Description
Rigid terrain model.
This class implements a terrain modeled as a rigid shape which can interact through contact and friction with any other bodies whose contact flag is enabled. In particular, this type of terrain can be used in conjunction with a ChRigidTire.
#include <RigidTerrain.h>
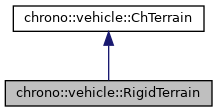
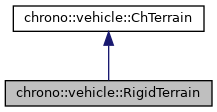
Classes | |
class | Patch |
Definition of a patch in a rigid terrain model. More... | |
Public Types | |
enum | PatchType { PatchType::BOX, PatchType::MESH, PatchType::HEIGHT_MAP } |
Patch type. More... | |
Public Member Functions | |
RigidTerrain (ChSystem *system) | |
Construct a default RigidTerrain. More... | |
RigidTerrain (ChSystem *system, const std::string &filename) | |
Construct a RigidTerrain from a JSON specification file. More... | |
std::shared_ptr< Patch > | AddPatch (std::shared_ptr< ChContactMaterial > material, const ChCoordsys<> &position, double length, double width, double thickness=1, bool tiled=false, double max_tile_size=1, bool visualization=true) |
Add a terrain patch represented by a rigid box. More... | |
std::shared_ptr< Patch > | AddPatch (std::shared_ptr< ChContactMaterial > material, const ChCoordsys<> &position, const std::string &mesh_file, bool connected_mesh=true, double sweep_sphere_radius=0, bool visualization=true) |
Add a terrain patch represented by a triangular mesh. More... | |
std::shared_ptr< Patch > | AddPatch (std::shared_ptr< ChContactMaterial > material, const ChCoordsys<> &position, const std::string &heightmap_file, double length, double width, double hMin, double hMax, bool connected_mesh=true, double sweep_sphere_radius=0, bool visualization=true) |
Add a terrain patch represented by a heightmap image. More... | |
std::shared_ptr< Patch > | AddPatch (std::shared_ptr< ChContactMaterial > material, const ChCoordsys<> &position, const std::vector< ChVector3d > &point_cloud, double length, double width, int unrefined_resolution, int heightmap_resolution, int max_refinements=5, double refine_angle_limit=30, double smoothing_factor=0.25, double max_edge_length=1.0, double sweep_sphere_radius=0.001, bool visualization=true) |
Add a terrain patch drawn from a vector of vectors - refined using the LEPP method. More... | |
void | Initialize () |
Initialize all defined terrain patches. | |
const std::vector< std::shared_ptr< Patch > > & | GetPatches () const |
Get the terrain patches currently added to the rigid terrain system. | |
void | BindPatch (std::shared_ptr< Patch > patch) |
Bind the visual and collision models of the specified patch. More... | |
void | RemovePatch (std::shared_ptr< Patch > patch) |
Remove the specified patch. | |
void | UseLocationDependentFriction (bool val) |
Enable use of location-dependent coefficient of friction in terrain-solid contacts. More... | |
virtual double | GetHeight (const ChVector3d &loc) const override |
Get the terrain height below the specified location. More... | |
virtual ChVector3d | GetNormal (const ChVector3d &loc) const override |
Get the terrain normal at the point below the specified location. More... | |
virtual float | GetCoefficientFriction (const ChVector3d &loc) const override |
Get the terrain coefficient of friction at the point below the specified location. More... | |
virtual void | GetProperties (const ChVector3d &loc, double &height, ChVector3d &normal, float &friction) const override |
Get all terrain characteristics at the point below the specified location. More... | |
void | ExportMeshPovray (const std::string &out_dir, bool smoothed=false) |
Export all patch meshes as macros in PovRay include files. | |
void | ExportMeshWavefront (const std::string &out_dir) |
Export all patch meshes as Wavefront files. | |
bool | FindPoint (const ChVector3d loc, double &height, ChVector3d &normal, float &friction) const |
Find the terrain height, normal, and coefficient of friction at the point below the specified location. More... | |
void | SetCollisionFamily (int family) |
Set common collision family for patches. More... | |
![]() | |
virtual void | Synchronize (double time) |
Update the state of the terrain system at the specified time. | |
virtual void | Advance (double step) |
Advance the state of the terrain system by the specified duration. | |
void | RegisterHeightFunctor (std::shared_ptr< HeightFunctor > functor) |
Specify the functor object to provide the terrain height at given locations. More... | |
void | RegisterNormalFunctor (std::shared_ptr< NormalFunctor > functor) |
Specify the functor object to provide the terrain normal at given locations. More... | |
void | RegisterFrictionFunctor (std::shared_ptr< FrictionFunctor > functor) |
Specify the functor object to provide the coefficient of friction at given locations. | |
Additional Inherited Members | |
![]() | |
std::shared_ptr< HeightFunctor > | m_height_fun |
functor for location-dependent terrain height | |
std::shared_ptr< NormalFunctor > | m_normal_fun |
functor for location-dependent terrain normal | |
std::shared_ptr< FrictionFunctor > | m_friction_fun |
functor for location-dependent coefficient of friction | |
Member Enumeration Documentation
◆ PatchType
|
strong |
Patch type.
Enumerator | |
---|---|
BOX | rectangular box |
MESH | triangular mesh (from a Wavefront OBJ file) |
HEIGHT_MAP | triangular mesh (generated from a gray-scale heightmap image) |
Constructor & Destructor Documentation
◆ RigidTerrain() [1/2]
chrono::vehicle::RigidTerrain::RigidTerrain | ( | ChSystem * | system | ) |
Construct a default RigidTerrain.
The user is responsible for calling various Set methods before Initialize.
- Parameters
-
[in] system pointer to the containing multibody system
◆ RigidTerrain() [2/2]
chrono::vehicle::RigidTerrain::RigidTerrain | ( | ChSystem * | system, |
const std::string & | filename | ||
) |
Construct a RigidTerrain from a JSON specification file.
- Parameters
-
[in] system pointer to the containing multibody system [in] filename name of the JSON specification file
Member Function Documentation
◆ AddPatch() [1/4]
std::shared_ptr< RigidTerrain::Patch > chrono::vehicle::RigidTerrain::AddPatch | ( | std::shared_ptr< ChContactMaterial > | material, |
const ChCoordsys<> & | position, | ||
const std::string & | heightmap_file, | ||
double | length, | ||
double | width, | ||
double | hMin, | ||
double | hMax, | ||
bool | connected_mesh = true , |
||
double | sweep_sphere_radius = 0 , |
||
bool | visualization = true |
||
) |
Add a terrain patch represented by a heightmap image.
If the image has an explicit gray channel, that value is used as an encoding of height. Otherwise, RGB values are converted to YUV and luminance (Y) is used as height encoding.
- Parameters
-
[in] material contact material [in] position patch location and orientation [in] heightmap_file filename for the height map (BMP) [in] length patch length [in] width patch width [in] hMin minimum height (black level) [in] hMax maximum height (white level) [in] connected_mesh use connected contact mesh? [in] sweep_sphere_radius radius of sweep sphere [in] visualization enable/disable construction of visualization assets
◆ AddPatch() [2/4]
std::shared_ptr< RigidTerrain::Patch > chrono::vehicle::RigidTerrain::AddPatch | ( | std::shared_ptr< ChContactMaterial > | material, |
const ChCoordsys<> & | position, | ||
const std::string & | mesh_file, | ||
bool | connected_mesh = true , |
||
double | sweep_sphere_radius = 0 , |
||
bool | visualization = true |
||
) |
Add a terrain patch represented by a triangular mesh.
The mesh is specified through a Wavefront file and is used for both contact and visualization.
- Parameters
-
[in] material contact material [in] position patch location and orientation [in] mesh_file filename of the input mesh (OBJ) [in] connected_mesh use connected contact mesh? [in] sweep_sphere_radius radius of sweep sphere [in] visualization enable/disable construction of visualization assets
◆ AddPatch() [3/4]
std::shared_ptr< RigidTerrain::Patch > chrono::vehicle::RigidTerrain::AddPatch | ( | std::shared_ptr< ChContactMaterial > | material, |
const ChCoordsys<> & | position, | ||
const std::vector< ChVector3d > & | point_cloud, | ||
double | length, | ||
double | width, | ||
int | unrefined_resolution, | ||
int | heightmap_resolution, | ||
int | max_refinements = 5 , |
||
double | refine_angle_limit = 30 , |
||
double | smoothing_factor = 0.25 , |
||
double | max_edge_length = 1.0 , |
||
double | sweep_sphere_radius = 0.001 , |
||
bool | visualization = true |
||
) |
Add a terrain patch drawn from a vector of vectors - refined using the LEPP method.
For each Chvector, x and y represent grid elements and the z value is the height. Usually, 10% to 25% of the heightmap resolution for unrefined_resolution produces good results.
- Parameters
-
[in] material contact material [in] position patch location and orientation [in] point_cloud point cloud of height vectors [in] length patch length (m) [in] width patch width (m) [in] unrefined_resolution the starting resolution of the alternating triangle mesh [in] heightmap_resolution resolution of the heightmap to filter the point cloud [in] max_refinements maximum triangle edge length for use in edge refinement [in] refine_angle_limit Normal threshold angle for refinement stop (degrees) [in] smoothing_factor Taubin smoothing degree [0,1] [in] max_edge_length Maximum edge length in Refine Mesh Edges LEPP method [in] sweep_sphere_radius radius of sweep sphere [in] visualization enable/disable construction of visualisation assets
◆ AddPatch() [4/4]
std::shared_ptr< RigidTerrain::Patch > chrono::vehicle::RigidTerrain::AddPatch | ( | std::shared_ptr< ChContactMaterial > | material, |
const ChCoordsys<> & | position, | ||
double | length, | ||
double | width, | ||
double | thickness = 1 , |
||
bool | tiled = false , |
||
double | max_tile_size = 1 , |
||
bool | visualization = true |
||
) |
Add a terrain patch represented by a rigid box.
The patch is constructed such that the center of its top surface (the "driving" surface) is in the x-y plane of the specified coordinate system. If tiled = true, multiple side-by-side boxes are used.
- Parameters
-
[in] material contact material [in] position patch location and orientation [in] length patch length [in] width patch width [in] thickness box thickness [in] tiled terrain created from multiple tiled boxes [in] max_tile_size maximum tile size [in] visualization enable/disable construction of visualization assets
◆ BindPatch()
void chrono::vehicle::RigidTerrain::BindPatch | ( | std::shared_ptr< Patch > | patch | ) |
Bind the visual and collision models of the specified patch.
This function should be called for any patches created dynamically during simulation, after the RigidTerrain was initialized.
◆ FindPoint()
bool chrono::vehicle::RigidTerrain::FindPoint | ( | const ChVector3d | loc, |
double & | height, | ||
ChVector3d & | normal, | ||
float & | friction | ||
) | const |
Find the terrain height, normal, and coefficient of friction at the point below the specified location.
The point on the terrain surface is obtained through ray casting into the terrain contact model. The return value is 'true' if the ray intersection succeeded and 'false' otherwise (in which case the output is set to heigh=0, normal=world vertical, and friction=0.8).
◆ GetCoefficientFriction()
|
overridevirtual |
Get the terrain coefficient of friction at the point below the specified location.
This function should return the coefficient of friction at the closest point below the specified location (in the direction of the current world vertical). For RigidTerrain, this function defers to the user-provided functor object of type ChTerrain::FrictionFunctor, if one was specified. Otherwise, it returns the constant value from the appropriate patch, as specified through SetContactFrictionCoefficient. Note that this function may be used by tire models to appropriately modify the tire characteristics, but it will have no effect on the interaction of the terrain with other objects (including tire models that do not explicitly use it). See UseLocationDependentFriction.
Reimplemented from chrono::vehicle::ChTerrain.
◆ GetHeight()
|
overridevirtual |
Get the terrain height below the specified location.
This function should return the height of the closest point below the specified location (in the direction of the current world vertical). If a user-provided functor object of type ChTerrain::HeightFunctor is provided, that will take precedence over the internal mechanism for calculating terrain height based on the specified geometry.
Reimplemented from chrono::vehicle::ChTerrain.
◆ GetNormal()
|
overridevirtual |
Get the terrain normal at the point below the specified location.
This function should return the normal at the closest point below the specified location (in the direction of the current world vertical). If a user-provided functor object of type ChTerrain::NormalFunctor is provided, that will take precedence over the internal mechanism for calculating terrain normal based on the specified geometry.
Reimplemented from chrono::vehicle::ChTerrain.
◆ GetProperties()
|
overridevirtual |
Get all terrain characteristics at the point below the specified location.
This function should return the terrain properties at the closest point below the specified location (in the direction of the current world vertical). This is more efficient than calling GetHeight, GetNormal, and GetCoefficientFriction separately, as it performs a single ray-casting operation (if needed at all).
Reimplemented from chrono::vehicle::ChTerrain.
◆ SetCollisionFamily()
|
inline |
Set common collision family for patches.
Default: 14. Collision is disabled with all other objects in this family.
◆ UseLocationDependentFriction()
|
inline |
Enable use of location-dependent coefficient of friction in terrain-solid contacts.
This assumes that a non-trivial functor (of type ChTerrain::FrictionFunctor) was defined and registered with the terrain subsystem. Enable this only if simulating a system that has contacts with the terrain that must be resolved using the underlying Chrono contact mechanism (e.g., for rigid tires, FEA tires, tracked vehicles). Note that this feature requires a relatively expensive traversal of all contacts at each simulation step. By default, this option is disabled. This function must be called before Initialize.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_vehicle/terrain/RigidTerrain.h
- /builds/uwsbel/chrono/src/chrono_vehicle/terrain/RigidTerrain.cpp