chrono::vehicle::ChFEATire Class Referenceabstract
Description
Co-rotational FEA tire template.
This tire is modeled as a mesh composed of co-rotational elements.
#include <ChFEATire.h>
Inheritance diagram for chrono::vehicle::ChFEATire:
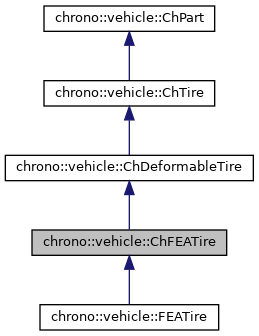
Collaboration diagram for chrono::vehicle::ChFEATire:
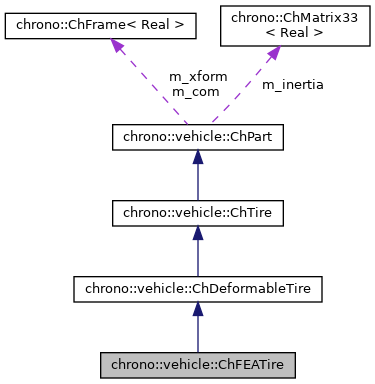
Public Member Functions | |
ChFEATire (const std::string &name) | |
virtual std::string | GetTemplateName () const override |
Get the name of the vehicle subsystem template. | |
![]() | |
ChDeformableTire (const std::string &name) | |
Construct a deformable tire with the specified name. | |
virtual double | GetTireMass () const override final |
Return the tire mass. | |
virtual ChVector3d | GetTireInertia () const override final |
Return the tire moments of inertia (in the tire centroidal frame). | |
std::shared_ptr< ChContactMaterialSMC > | GetContactMaterial () const |
Get the tire contact material. More... | |
void | EnablePressure (bool val) |
Enable/disable tire pressure (default: true). | |
bool | IsPressureEnabled () const |
void | EnableContact (bool val) |
Enable/disable tire contact (default: true). | |
bool | IsContactEnabled () const |
void | EnableRimConnection (bool val) |
Enable/disable tire-rim connection (default: true). | |
bool | IsRimConnectionEnabled () const |
void | AddVisualShapeFEA (std::shared_ptr< ChVisualShapeFEA > shape) |
Attach an FEA visual shape for run-time visualization. | |
std::shared_ptr< fea::ChMesh > | GetMesh () const |
Get the underlying FEA mesh. | |
std::shared_ptr< fea::ChContactSurface > | GetContactSurface () const |
Get the mesh contact surface. More... | |
std::shared_ptr< ChLoadContainer > | GetLoadContainer () const |
Get the load container associated with this tire. | |
virtual double | GetRimRadius () const =0 |
Get the rim radius (inner tire radius). / TODO: consider removing. | |
virtual TerrainForce | ReportTireForce (ChTerrain *terrain) const override |
Report the tire force and moment. More... | |
virtual TerrainForce | ReportTireForceLocal (ChTerrain *terrain, ChCoordsys<> &tire_frame) const override |
Get the tire force and moment expressed in the tire frame. More... | |
virtual void | AddVisualizationAssets (VisualizationType vis) override |
Add visualization assets for the rigid tire subsystem. | |
virtual void | RemoveVisualizationAssets () override |
Remove visualization assets for the rigid tire subsystem. | |
![]() | |
void | SetStepsize (double val) |
Set the integration step size for the underlying dynamics (default: 1ms). More... | |
double | GetStepsize () const |
Get the current value of the integration step size. | |
void | SetCollisionType (CollisionType collision_type) |
Set the collision type for tire-terrain interaction (default: SINGLE_POINT). More... | |
void | SetContactSurfaceType (ContactSurfaceType type, double dim=0.01, int collision_family=13) |
Set the contact surface type (default: NODE_CLOUD). More... | |
void | SetPressure (double pressure) |
Set the internal tire pressure [Pa] (default: 0). More... | |
double | GetPressure () const |
Get the internal tire pressure [Pa]. | |
virtual double | GetRadius () const =0 |
Get the tire radius. | |
virtual double | GetWidth () const =0 |
Get the tire width. | |
double | GetSlipAngle () const |
Return the tire slip angle calculated based on the current state of the associated wheel body. More... | |
double | GetLongitudinalSlip () const |
Return the tire longitudinal slip calculated based on the current state of the associated wheel body. More... | |
double | GetCamberAngle () const |
Return the tire camber angle calculated based on the current state of the associated wheel body. More... | |
virtual double | GetDeflection () const |
Report the tire deflection. | |
const std::string & | GetMeshFilename () const |
Get the name of the Wavefront file with tire visualization mesh. More... | |
std::shared_ptr< ChWheel > | GetWheel () const |
Get the associated wheel. More... | |
virtual void | Synchronize (double time, const ChTerrain &terrain) |
Update the state of this tire system at the current time. More... | |
virtual void | Advance (double step) |
Advance the state of this tire by the specified time step. | |
![]() | |
const std::string & | GetName () const |
Get the name identifier for this subsystem. | |
void | SetName (const std::string &name) |
Set the name identifier for this subsystem. | |
bool | IsInitialized () const |
Return flag indicating whether or not the part is fully constructed. | |
virtual uint16_t | GetVehicleTag () const |
Get the tag of the associated vehicle. More... | |
int | GetBodyTag () const |
Get the tag for component bodies. | |
double | GetMass () const |
Get the subsystem mass. More... | |
const ChFrame & | GetCOMFrame () const |
Get the current subsystem COM frame (relative to and expressed in the subsystem's reference frame). More... | |
const ChMatrix33 & | GetInertia () const |
Get the current subsystem inertia (relative to the subsystem COM frame). More... | |
const ChFrame & | GetTransform () const |
Get the current subsystem position relative to the global frame. More... | |
void | SetVisualizationType (VisualizationType vis) |
Set the visualization mode for this subsystem. | |
virtual void | SetOutput (bool state) |
Enable/disable output for this subsystem. | |
bool | OutputEnabled () const |
Return the output state for this subsystem. | |
virtual void | ExportComponentList (rapidjson::Document &jsonDocument) const |
Export this subsystem's component list to the specified JSON object. More... | |
virtual void | Output (ChVehicleOutput &database) const |
Output data for this subsystem's component list to the specified database. | |
Protected Member Functions | |
virtual std::vector< std::shared_ptr< fea::ChNodeFEAbase > > | GetInternalNodes () const =0 |
Return list of internal nodes. More... | |
virtual void | CreatePressureLoad () override final |
Create the ChLoad for applying pressure to the tire. | |
virtual void | CreateContactSurface () override final |
Create the contact surface for the tire mesh. | |
virtual void | CreateRimConnections (std::shared_ptr< ChBody > wheel) override final |
Create the tire-rim connections. | |
![]() | |
virtual double | GetDefaultPressure () const =0 |
Return the default tire pressure. | |
virtual std::vector< std::shared_ptr< fea::ChNodeFEAbase > > | GetConnectedNodes () const =0 |
Return list of nodes connected to the rim. / TODO Consider moving down to derived classes (CHANCFTire, CHReissnerTire, etc) | |
virtual void | CreateMesh (const ChFrameMoving<> &wheel_frame, VehicleSide side)=0 |
Create the FEA nodes and elements. More... | |
virtual void | CreateContactMaterial ()=0 |
Create the SMC contact material. | |
virtual double | GetAddedMass () const override final |
Get the mass added to the associated spindle body. More... | |
virtual ChVector3d | GetAddedInertia () const override final |
Get the inertia added to the associated spindle body. More... | |
virtual void | InitializeInertiaProperties () override |
Return the tire mass. | |
virtual void | UpdateInertiaProperties () override |
Return the tire moments of inertia (in the tire centroidal frame). | |
virtual void | Initialize (std::shared_ptr< ChWheel > wheel) override |
Initialize this tire by associating it to the specified wheel. | |
virtual TerrainForce | GetTireForce () const override final |
Get the tire force and moment. More... | |
![]() | |
ChTire (const std::string &name) | |
Construct a tire subsystem with given name. | |
void | CalculateKinematics (const WheelState &wheel_state, const ChCoordsys<> &tire_frame) |
Calculate kinematics quantities based on the given state of the associated wheel body. More... | |
double | GetOffset () const |
Get offset from spindle center. More... | |
std::shared_ptr< ChVisualShapeTriangleMesh > | AddVisualizationMesh (const std::string &mesh_file_left, const std::string &mesh_file_right) |
Add mesh visualization to the body associated with this tire (a wheel spindle body). More... | |
![]() | |
ChPart (const std::string &name) | |
Construct a vehicle subsystem with the specified name. | |
void | AddMass (double &mass) |
Add this subsystem's mass. More... | |
void | AddInertiaProperties (ChVector3d &com, ChMatrix33<> &inertia) |
Add this subsystem's inertia properties. More... | |
virtual void | Create (const rapidjson::Document &d) |
Create a vehicle subsystem from JSON data. More... | |
void | ExportBodyList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChBody >> bodies) const |
Export the list of bodies to the specified JSON document. | |
void | ExportShaftList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaft >> shafts) const |
Export the list of shafts to the specified JSON document. | |
void | ExportJointList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLink >> joints) const |
Export the list of joints to the specified JSON document. | |
void | ExportCouplesList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaftsCouple >> couples) const |
Export the list of shaft couples to the specified JSON document. | |
void | ExportMarkerList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChMarker >> markers) const |
Export the list of markers to the specified JSON document. | |
void | ExportLinSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkTSDA >> springs) const |
Export the list of translational springs to the specified JSON document. | |
void | ExportRotSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkRSDA >> springs) const |
Export the list of rotational springs to the specified JSON document. | |
void | ExportBodyLoadList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLoadBodyBody >> loads) const |
Export the list of body-body loads to the specified JSON document. | |
Additional Inherited Members | |
![]() | |
enum | CollisionType { SINGLE_POINT, FOUR_POINTS, ENVELOPE } |
Collision detection type. More... | |
enum | ContactSurfaceType { NODE_CLOUD, TRIANGLE_MESH } |
Contact surface type. More... | |
![]() | |
static ChVector3d | EstimateInertia (double tire_width, double aspect_ratio, double rim_diameter, double tire_mass, double t_factor=2) |
Utility function for estimating the tire moments of inertia. More... | |
![]() | |
static ChMatrix33 | TransformInertiaMatrix (const ChVector3d &moments, const ChVector3d &products, const ChMatrix33<> &vehicle_rot, const ChMatrix33<> &body_rot) |
Utility function for transforming inertia tensors between centroidal frames. More... | |
![]() | |
static bool | DiscTerrainCollision (CollisionType method, const ChTerrain &terrain, const ChVector3d &disc_center, const ChVector3d &disc_normal, double disc_radius, double width, const ChFunctionInterp &areaDep, ChCoordsys<> &contact, double &depth, float &mu) |
Perform disc-terrain collision detection, using the specified method. More... | |
static void | ConstructAreaDepthTable (double disc_radius, ChFunctionInterp &areaDep) |
Utility function to construct a loopkup table for penetration depth as function of intersection area, for a given tire radius. More... | |
static bool | DiscTerrainCollision1pt (const ChTerrain &terrain, const ChVector3d &disc_center, const ChVector3d &disc_normal, double disc_radius, ChCoordsys<> &contact, double &depth, float &mu) |
Perform disc-terrain collision detection. More... | |
static bool | DiscTerrainCollision4pt (const ChTerrain &terrain, const ChVector3d &disc_center, const ChVector3d &disc_normal, double disc_radius, double width, ChCoordsys<> &contact, double &depth, float &mu) |
Perform disc-terrain collision detection considering the curvature of the road surface. More... | |
static bool | DiscTerrainCollisionEnvelope (const ChTerrain &terrain, const ChVector3d &disc_center, const ChVector3d &disc_normal, double disc_radius, double width, const ChFunctionInterp &areaDep, ChCoordsys<> &contact, double &depth, float &mu) |
Collsion algorithm based on a paper of J. More... | |
![]() | |
static void | RemoveVisualizationAssets (std::shared_ptr< ChPhysicsItem > item) |
Erase all visual shapes from the visual model associated with the specified physics item (if any). | |
static void | RemoveVisualizationAsset (std::shared_ptr< ChPhysicsItem > item, std::shared_ptr< ChVisualShape > shape) |
Erase the given shape from the visual model associated with the specified physics item (if any). | |
![]() | |
std::shared_ptr< fea::ChMesh > | m_mesh |
tire mesh | |
std::shared_ptr< ChLoadContainer > | m_load_container |
load container (for pressure load) | |
std::vector< std::shared_ptr< fea::ChLinkNodeFrame > > | m_connections |
tire-wheel point connections | |
std::vector< std::shared_ptr< fea::ChLinkNodeSlopeFrame > > | m_connectionsD |
tire-wheel direction connections | |
std::vector< std::shared_ptr< ChLinkMateFix > > | m_connectionsF |
tire-wheel fix connection (point+rotation) | |
bool | m_connection_enabled |
enable tire connections to rim | |
bool | m_pressure_enabled |
enable internal tire pressure | |
bool | m_contact_enabled |
enable tire-terrain contact | |
std::shared_ptr< ChContactMaterialSMC > | m_contact_mat |
tire contact material | |
std::vector< std::shared_ptr< ChVisualShapeFEA > > | m_visFEA |
tire mesh FEA visual shapes | |
![]() | |
std::shared_ptr< ChWheel > | m_wheel |
associated wheel subsystem | |
double | m_stepsize |
tire integration step size (if applicable) | |
double | m_pressure |
internal tire pressure | |
CollisionType | m_collision_type |
method used for tire-terrain collision | |
ContactSurfaceType | m_contact_surface_type |
type of contact surface model (node cloud or mesh) | |
double | m_contact_surface_dim |
contact surface dimension (node radius or mesh thickness) | |
int | m_collision_family |
collision family for this tire | |
std::string | m_vis_mesh_file |
name of OBJ file for visualization of this tire (may be empty) | |
double | m_slip_angle |
double | m_longitudinal_slip |
double | m_camber_angle |
![]() | |
std::string | m_name |
subsystem name | |
bool | m_initialized |
specifies whether ot not the part is fully constructed | |
bool | m_output |
specifies whether or not output is generated for this subsystem | |
std::shared_ptr< ChPart > | m_parent |
parent subsystem (empty if parent is vehicle) | |
double | m_mass |
subsystem mass | |
ChMatrix33 | m_inertia |
inertia tensor (relative to subsystem COM) | |
ChFrame | m_com |
COM frame (relative to subsystem reference frame) | |
ChFrame | m_xform |
subsystem frame expressed in the global frame | |
int | m_obj_tag |
tag for part objects | |
Member Function Documentation
◆ GetInternalNodes()
|
protectedpure virtual |
Return list of internal nodes.
These nodes define the mesh surface over which pressure loads are applied.
Implemented in chrono::vehicle::FEATire.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_vehicle/wheeled_vehicle/tire/ChFEATire.h
- /builds/uwsbel/chrono/src/chrono_vehicle/wheeled_vehicle/tire/ChFEATire.cpp