Description
Base class for a chrono sensor. A specific sensor can inherit from here.
#include <ChSensor.h>
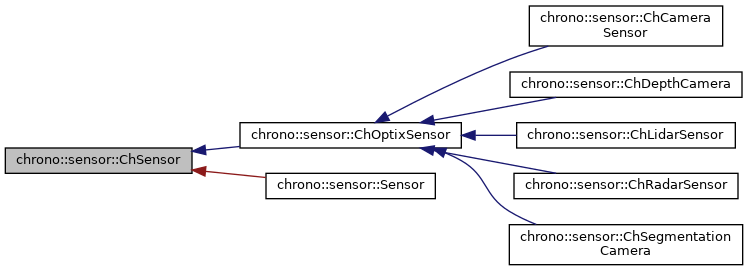
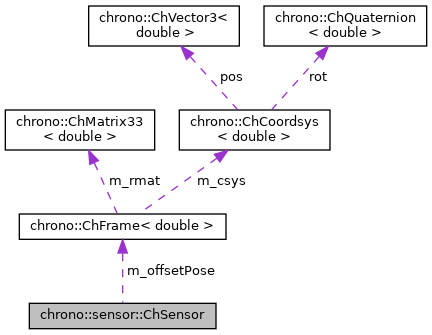
Public Member Functions | |
ChSensor (std::shared_ptr< chrono::ChBody > parent, float updateRate, chrono::ChFrame< double > offsetPose) | |
Constructor for the base sensor class. More... | |
virtual | ~ChSensor () |
Class destructor. | |
void | SetOffsetPose (chrono::ChFrame< double > pose) |
Set the sensor's relative position and orientation. More... | |
ChFrame< double > | GetOffsetPose () |
Get the sensor's relative position and orientation. More... | |
std::shared_ptr< ChBody > | GetParent () const |
Get the object to which the sensor is attached. More... | |
void | SetName (std::string name) |
Set the sensor's name. More... | |
std::string | GetName () const |
Get the name of the sensor. More... | |
float | GetUpdateRate () const |
Get the sensor update rate (Hz) More... | |
void | SetLag (float t) |
Set the lag parameter. More... | |
float | GetLag () const |
Get the sensor lag (seconds) More... | |
void | SetCollectionWindow (float t) |
Set the collection window. More... | |
float | GetCollectionWindow () const |
Get the sensor data collection window (seconds) More... | |
void | SetUpdateRate (float updateRate) |
Set the sensor update rate (Hz) More... | |
unsigned int | GetNumLaunches () |
Get the number of times the sensor has been updated. More... | |
void | IncrementNumLaunches () |
Increments the count of number of updates. | |
std::list< std::shared_ptr< ChFilter > > | GetFilterList () const |
Get the sensor's list of filters. More... | |
void | PushFilter (std::shared_ptr< ChFilter > filter) |
Add a filter to the sensor. More... | |
void | PushFilterFront (std::shared_ptr< ChFilter > filter) |
Add a filter to the front of the list on a sensor. More... | |
void | LockFilterList () |
Gives ability to lock the filter list to prevent race conditions. More... | |
template<class UserBufferType > | |
UserBufferType | GetMostRecentBuffer () |
Get the last filter in the list that matches the template type. More... | |
template<> | |
CH_SENSOR_API UserR8BufferPtr | GetMostRecentBuffer () |
template<> | |
CH_SENSOR_API UserRGBA8BufferPtr | GetMostRecentBuffer () |
template<> | |
CH_SENSOR_API UserDepthBufferPtr | GetMostRecentBuffer () |
template<> | |
CH_SENSOR_API UserDIBufferPtr | GetMostRecentBuffer () |
template<> | |
CH_SENSOR_API UserXYZIBufferPtr | GetMostRecentBuffer () |
template<> | |
CH_SENSOR_API UserRadarBufferPtr | GetMostRecentBuffer () |
template<> | |
CH_SENSOR_API UserRadarXYZBufferPtr | GetMostRecentBuffer () |
template<> | |
CH_SENSOR_API UserAccelBufferPtr | GetMostRecentBuffer () |
template<> | |
CH_SENSOR_API UserGyroBufferPtr | GetMostRecentBuffer () |
template<> | |
CH_SENSOR_API UserMagnetBufferPtr | GetMostRecentBuffer () |
template<> | |
CH_SENSOR_API UserGPSBufferPtr | GetMostRecentBuffer () |
template<> | |
CH_SENSOR_API UserTachometerBufferPtr | GetMostRecentBuffer () |
Protected Attributes | |
float | m_updateRate |
sensor update rate | |
float | m_lag |
sensor lag from the time all scene information is available (sensor processing time) | |
float | m_collection_window |
time over which data is collected. More... | |
float | m_timeLastUpdated |
time since previous update | |
std::shared_ptr< chrono::ChBody > | m_parent |
object to which the sensor is attached | |
chrono::ChFrame< double > | m_offsetPose |
position and orientation of the sensor relative to its parent | |
std::string | m_name |
name of the sensor | |
unsigned int | m_num_launches |
number of times the sensor has been updated | |
std::list< std::shared_ptr< ChFilter > > | m_filters |
filter list for post-processing sensor data | |
bool | m_filter_list_locked = false |
gives ability to lock the filter list to prevent race conditions | |
Constructor & Destructor Documentation
◆ ChSensor()
CH_SENSOR_API chrono::sensor::ChSensor::ChSensor | ( | std::shared_ptr< chrono::ChBody > | parent, |
float | updateRate, | ||
chrono::ChFrame< double > | offsetPose | ||
) |
Constructor for the base sensor class.
- Parameters
-
parent Body to which the sensor is attached. updateRate Rate at which the sensor should update. offsetPose Relative position and orientation of the sensor with respect to its parent object.
Member Function Documentation
◆ GetCollectionWindow()
|
inline |
Get the sensor data collection window (seconds)
- Returns
- The duration of simulation time over which the sensor generates its data
◆ GetFilterList()
|
inline |
Get the sensor's list of filters.
- Returns
- An immutable list of filters that are used to generate and augment the sensor's data
◆ GetLag()
|
inline |
Get the sensor lag (seconds)
- Returns
- The lag of the sensor
◆ GetMostRecentBuffer()
UserBufferType chrono::sensor::ChSensor::GetMostRecentBuffer | ( | ) |
Get the last filter in the list that matches the template type.
- Returns
- A shared pointer to a ChSensorBuffer of the templated type.
◆ GetName()
|
inline |
Get the name of the sensor.
- Returns
- The string name of the sensor
◆ GetNumLaunches()
|
inline |
Get the number of times the sensor has been updated.
- Returns
- The number of times an update for the sensor has been started
◆ GetOffsetPose()
|
inline |
Get the sensor's relative position and orientation.
- Returns
- The frame that specifies the offset pose
◆ GetParent()
|
inline |
Get the object to which the sensor is attached.
- Returns
- A shared pointer to the body on which the sensor is attached
◆ GetUpdateRate()
|
inline |
Get the sensor update rate (Hz)
- Returns
- The update rate in Hz
◆ LockFilterList()
|
inline |
Gives ability to lock the filter list to prevent race conditions.
This is called automatically when the sensor is added to the ChSensor manager. This is needed to prevent changing of filters while a worker thread is processing the filters. WARNING: this operation cannot be undone.
◆ PushFilter()
CH_SENSOR_API void chrono::sensor::ChSensor::PushFilter | ( | std::shared_ptr< ChFilter > | filter | ) |
Add a filter to the sensor.
- Parameters
-
filter A filter that should be added to the filter list if the filter list is not yet locked. If the filter list has been locked (i.e. the sensor has started generating data) the new filter will be ignored.
◆ PushFilterFront()
CH_SENSOR_API void chrono::sensor::ChSensor::PushFilterFront | ( | std::shared_ptr< ChFilter > | filter | ) |
Add a filter to the front of the list on a sensor.
- Parameters
-
filter A filter that should be added to the filter list if the filter list is not yet locked. If the filter list has been locked (i.e. the sensor has started generating data) the new filter will be ignored.
◆ SetCollectionWindow()
CH_SENSOR_API void chrono::sensor::ChSensor::SetCollectionWindow | ( | float | t | ) |
Set the collection window.
- Parameters
-
t The collection time of the sensor
◆ SetLag()
CH_SENSOR_API void chrono::sensor::ChSensor::SetLag | ( | float | t | ) |
Set the lag parameter.
- Parameters
-
t The lag time
◆ SetName()
|
inline |
Set the sensor's name.
- Parameters
-
name Name of the sensor -> not used for any internal critical mechanisms
◆ SetOffsetPose()
|
inline |
Set the sensor's relative position and orientation.
- Parameters
-
pose The relative position and orientation with respect to the parent body
◆ SetUpdateRate()
|
inline |
Set the sensor update rate (Hz)
- Parameters
-
updateRate Desired update rate in Hz
Member Data Documentation
◆ m_collection_window
|
protected |
time over which data is collected.
(lag+shutter = time from when data collection is started to when it is available to the user)
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_sensor/sensors/ChSensor.h
- /builds/uwsbel/chrono/src/chrono_sensor/sensors/ChSensor.cpp