Description
Camera class.
#include <ChCameraSensor.h>
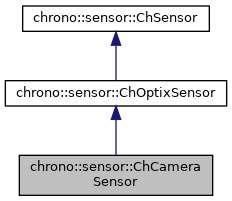
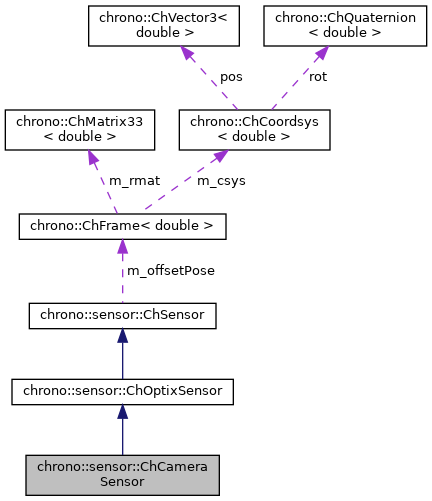
Public Member Functions | |
ChCameraSensor (std::shared_ptr< chrono::ChBody > parent, float updateRate, chrono::ChFrame< double > offsetPose, unsigned int w, unsigned int h, float hFOV, unsigned int supersample_factor=1, CameraLensModelType lens_model=CameraLensModelType::PINHOLE, bool use_gi=false, float gamma=2.2, bool use_fog=true) | |
Constructor for the base camera class that defaults to a pinhole lens model. More... | |
~ChCameraSensor () | |
camera class destructor | |
float | GetHFOV () const |
returns the camera's horizontal field of view. More... | |
CameraLensModelType | GetLensModelType () const |
returns the lens model type used for rendering More... | |
LensParams | GetLensParameters () const |
returns the lens model parameters More... | |
void | SetRadialLensParameters (ChVector3f params) |
@briefSets the parameters for a radial lens distortion model. More... | |
bool | GetUseGI () |
returns if the cemera requesting global illumination More... | |
float | GetGamma () |
returns the gamma correction value of this camera. More... | |
unsigned int | GetSampleFactor () |
Gets the number of samples per pixels in each direction used for super sampling. More... | |
bool | GetUseFog () |
returns if the cemera should use fog as dictated by the scene More... | |
ChMatrix33< float > | GetCameraIntrinsicMatrix () |
returns the 3x3 Intrinsic Matrix(K) of the camera More... | |
ChVector3f | GetCameraDistortionCoefficients () |
returns the camera distortion coefficients k1, k2, k3 More... | |
![]() | |
ChOptixSensor (std::shared_ptr< chrono::ChBody > parent, float updateRate, chrono::ChFrame< double > offsetPose, unsigned int w, unsigned int h) | |
Constructor for the base camera class that defaults to a pinhole lens model. More... | |
virtual | ~ChOptixSensor () |
camera class destructor | |
PipelineType | GetPipelineType () |
unsigned int | GetWidth () |
unsigned int | GetHeight () |
CUstream | GetCudaStream () |
![]() | |
ChSensor (std::shared_ptr< chrono::ChBody > parent, float updateRate, chrono::ChFrame< double > offsetPose) | |
Constructor for the base sensor class. More... | |
virtual | ~ChSensor () |
Class destructor. | |
void | SetOffsetPose (chrono::ChFrame< double > pose) |
Set the sensor's relative position and orientation. More... | |
ChFrame< double > | GetOffsetPose () |
Get the sensor's relative position and orientation. More... | |
std::shared_ptr< ChBody > | GetParent () const |
Get the object to which the sensor is attached. More... | |
void | SetName (std::string name) |
Set the sensor's name. More... | |
std::string | GetName () const |
Get the name of the sensor. More... | |
float | GetUpdateRate () const |
Get the sensor update rate (Hz) More... | |
void | SetLag (float t) |
Set the lag parameter. More... | |
float | GetLag () const |
Get the sensor lag (seconds) More... | |
void | SetCollectionWindow (float t) |
Set the collection window. More... | |
float | GetCollectionWindow () const |
Get the sensor data collection window (seconds) More... | |
void | SetUpdateRate (float updateRate) |
Set the sensor update rate (Hz) More... | |
unsigned int | GetNumLaunches () |
Get the number of times the sensor has been updated. More... | |
void | IncrementNumLaunches () |
Increments the count of number of updates. | |
std::list< std::shared_ptr< ChFilter > > | GetFilterList () const |
Get the sensor's list of filters. More... | |
void | PushFilter (std::shared_ptr< ChFilter > filter) |
Add a filter to the sensor. More... | |
void | PushFilterFront (std::shared_ptr< ChFilter > filter) |
Add a filter to the front of the list on a sensor. More... | |
void | LockFilterList () |
Gives ability to lock the filter list to prevent race conditions. More... | |
template<class UserBufferType > | |
UserBufferType | GetMostRecentBuffer () |
Get the last filter in the list that matches the template type. More... | |
template<> | |
CH_SENSOR_API UserR8BufferPtr | GetMostRecentBuffer () |
template<> | |
CH_SENSOR_API UserRGBA8BufferPtr | GetMostRecentBuffer () |
template<> | |
CH_SENSOR_API UserDepthBufferPtr | GetMostRecentBuffer () |
template<> | |
CH_SENSOR_API UserDIBufferPtr | GetMostRecentBuffer () |
template<> | |
CH_SENSOR_API UserXYZIBufferPtr | GetMostRecentBuffer () |
template<> | |
CH_SENSOR_API UserRadarBufferPtr | GetMostRecentBuffer () |
template<> | |
CH_SENSOR_API UserRadarXYZBufferPtr | GetMostRecentBuffer () |
template<> | |
CH_SENSOR_API UserAccelBufferPtr | GetMostRecentBuffer () |
template<> | |
CH_SENSOR_API UserGyroBufferPtr | GetMostRecentBuffer () |
template<> | |
CH_SENSOR_API UserMagnetBufferPtr | GetMostRecentBuffer () |
template<> | |
CH_SENSOR_API UserGPSBufferPtr | GetMostRecentBuffer () |
template<> | |
CH_SENSOR_API UserTachometerBufferPtr | GetMostRecentBuffer () |
Static Public Member Functions | |
static LensParams | CalcInvRadialModel (ChVector3f params) |
calculate the parameters for the inverse polynomial model | |
Additional Inherited Members | |
![]() | |
PipelineType | m_pipeline_type |
the type of pipeline for rendering | |
![]() | |
float | m_updateRate |
sensor update rate | |
float | m_lag |
sensor lag from the time all scene information is available (sensor processing time) | |
float | m_collection_window |
time over which data is collected. More... | |
float | m_timeLastUpdated |
time since previous update | |
std::shared_ptr< chrono::ChBody > | m_parent |
object to which the sensor is attached | |
chrono::ChFrame< double > | m_offsetPose |
position and orientation of the sensor relative to its parent | |
std::string | m_name |
name of the sensor | |
unsigned int | m_num_launches |
number of times the sensor has been updated | |
std::list< std::shared_ptr< ChFilter > > | m_filters |
filter list for post-processing sensor data | |
bool | m_filter_list_locked = false |
gives ability to lock the filter list to prevent race conditions | |
Constructor & Destructor Documentation
◆ ChCameraSensor()
CH_SENSOR_API chrono::sensor::ChCameraSensor::ChCameraSensor | ( | std::shared_ptr< chrono::ChBody > | parent, |
float | updateRate, | ||
chrono::ChFrame< double > | offsetPose, | ||
unsigned int | w, | ||
unsigned int | h, | ||
float | hFOV, | ||
unsigned int | supersample_factor = 1 , |
||
CameraLensModelType | lens_model = CameraLensModelType::PINHOLE , |
||
bool | use_gi = false , |
||
float | gamma = 2.2 , |
||
bool | use_fog = true |
||
) |
Constructor for the base camera class that defaults to a pinhole lens model.
- Parameters
-
parent A shared pointer to a body on which the sensor should be attached. updateRate The desired update rate of the sensor in Hz. offsetPose The desired relative position and orientation of the sensor on the body. w The width of the image the camera should generate. h The height of the image the camera should generate. hFOV The horizontal field of view of the camera lens. supersample_factor The number of rays that should be sampled per pixel for antialiasing. lens_model A enum specifying the desired lens model. use_gi Enable the global illumination, with significant decrease in performace gamma correction of the image, 1 for linear color space, 2.2 for sRGB use_fog whether to use fog on this camera
Member Function Documentation
◆ GetCameraDistortionCoefficients()
|
inline |
returns the camera distortion coefficients k1, k2, k3
- Returns
- ChVector3f of the camera distortion coefficients k1, k2, k3
◆ GetCameraIntrinsicMatrix()
ChMatrix33< float > chrono::sensor::ChCameraSensor::GetCameraIntrinsicMatrix | ( | ) |
returns the 3x3 Intrinsic Matrix(K) of the camera
- Returns
- 3x3 ChMatrix33<flaot> Intrinsic Matrix(K) of the camera
◆ GetGamma()
|
inline |
returns the gamma correction value of this camera.
1 means no correction and the image is in linear color space. Useful for other ML applications 2.2 means the image is in sRGB color space. Useful for display
- Returns
- Gamma value of the image
◆ GetHFOV()
|
inline |
returns the camera's horizontal field of view.
Vertical field of view is determined by the image aspect ratio and the lens model
- Returns
- The horizontal field of view of the camera lens
◆ GetLensModelType()
|
inline |
returns the lens model type used for rendering
- Returns
- An enum specifying which lens model is being used. (0: PINHOLE, 1: FOV, 2: Radial)
◆ GetLensParameters()
|
inline |
returns the lens model parameters
- Returns
- LensParams struct of lens parameters. Will default to zeros for any terms not used. These are coverted for the inverse model
◆ GetSampleFactor()
|
inline |
Gets the number of samples per pixels in each direction used for super sampling.
- Returns
- the number of samples per pixel
◆ GetUseFog()
|
inline |
returns if the cemera should use fog as dictated by the scene
- Returns
- True if it does request
◆ GetUseGI()
|
inline |
returns if the cemera requesting global illumination
- Returns
- True if it does request
◆ SetRadialLensParameters()
void chrono::sensor::ChCameraSensor::SetRadialLensParameters | ( | ChVector3f | params | ) |
@briefSets the parameters for a radial lens distortion model.
Parameters should be given for the forward model. The backward distortion model will the used and calculated from the forward parameters given.
- Parameters
-
params the set of 3 radial parameters (k1,k2,k3)
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_sensor/sensors/ChCameraSensor.h
- /builds/uwsbel/chrono/src/chrono_sensor/sensors/ChCameraSensor.cpp