Description
Tracked vehicle continuous-band sprocket model constructed with data from file (JSON format).
#include <SprocketBand.h>
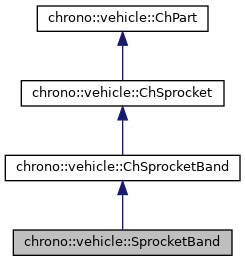
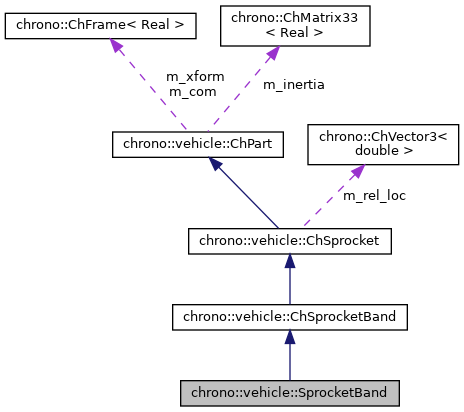
Public Member Functions | |
SprocketBand (const std::string &filename) | |
SprocketBand (const rapidjson::Document &d) | |
virtual unsigned int | GetNumTeeth () const override |
Get the number of teeth of the gear. | |
virtual double | GetAssemblyRadius () const override |
Get the radius of the gear. More... | |
virtual double | GetAddendumRadius () const override |
Get the addendum radius. More... | |
virtual double | GetGearMass () const override |
Return the mass of the gear body. | |
virtual const ChVector3d & | GetGearInertia () override |
Return the moments of inertia of the gear body. | |
virtual double | GetAxleInertia () const override |
Return the inertia of the axle shaft. | |
virtual double | GetSeparation () const override |
Return the distance between the two gear profiles. | |
virtual double | GetOuterRadius () const override |
Return the radius of the addendum circle. | |
virtual double | GetBaseWidth () const override |
Return the base width of the sprocket profile length of the chord where the tooth profile meets the sprocket's outer radius. | |
virtual double | GetTipWidth () const override |
Return the width of the inner tip of the sprocket profile. | |
virtual double | GetToothDepth () const override |
Return the depth of the sprocket profile. More... | |
virtual double | GetArcRadius () const override |
Return the radius of the (concave) tooth circular arc. | |
virtual double | GetLateralBacklash () const override |
Return the allowed backlash (play) before lateral contact with track shoes is enabled (to prevent detracking). | |
![]() | |
ChSprocketBand (const std::string &name) | |
virtual std::string | GetTemplateName () const override |
Get the name of the vehicle subsystem template. | |
virtual std::shared_ptr< ChLinePath > | GetProfile () const override |
Return the 2D gear profile. More... | |
virtual std::shared_ptr< ChSystem::CustomCollisionCallback > | GetCollisionCallback (ChTrackAssembly *track) override |
Return the custom collision callback object. More... | |
![]() | |
std::shared_ptr< ChBody > | GetGearBody () const |
Get a handle to the gear body. | |
std::shared_ptr< ChShaft > | GetAxle () const |
Get a handle to the axle shaft. | |
std::shared_ptr< ChLinkLockRevolute > | GetRevolute () const |
Get a handle to the revolute joint. | |
double | GetAxleSpeed () const |
Get the angular speed of the axle. | |
void | EnableCollision (bool val) |
Turn on/off collision flag for the gear wheel. | |
std::shared_ptr< ChContactMaterial > | GetContactMaterial () const |
Get the sprocket contact material. | |
void | DisableLateralContact () |
Disable lateral contact for preventing detracking (default: enabled). | |
void | Initialize (std::shared_ptr< ChChassis > chassis, const ChVector3d &location, ChTrackAssembly *track) |
Initialize this sprocket subsystem. More... | |
void | ApplyAxleTorque (double torque) |
Apply the provided torque to the sprocket's axle (for debugging and testing). More... | |
std::shared_ptr< ChTriangleMeshConnected > | CreateVisualizationMesh (double radius, double width, double delta, ChColor color=ChColor(1, 1, 1)) const |
Utility function to create a sprocket visualization mesh. More... | |
virtual void | RemoveVisualizationAssets () override |
Remove visualization assets for the sprocket subsystem. | |
void | LogConstraintViolations () |
Log current constraint violations. | |
![]() | |
const std::string & | GetName () const |
Get the name identifier for this subsystem. | |
void | SetName (const std::string &name) |
Set the name identifier for this subsystem. | |
bool | IsInitialized () const |
Return flag indicating whether or not the part is fully constructed. | |
virtual uint16_t | GetVehicleTag () const |
Get the tag of the associated vehicle. More... | |
int | GetBodyTag () const |
Get the tag for component bodies. | |
double | GetMass () const |
Get the subsystem mass. More... | |
const ChFrame & | GetCOMFrame () const |
Get the current subsystem COM frame (relative to and expressed in the subsystem's reference frame). More... | |
const ChMatrix33 & | GetInertia () const |
Get the current subsystem inertia (relative to the subsystem COM frame). More... | |
const ChFrame & | GetTransform () const |
Get the current subsystem position relative to the global frame. More... | |
void | SetVisualizationType (VisualizationType vis) |
Set the visualization mode for this subsystem. | |
virtual void | SetOutput (bool state) |
Enable/disable output for this subsystem. | |
bool | OutputEnabled () const |
Return the output state for this subsystem. | |
Additional Inherited Members | |
![]() | |
static ChMatrix33 | TransformInertiaMatrix (const ChVector3d &moments, const ChVector3d &products, const ChMatrix33<> &vehicle_rot, const ChMatrix33<> &body_rot) |
Utility function for transforming inertia tensors between centroidal frames. More... | |
![]() | |
ChSprocket (const std::string &name) | |
Construct a sprocket subsystem with given name. | |
virtual void | InitializeInertiaProperties () override |
Initialize subsystem inertia properties. More... | |
virtual void | UpdateInertiaProperties () override |
Update subsystem inertia properties. More... | |
virtual void | ExportComponentList (rapidjson::Document &jsonDocument) const override |
Export this subsystem's component list to the specified JSON object. | |
virtual void | Output (ChVehicleOutput &database) const override |
Output data for this subsystem's component list to the specified database. | |
![]() | |
ChPart (const std::string &name) | |
Construct a vehicle subsystem with the specified name. | |
void | AddMass (double &mass) |
Add this subsystem's mass. More... | |
void | AddInertiaProperties (ChVector3d &com, ChMatrix33<> &inertia) |
Add this subsystem's inertia properties. More... | |
void | ExportBodyList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChBody >> bodies) const |
Export the list of bodies to the specified JSON document. | |
void | ExportShaftList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaft >> shafts) const |
Export the list of shafts to the specified JSON document. | |
void | ExportJointList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLink >> joints) const |
Export the list of joints to the specified JSON document. | |
void | ExportCouplesList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaftsCouple >> couples) const |
Export the list of shaft couples to the specified JSON document. | |
void | ExportMarkerList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChMarker >> markers) const |
Export the list of markers to the specified JSON document. | |
void | ExportLinSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkTSDA >> springs) const |
Export the list of translational springs to the specified JSON document. | |
void | ExportRotSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkRSDA >> springs) const |
Export the list of rotational springs to the specified JSON document. | |
void | ExportBodyLoadList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLoadBodyBody >> loads) const |
Export the list of body-body loads to the specified JSON document. | |
![]() | |
static void | RemoveVisualizationAssets (std::shared_ptr< ChPhysicsItem > item) |
Erase all visual shapes from the visual model associated with the specified physics item (if any). | |
static void | RemoveVisualizationAsset (std::shared_ptr< ChPhysicsItem > item, std::shared_ptr< ChVisualShape > shape) |
Erase the given shape from the visual model associated with the specified physics item (if any). | |
![]() | |
ChVector3d | m_rel_loc |
sprocket subsystem location relative to chassis | |
std::shared_ptr< ChBody > | m_gear |
sprocket gear body | |
std::shared_ptr< ChShaft > | m_axle |
gear shafts | |
std::shared_ptr< ChShaftBodyRotation > | m_axle_to_spindle |
gear-shaft connector | |
std::shared_ptr< ChLinkLockRevolute > | m_revolute |
sprocket revolute joint | |
std::shared_ptr< ChContactMaterial > | m_material |
contact material; | |
std::shared_ptr< ChSystem::CustomCollisionCallback > | m_callback |
cached collision callback | |
bool | m_lateral_contact |
if 'true', enable lateral conatact to prevent detracking | |
![]() | |
std::string | m_name |
subsystem name | |
bool | m_initialized |
specifies whether ot not the part is fully constructed | |
bool | m_output |
specifies whether or not output is generated for this subsystem | |
std::shared_ptr< ChPart > | m_parent |
parent subsystem (empty if parent is vehicle) | |
double | m_mass |
subsystem mass | |
ChMatrix33 | m_inertia |
inertia tensor (relative to subsystem COM) | |
ChFrame | m_com |
COM frame (relative to subsystem reference frame) | |
ChFrame | m_xform |
subsystem frame expressed in the global frame | |
int | m_obj_tag |
tag for part objects | |
Member Function Documentation
◆ GetAddendumRadius()
|
inlineoverridevirtual |
Get the addendum radius.
This quantity is an average radius for sprocket-track engagement used to estimate longitudinal slip.
Implements chrono::vehicle::ChSprocket.
◆ GetAssemblyRadius()
|
inlineoverridevirtual |
Get the radius of the gear.
This quantity is used during the automatic track assembly.
Implements chrono::vehicle::ChSprocket.
◆ GetToothDepth()
|
inlineoverridevirtual |
Return the depth of the sprocket profile.
This is measured as the distance from the center of the profile tip line to the center of the base width line.
Implements chrono::vehicle::ChSprocketBand.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_vehicle/tracked_vehicle/sprocket/SprocketBand.h
- /builds/uwsbel/chrono/src/chrono_vehicle/tracked_vehicle/sprocket/SprocketBand.cpp