode (std::shared_ptr< ChNodePeri > mnode) override |
Remove a node from the particle cluster. | |
virtual bool | AddProximity (ChNodePeri *nodeA, ChNodePeri *nodeB) override |
Add a proximity: this will be called thousand of times by the broadphase collision detection. More... | |
virtual void | ComputeForcesReset () override |
CONSTITUTIVE MODEL - INTERFACE TO IMPLEMENT: (optionally) Base behaviour: More... | |
virtual void | ComputeCollisionStateChanges () override |
CONSTITUTIVE MODEL - INTERFACE TO IMPLEMENT: (optionally) Changes the collision model of nodes, from collision to no collision, etc., ex when an interface is generated, depending on the evolution of the system. More... | |
void | SetContactMaterial (const std::shared_ptr< ChContactMaterial > &mnewsurf) |
Set the material surface for 'boundary contact'. | |
std::shared_ptr< ChContactMaterial > & | GetContactMaterial () |
Set the material surface for 'boundary contact'. | |
virtual void | ArchiveOut (ChArchiveOut &marchive) |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIn (ChArchiveIn &marchive) |
Method to allow de-serialization of transient data from archives. | |
![]() | |
virtual void | SetupInitial () |
CONSTITUTIVE MODEL - INTERFACE TO IMPLEMENT: (optionally) This function is called where system construction is completed, at the beginning of the simulation. More... | |
virtual void | Setup () |
CONSTITUTIVE MODEL - INTERFACE TO IMPLEMENT: (optionally) This function is called at each time step. More... | |
virtual unsigned int | GetNumConstraints () |
Get the number of scalar constraints (maybe used for implicit materials) | |
virtual void | IntLoadResidual_CqL (const unsigned int off_L, ChVectorDynamic<> &R, const ChVectorDynamic<> &L, const double c) |
virtual void | IntLoadConstraint_C (const unsigned int off, ChVectorDynamic<> &Qc, const double c, bool do_clamp, double recovery_clamp) |
Takes the term C, scale and adds to Qc at given offset: Qc += c*C. More... | |
virtual void | InjectConstraints (ChSystemDescriptor &descriptor) |
Register with the given system descriptor any ChConstraint objects associated with this item. | |
virtual void | LoadConstraintJacobians () |
Compute and load current Jacobians in encapsulated ChConstraint objects. | |
virtual void | IntToDescriptor (const unsigned int off_v, const ChStateDelta &v, const ChVectorDynamic<> &R, const unsigned int off_L, const ChVectorDynamic<> &L, const ChVectorDynamic<> &Qc) |
virtual void | IntFromDescriptor (const unsigned int off_v, ChStateDelta &v, const unsigned int off_L, ChVectorDynamic<> &L) |
ChPeridynamics * | GetContainer () const |
void | SetContainer (ChPeridynamics *mc) |
Public Attributes | |
double | k = 100 |
double | r = 10 |
Additional Inherited Members | |
![]() | |
std::unordered_map< ChNodePeri *, ChMatterDataPerNode > | nodes |
nodes | |
std::unordered_map< std::pair< ChNodePeri *, ChNodePeri * >, ChMatterDataPerBond > | bonds |
bonds | |
std::shared_ptr< ChContactMaterial > | matsurface |
data for surface contact and impact | |
![]() | |
ChPeridynamics * | container = 0 |
Member Function Documentation
◆ ComputeForces()
|
inlinevirtual |
CONSTITUTIVE MODEL - INTERFACE TO IMPLEMENT: IMPORTANT Add the forces caused by this material to the ChNodePeri::F vector of each ChNodePeri.
Child class can use the containers this->nodes and this->bonds to compute F, assuming bonds have been updated with latest collision detection.
Implements chrono::peridynamics::ChMatterPeriBase.
The documentation for this class was generated from the following file:
- /builds/uwsbel/chrono/src/chrono_peridynamics/ChMatterPeriSprings.h